mirror of
https://github.com/microsoft/PowerToys
synced 2024-11-22 00:03:48 +00:00
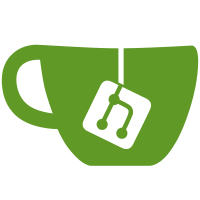
* Flatten everything and succeed build * Figure out Settings assets * Remove UseCommonOutputDirectory tag * Proper settings app path * [VCM] Fix assets location * Fix some runtime paths * [RegistryPreview]Use MRTCore specific pri file * [Hosts]Use MRTCore specific pri file * [Settings]Use MRTCore specific pri file * [Peek]Use MRTCore specific pri file * [FileLocksmith]Use MRTCore specific pri file * [ScreenRuler]Use MRTCore specific pri file * [PowerRename]Use MRTCore specific pri file * [Peek]Move assets to own folder * [FileLocksmith] Use own Assets path * [Hosts]Use own assets folder * [PowerRename]Use own assets dir * [MeasureTool] Use its own assets folder * [ImageResizer]Use its own assets path * Fix spellcheck * Fix tab instead of space in project files * Normalize target frameworks and platforms * Remove WINRT_NO_MAKE_DETECTION flag. No longer needed? * Fix AOT and Hosts locations * Fix Dll version differences on dependency * Add Common.UI.csproj as refernce to fix dll versions * Update ControlzEx to normalize dll versions * Update System.Management version to 7.0.2 * Add GPOWrapper to Registry Preview to fix dll versions * [PTRun]Reference Microsoft.Extensions.Hosting to fix dll versions * Fix remaining output paths / dll version conflicts * [KeyboardManager]Executables still on their own directories * Fix Monaco paths * WinAppSDK apps get to play outside again * Enable VCM settings again * Fix KBM Editor path again * [Monaco]Set to own Assets dir * Fix installer preamble; Fix publish. remove unneeded publishes * Remove Hardlink functions * Installer builds again (still needs work to work) * Readd Monaco to spellcheck excludes * Fix spellcheck and call publish.cmd again * [Installer] Remove components that don't need own dirs * [Installer] Refactor Power Launcher * [Installer] Refactor Color Picker * [Installer] Refactor Monaco assets * [Installer]Generate File script no longer needs to remove files * [Installer]Refactor FileLocksmith * [Installer] Refactor Hosts * [Installer]Refactor Image Resizer * [Installer]Refactor MouseUtils * [Installer]Refactor MWB * [Installer]Refactor MeasureTool * [Installer]Refactor Peek * [Installer]Refactor PowerRename and registry fixes * [Installer]Refactor RegistryPreview * [Installer]Refactor ShortcutGuide * [Installer]Refactor Settings * [Installer]Clean up some unused stuff * [Installer]Clean up stuff for user install * [Installer]Fix WinUi3Apps wxs * [Installer]Fix misplaced folders * [Installer]Move x86 VCM dll to right path * Fix monaco language list location * [Installer]Fix VCM directory reference * [CI]Fix signing * [Installer] Fix resources folder for release CI * [ci]Looks like we still ship NLog on PowerToys Run * [Settings]Add dependency to avoid dll collision with Experimentation * [RegistryPreview]Move XAML files to own path * [RegistryPreview]Fix app icon * [Hosts]Move XAML files to their own path * [FileLocksmith]Move XAML files to their own path * [Peek]Move XAML files to own path * [ScreenRuler]Move XAML files to its own path * [Settings]Move XAML to its own path * [ColorPicker]Move Resources to Assets * [ShortcutGuide]Move svgs to own Assets path * [Awake]Move images to assets path * [Runner]Remove debug checks for PowerToys Run assets * [PTRun]Move images to its own assets path * [ImageResizer]Icon for context menu on own assets path * [PowerRename]Move ico to its own path * Remove unneeded intermediary directories * Remove further int dirs * Move tests to its own output path * Fix spellcheck * spellcheck: remove warnings * [CppAnalyzers]Ignore rule in a tool * [CI]Check if all deps.json files reference same versions * fix spellcheck * [ci]Fix task identation * [ci]Add script to guard against asset conflicts * [ci]Add more deps.json audit steps in the release build * Add xbf to spellcheck expects * Fix typo in asset conflict check scripts * Fix some more dependency conflicts * Downgrade CsWinRT to have the same dll version as sdk * [ci]Do a recursive check for every deps.json * Fix spellcheck error inside comment * [ci]Fix asset script error * [ci]Name deps.json verify tasks a bit better * [ci]Improve deps json verify script output * [ci]Update WinRT version to the same running in CI * Also upgrade CsWinRT in NOTICE.MD * [PowerRename]Move XAML files to own path * [Common]Fix Settings executable path * [ci]Verify there's no xbf files in app directories * [installer]Fix firewall path * [Monaco]Move new files to their proper assets path * [Monaco]Fix paths for new files after merge * [Feedback]Removed unneeded build conditions * [Feedback]Clear vcxproj direct reference to frameworks * [Feedback]RunPlugins name to hold PTRun plugins * [Feedback]Remove unneeded foreach * [Feedback]Shortcut Guide svgs with ** in project file * [Feedback]Fix spellcheck
241 lines
6.7 KiB
C++
241 lines
6.7 KiB
C++
#include <initguid.h>
|
|
#define WIN32_LEAN_AND_MEAN
|
|
|
|
#include <windows.h>
|
|
#include <dshow.h>
|
|
#include <cguid.h>
|
|
|
|
// disable warning 26471 - Don't use reinterpret_cast. A cast from void* can use static_cast
|
|
// disable warning 26492 - Don't use const_cast to cast away const
|
|
// disable warning 26493 - Don't use C-style casts
|
|
// Disable 26497 for winrt - This function function-name could be marked constexpr if compile-time evaluation is desired.
