mirror of
https://github.com/Z4nzu/hackingtool
synced 2024-11-14 19:55:19 +00:00
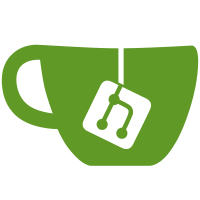
List of changes + Handling information about a tool has been improved a lot by providing a `HackingTool` class, which takes care of showing the options, running the selected option, executing the required commands + This class is designed with flexibililty and simplicity in mind, so adding a new tool is a lot easier, mention TITLE, DESCRIPTION, list of INSTALL_COMMANDS, RUN_COMMANDS and PROJECT_URL and there you go... + grouping all the `HackingTool`s is also made super simpler by providing a `HackingToolsCollection` class which groups the tools into their respective categories. Just add the instances of `HackingTool` classes to the TOOLS property of the `HackingToolsCollection`. + Refactored all the tools into separate files based on their categories. + Added a READM_template.md and generate_readme.py script to automatically generate Table of contents and the list of tools available automatically. + Now each tool in the README.md points to its project url if provided. This makes it easier to visit the project from the readme.
92 lines
3.0 KiB
Python
92 lines
3.0 KiB
Python
# coding=utf-8
|
||
import os
|
||
|
||
from core import HackingTool
|
||
from core import HackingToolsCollection
|
||
|
||
|
||
class Autopsy(HackingTool):
|
||
TITLE = "Autopsy"
|
||
DESCRIPTION = "Autopsy is a platform that is used by Cyber Investigators.\n" \
|
||
"[!] Works in any Os\n" \
|
||
"[!] Recover Deleted Files from any OS & MEdia \n" \
|
||
"[!] Extract Image Metadata"
|
||
RUN_COMMANDS = "sudo autopsy"
|
||
|
||
def __init__(self):
|
||
super(Autopsy, self).__init__(installable = False)
|
||
|
||
|
||
class Wireshark(HackingTool):
|
||
TITLE = "Wireshark"
|
||
DESCRIPTION = "Wireshark is a network capture and analyzer \n" \
|
||
"tool to see what’s happening in your network.\n " \
|
||
"And also investigate Network related incident"
|
||
RUN_COMMANDS = ["sudo wireshark"]
|
||
|
||
def __init__(self):
|
||
super(Wireshark, self).__init__(installable = False)
|
||
|
||
|
||
class BulkExtractor(HackingTool):
|
||
TITLE = "Bulk extractor"
|
||
DESCRIPTION = ""
|
||
PROJECT_URL = "https://github.com/simsong/bulk_extractor"
|
||
|
||
def __init__(self):
|
||
super(BulkExtractor, self).__init__([
|
||
('GUI Mode (Download required)', self.gui_mode),
|
||
('CLI Mode', self.cli_mode)
|
||
], installable = False, runnable = False)
|
||
|
||
def gui_mode(self):
|
||
os.system(
|
||
"sudo git clone https://github.com/simsong/bulk_extractor.git")
|
||
os.system("ls src/ && cd .. && cd java_gui && ./BEViewer")
|
||
print(
|
||
"If you getting error after clone go to /java_gui/src/ And Compile .Jar file && run ./BEViewer")
|
||
print(
|
||
"Please Visit For More Details About Installation >> https://github.com/simsong/bulk_extractor")
|
||
|
||
def cli_mode(self):
|
||
os.system("sudo apt-get install bulk_extractor")
|
||
print("bulk_extractor and options")
|
||
os.system("bulk_extractor")
|
||
os.system(
|
||
'echo "bulk_extractor [options] imagefile" | boxes -d headline | lolcat')
|
||
|
||
|
||
class Guymager(HackingTool):
|
||
TITLE = "Disk Clone and ISO Image Aquire"
|
||
DESCRIPTION = "Guymager is a free forensic imager for media acquisition."
|
||
INSTALL_COMMANDS = ["sudo apt install guymager"]
|
||
RUN_COMMANDS = ["sudo guymager"]
|
||
PROJECT_URL = "https://guymager.sourceforge.io/"
|
||
|
||
|
||
class Toolsley(HackingTool):
|
||
TITLE = "Toolsley"
|
||
DESCRIPTION = "Toolsley got more than ten useful tools for investigation.\n" \
|
||
"[+]File signature verifier\n" \
|
||
"[+]File identifier \n" \
|
||
"[+]Hash & Validate \n" \
|
||
"[+]Binary inspector \n " \
|
||
"[+]Encode text \n" \
|
||
"[+]Data URI generator \n" \
|
||
"[+]Password generator"
|
||
PROJECT_URL = "https://www.toolsley.com/"
|
||
|
||
def __init__(self):
|
||
super(Toolsley, self).__init__(installable = False, runnable = False)
|
||
|
||
|
||
class ForensicTools(HackingToolsCollection):
|
||
TITLE = "Forensic tools"
|
||
TOOLS = [
|
||
Autopsy(),
|
||
Wireshark(),
|
||
BulkExtractor(),
|
||
Guymager(),
|
||
Toolsley()
|
||
]
|