mirror of
https://github.com/Z4nzu/hackingtool
synced 2024-11-14 19:55:19 +00:00
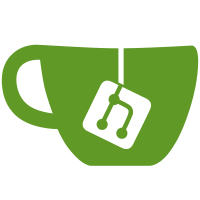
List of changes + Handling information about a tool has been improved a lot by providing a `HackingTool` class, which takes care of showing the options, running the selected option, executing the required commands + This class is designed with flexibililty and simplicity in mind, so adding a new tool is a lot easier, mention TITLE, DESCRIPTION, list of INSTALL_COMMANDS, RUN_COMMANDS and PROJECT_URL and there you go... + grouping all the `HackingTool`s is also made super simpler by providing a `HackingToolsCollection` class which groups the tools into their respective categories. Just add the instances of `HackingTool` classes to the TOOLS property of the `HackingToolsCollection`. + Refactored all the tools into separate files based on their categories. + Added a READM_template.md and generate_readme.py script to automatically generate Table of contents and the list of tools available automatically. + Now each tool in the README.md points to its project url if provided. This makes it easier to visit the project from the readme.
67 lines
2.1 KiB
Python
67 lines
2.1 KiB
Python
# coding=utf-8
|
|
import os
|
|
from time import sleep
|
|
|
|
from core import HackingTool
|
|
from core import HackingToolsCollection
|
|
|
|
|
|
class UpdateTool(HackingTool):
|
|
TITLE = "Update Tool or System"
|
|
DESCRIPTION = "Update Tool or System"
|
|
|
|
def __init__(self):
|
|
super(UpdateTool, self).__init__([
|
|
("Update System", self.update_sys),
|
|
("Update Hackingtool", self.update_ht)
|
|
], installable = False, runnable = False)
|
|
|
|
def update_sys(self):
|
|
os.system("sudo apt update && sudo apt full-upgrade -y")
|
|
os.system(
|
|
"sudo apt-get install tor openssl curl && sudo apt-get update tor openssl curl")
|
|
os.system("sudo apt-get install python3-pip")
|
|
|
|
def update_ht(self):
|
|
os.system("sudo chmod +x /etc/;"
|
|
"sudo chmod +x /usr/share/doc;"
|
|
"sudo rm -rf /usr/share/doc/hackingtool/;"
|
|
"cd /etc/;"
|
|
"sudo rm -rf /etc/hackingtool/;"
|
|
"mkdir hackingtool;"
|
|
"cd hackingtool;"
|
|
"git clone https://github.com/Z4nzu/hackingtool.git;"
|
|
"cd hackingtool;"
|
|
"sudo chmod +x install.sh;"
|
|
"./install.sh")
|
|
|
|
|
|
class UninstallTool(HackingTool):
|
|
TITLE = "Uninstall HackingTool"
|
|
DESCRIPTION = "Uninstall HackingTool"
|
|
|
|
def __init__(self):
|
|
super(UninstallTool, self).__init__([
|
|
('Uninstall', self.uninstall)
|
|
], installable = False, runnable = False)
|
|
|
|
def uninstall(self):
|
|
print("hackingtool started to uninstall..\n")
|
|
sleep(1)
|
|
os.system("sudo chmod +x /etc/;"
|
|
"sudo chmod +x /usr/share/doc;"
|
|
"sudo rm -rf /usr/share/doc/hackingtool/;"
|
|
"cd /etc/;"
|
|
"sudo rm -rf /etc/hackingtool/;")
|
|
print("\nHackingtool Successfully Uninstalled..")
|
|
print("Happy Hacking..!!")
|
|
sleep(1)
|
|
|
|
|
|
class ToolManager(HackingToolsCollection):
|
|
TITLE = "Update or Uninstall | Hackingtool"
|
|
TOOLS = [
|
|
UpdateTool(),
|
|
UninstallTool()
|
|
]
|