|
|
// disable 26403 - Reset or explicitly delete an owner<T> pointer
|
|
#pragma warning(push)
|
|
#pragma warning(disable : 26471 26492 26493 26497 26403)
|
|
#include <wil/com.h>
|
|
#pragma warning(pop)
|
|
|
|
#include <wil/resource.h>
|
|
|
|
#include <iostream>
|
|
#include <fstream>
|
|
#include <sstream>
|
|
#include <string>
|
|
#include <Shlobj.h>
|
|
#include <Shlobj_core.h>
|
|
|
|
#include "DirectShowUtils.h"
|
|
|
|
std::ofstream& log()
|
|
{
|
|
static std::ofstream report = []{
|
|
char buffer[MAX_PATH]{};
|
|
if (SHGetSpecialFolderPathA(HWND_DESKTOP, buffer, CSIDL_DESKTOP, false))
|
|
{
|
|
std::string path = buffer;
|
|
path += "\\WebcamReport.txt";
|
|
return std::ofstream{ path };
|
|
}
|
|
else
|
|
{
|
|
return std::ofstream{ "WebcamReport.txt" };
|
|
}
|
|
}();
|
|
return report;
|
|
}
|
|
|
|
std::string GetMediaSubTypeString(const GUID& guid)
|
|
{
|
|
if (guid == MEDIASUBTYPE_RGB24)
|
|
{
|
|
return "MEDIASUBTYPE_RGB24";
|
|
}
|
|
else if (guid == MEDIASUBTYPE_RGB32)
|
|
{
|
|
return "MEDIASUBTYPE_RGB32";
|
|
}
|
|
else if (guid == MEDIASUBTYPE_YUY2)
|
|
{
|
|
return "MEDIASUBTYPE_YUY2";
|
|
}
|
|
else if (guid == MEDIASUBTYPE_MJPG)
|
|
{
|
|
return "MEDIASUBTYPE_MJPG";
|
|
}
|
|
else if (guid == MEDIASUBTYPE_NV12)
|
|
{
|
|
return "MEDIASUBTYPE_NV12";
|
|
}
|
|
else if (guid == MEDIASUBTYPE_NV11)
|
|
{
|
|
return "MEDIASUBTYPE_NV11";
|
|
}
|
|
else if (guid == MEDIASUBTYPE_YV12)
|
|
{
|
|
return "MEDIASUBTYPE_YV12";
|
|
}
|
|
else if (guid == MEDIASUBTYPE_YUYV)
|
|
{
|
|
return "MEDIASUBTYPE_YUYV";
|
|
}
|
|
else
|
|
{
|
|
OLECHAR* guidString = nullptr;
|
|
StringFromCLSID(guid, &guidString);
|
|
if (guidString)
|
|
{
|
|
std::wstring_view wideView{guidString};
|
|
std::string result;
|
|
for (const auto c :wideView)
|
|
{
|
|
result += static_cast<char>(c);
|
|
}
|
|
::CoTaskMemFree(guidString);
|
|
return result;
|
|
}
|
|
else
|
|
{
|
|
return "MEDIASUBTYPE_UNKNOWN";
|
|
}
|
|
}
|
|
}
|
|
|
|
std::string asString(RECT r)
|
|
{
|
|
std::ostringstream s;
|
|
if (r.left == r.right && r.bottom == r.right && r.top == r.right && r.right == 0)
|
|
return "[ZEROES]";
|
|
s << '(' << r.left << ", " << r.top << ") - (" << r.right << ", " << r.bottom << ")";
|
|
return s.str();
|
|
}
|
|
|
|
void LogMediaTypes(wil::com_ptr_nothrow<IPin>& pin)
|
|
{
|
|
wil::com_ptr_nothrow<IEnumMediaTypes> mediaTypeEnum;
|
|
if (pin->EnumMediaTypes(&mediaTypeEnum); !mediaTypeEnum)
|
|
{
|
|
return;
|
|
}
|
|
ULONG _ = 0;
|
|
unique_media_type_ptr mt;
|
|
log() << "Supported formats:\n";
|
|
while (mediaTypeEnum->Next(1, wil::out_param(mt), &_) == S_OK)
|
|
{
|
|
if (mt->majortype != MEDIATYPE_Video)
|
|
{
|
|
continue;
|
|
}
|
|
auto format = reinterpret_cast<VIDEOINFOHEADER*>(mt->pbFormat);
|
|
if (!format->AvgTimePerFrame)
|
|
{
|
|
continue;
|
|
}
|
|
const auto formatAvgFPS = 10000000LL / format->AvgTimePerFrame;
|
|
log() << GetMediaSubTypeString(mt->subtype) << '\t' << format->bmiHeader.biWidth << "x"
