mirror of
https://github.com/Z4nzu/hackingtool
synced 2024-11-14 19:55:19 +00:00
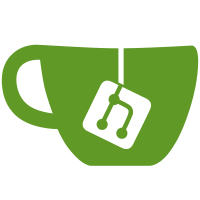
List of changes + Handling information about a tool has been improved a lot by providing a `HackingTool` class, which takes care of showing the options, running the selected option, executing the required commands + This class is designed with flexibililty and simplicity in mind, so adding a new tool is a lot easier, mention TITLE, DESCRIPTION, list of INSTALL_COMMANDS, RUN_COMMANDS and PROJECT_URL and there you go... + grouping all the `HackingTool`s is also made super simpler by providing a `HackingToolsCollection` class which groups the tools into their respective categories. Just add the instances of `HackingTool` classes to the TOOLS property of the `HackingToolsCollection`. + Refactored all the tools into separate files based on their categories. + Added a READM_template.md and generate_readme.py script to automatically generate Table of contents and the list of tools available automatically. + Now each tool in the README.md points to its project url if provided. This makes it easier to visit the project from the readme.
118 lines
4.5 KiB
Python
118 lines
4.5 KiB
Python
# coding=utf-8
|
|
from core import HackingTool
|
|
from core import HackingToolsCollection
|
|
|
|
|
|
class Sqlmap(HackingTool):
|
|
TITLE = "Sqlmap tool"
|
|
DESCRIPTION = "sqlmap is an open source penetration testing tool that " \
|
|
"automates the process of \n" \
|
|
"detecting and exploiting SQL injection flaws and taking " \
|
|
"over of database servers \n " \
|
|
"[!] python sqlmap.py -u [<http://example.com>] --batch --banner \n " \
|
|
"More Usage [!] https://github.com/sqlmapproject/sqlmap/wiki/Usage"
|
|
INSTALL_COMMANDS = [
|
|
"sudo git clone --depth 1 https://github.com/sqlmapproject/sqlmap.git sqlmap-dev"]
|
|
PROJECT_URL = "https://github.com/sqlmapproject/sqlmap"
|
|
|
|
def __init__(self):
|
|
super(Sqlmap, self).__init__(runnable = False)
|
|
|
|
|
|
class NoSqlMap(HackingTool):
|
|
TITLE = "NoSqlMap"
|
|
DESCRIPTION = "NoSQLMap is an open source Python tool designed to \n " \
|
|
"audit for as well as automate injection attacks and exploit.\n " \
|
|
"\033[91m " \
|
|
"[*] Please Install MongoDB \n "
|
|
INSTALL_COMMANDS = [
|
|
"git clone https://github.com/codingo/NoSQLMap.git",
|
|
"sudo chmod -R 755 NoSQLMap;cd NoSQLMap;python setup.py install"
|
|
]
|
|
RUN_COMMANDS = ["python NoSQLMap"]
|
|
PROJECT_URL = "https://github.com/codingo/NoSQLMap"
|
|
|
|
|
|
class SQLiScanner(HackingTool):
|
|
TITLE = "Damn Small SQLi Scanner"
|
|
DESCRIPTION = "Damn Small SQLi Scanner (DSSS) is a fully functional SQL " \
|
|
"injection\nvulnerability scanner also supporting GET and " \
|
|
"POST parameters.\n" \
|
|
"[*]python3 dsss.py -h[help] | -u[URL]"
|
|
INSTALL_COMMANDS = ["git clone https://github.com/stamparm/DSSS.git"]
|
|
PROJECT_URL = "https://github.com/stamparm/DSSS"
|
|
|
|
def __init__(self):
|
|
super(SQLiScanner, self).__init__(runnable = False)
|
|
|
|
|
|
class Explo(HackingTool):
|
|
TITLE = "Explo"
|
|
DESCRIPTION = "Explo is a simple tool to describe web security issues " \
|
|
"in a human and machine readable format.\n " \
|
|
"Usage:- \n " \
|
|
"[1] explo [--verbose|-v] testcase.yaml \n " \
|
|
"[2] explo [--verbose|-v] examples/*.yaml"
|
|
INSTALL_COMMANDS = [
|
|
"git clone https://github.com/dtag-dev-sec/explo.git",
|
|
"cd explo;sudo python setup.py install"
|
|
]
|
|
PROJECT_URL = "https://github.com/dtag-dev-sec/explo"
|
|
|
|
def __init__(self):
|
|
super(Explo, self).__init__(runnable = False)
|
|
|
|
|
|
class Blisqy(HackingTool):
|
|
TITLE = "Blisqy - Exploit Time-based blind-SQL injection"
|
|
DESCRIPTION = "Blisqy is a tool to aid Web Security researchers to find " \
|
|
"Time-based Blind SQL injection \n on HTTP Headers and also " \
|
|
"exploitation of the same vulnerability.\n " \
|
|
"For Usage >> \n"
|
|
INSTALL_COMMANDS = ["git clone https://github.com/JohnTroony/Blisqy.git"]
|
|
PROJECT_URL = "https://github.com/JohnTroony/Blisqy"
|
|
|
|
def __init__(self):
|
|
super(Blisqy, self).__init__(runnable = False)
|
|
|
|
|
|
class Leviathan(HackingTool):
|
|
TITLE = "Leviathan - Wide Range Mass Audit Toolkit"
|
|
DESCRIPTION = "Leviathan is a mass audit toolkit which has wide range " \
|
|
"service discovery,\nbrute force, SQL injection detection " \
|
|
"and running custom exploit capabilities. \n " \
|
|
"[*] It Requires API Keys \n " \
|
|
"More Usage [!] https://github.com/utkusen/leviathan/wiki"
|
|
INSTALL_COMMANDS = [
|
|
"git clone https://github.com/leviathan-framework/leviathan.git",
|
|
"cd leviathan;sudo pip install -r requirements.txt"
|
|
]
|
|
RUN_COMMANDS = ["cd leviathan;python leviathan.py"]
|
|
PROJECT_URL = "https://github.com/leviathan-framework/leviathan"
|
|
|
|
|
|
class SQLScan(HackingTool):
|
|
TITLE = "SQLScan"
|
|
DESCRIPTION = "sqlscan is quick web scanner for find an sql inject point." \
|
|
" not for educational, this is for hacking."
|
|
INSTALL_COMMANDS = [
|
|
"sudo apt install php php-bz2 php-curl php-mbstring curl",
|
|
"sudo curl https://raw.githubusercontent.com/Cvar1984/sqlscan/dev/build/main.phar --output /usr/local/bin/sqlscan",
|
|
"chmod +x /usr/local/bin/sqlscan"
|
|
]
|
|
RUN_COMMANDS = ["sudo sqlscan"]
|
|
PROJECT_URL = "https://github.com/Cvar1984/sqlscan"
|
|
|
|
|
|
class SqlInjectionTools(HackingToolsCollection):
|
|
TITLE = "SQL Injection Tools"
|
|
TOOLS = [
|
|
Sqlmap(),
|
|
NoSqlMap(),
|
|
SQLiScanner(),
|
|
Explo(),
|
|
Blisqy(),
|
|
Leviathan(),
|
|
SQLScan()
|
|
]
|