|
|
<< format->bmiHeader.biHeight << " - " << formatAvgFPS << "fps src rect: " << asString(format->rcSource)
|
|
<< " dst rect: " << asString(format->rcSource)
|
|
<< " flipped: " << std::boolalpha << (format->bmiHeader.biHeight < 0) << '\n';
|
|
}
|
|
log() << '\n';
|
|
}
|
|
|
|
void ReportAllWebcams()
|
|
{
|
|
auto enumeratorFactory = wil::CoCreateInstanceNoThrow<ICreateDevEnum>(CLSID_SystemDeviceEnum);
|
|
if (!enumeratorFactory)
|
|
{
|
|
LOG("Couldn't create devenum factory");
|
|
return;
|
|
}
|
|
|
|
wil::com_ptr_nothrow<IEnumMoniker> enumMoniker;
|
|
enumeratorFactory->CreateClassEnumerator(CLSID_VideoInputDeviceCategory, &enumMoniker, CDEF_DEVMON_PNP_DEVICE);
|
|
if (!enumMoniker)
|
|
{
|
|
LOG("Couldn't create class enumerator");
|
|
return;
|
|
}
|
|
|
|
ULONG _ = 0;
|
|
wil::com_ptr_nothrow<IMoniker> moniker;
|
|
while (enumMoniker->Next(1, &moniker, &_) == S_OK)
|
|
{
|
|
wil::com_ptr_nothrow<IPropertyBag> propertyData;
|
|
moniker->BindToStorage(nullptr, nullptr, IID_IPropertyBag, reinterpret_cast<void**>(&propertyData));
|
|
if (!propertyData)
|
|
{
|
|
LOG("BindToStorage failed");
|
|
continue;
|
|
}
|
|
|
|
wil::unique_variant propVal;
|
|
propVal.vt = VT_BSTR;
|
|
|
|
if (FAILED(propertyData->Read(L"FriendlyName", &propVal, nullptr)))
|
|
{
|
|
LOG("Couldn't obtain FriendlyName property");
|
|
continue;
|
|
}
|
|
std::wstring wideFriendlyName = { propVal.bstrVal, SysStringLen(propVal.bstrVal) };
|
|
std::string friendlyName;
|
|
for (wchar_t c : wideFriendlyName)
|
|
{
|
|
friendlyName += static_cast<char>(c);
|
|
}
|
|
log() << "Webcam " << friendlyName << '\n';
|
|
|
|
propVal.reset();
|
|
propVal.vt = VT_BSTR;
|
|
|
|
if (FAILED(propertyData->Read(L"DevicePath", &propVal, nullptr)))
|
|
{
|
|
LOG("Couldn't obtain DevicePath property");
|
|
continue;
|
|
}
|
|
wil::com_ptr_nothrow<IBaseFilter> filter;
|
|
moniker->BindToObject(nullptr, nullptr, IID_IBaseFilter, reinterpret_cast<void**>(&filter));
|
|
if (!filter)
|
|
{
|
|
LOG("Couldn't BindToObject");
|
|
continue;
|
|
}
|
|
|
|
wil::com_ptr_nothrow<IEnumPins> pinsEnum;
|
|
if (FAILED(filter->EnumPins(&pinsEnum)))
|
|
{
|
|
LOG("BindToObject EnumPins");
|
|
continue;
|
|
}
|
|
wil::com_ptr_nothrow<IPin> pin;
|
|
|
|
while (pinsEnum->Next(1, &pin, &_) == S_OK)
|
|
{
|
|
// Skip pins which do not belong to capture category
|
|
GUID category{};
|
|
DWORD __;
|
|
if (auto props = pin.try_copy<IKsPropertySet>();
|
|
!props ||
|
|
FAILED(props->Get(AMPROPSETID_Pin, AMPROPERTY_PIN_CATEGORY, nullptr, 0, &category, sizeof(GUID), &__)) ||
|
|
category != PIN_CATEGORY_CAPTURE)
|
|
{
|
|
continue;
|
|
}
|
|
|
|
// Skip non-output pins
|
|
if (PIN_DIRECTION direction = {}; FAILED(pin->QueryDirection(&direction)) || direction != PINDIR_OUTPUT)
|
|
{
|
|
continue;
|
|
}
|
|
LogMediaTypes(pin);
|
|
}
|
|
}
|
|
}
|
|
|
|
int main()
|
|
{
|
|
auto comCtx = wil::CoInitializeEx();
|
|
log() << "Report started\n";
|
|
ReportAllWebcams();
|
|
return 0;
|
|
}
|