mirror of
https://github.com/Z4nzu/hackingtool
synced 2024-11-15 04:05:29 +00:00
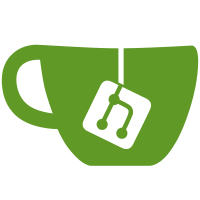
List of changes + Handling information about a tool has been improved a lot by providing a `HackingTool` class, which takes care of showing the options, running the selected option, executing the required commands + This class is designed with flexibililty and simplicity in mind, so adding a new tool is a lot easier, mention TITLE, DESCRIPTION, list of INSTALL_COMMANDS, RUN_COMMANDS and PROJECT_URL and there you go... + grouping all the `HackingTool`s is also made super simpler by providing a `HackingToolsCollection` class which groups the tools into their respective categories. Just add the instances of `HackingTool` classes to the TOOLS property of the `HackingToolsCollection`. + Refactored all the tools into separate files based on their categories. + Added a READM_template.md and generate_readme.py script to automatically generate Table of contents and the list of tools available automatically. + Now each tool in the README.md points to its project url if provided. This makes it easier to visit the project from the readme.
156 lines
6.2 KiB
Python
156 lines
6.2 KiB
Python
# coding=utf-8
|
|
import os
|
|
|
|
from core import HackingTool
|
|
from core import HackingToolsCollection
|
|
|
|
|
|
class WIFIPumpkin(HackingTool):
|
|
TITLE = "WiFi-Pumpkin"
|
|
DESCRIPTION = "The WiFi-Pumpkin is a rogue AP framework to easily create " \
|
|
"these fake networks\n" \
|
|
"all while forwarding legitimate traffic to and from the " \
|
|
"unsuspecting target."
|
|
INSTALL_COMMANDS = [
|
|
"sudo apt install libssl-dev libffi-dev build-essential",
|
|
"sudo git clone https://github.com/P0cL4bs/wifipumpkin3.git",
|
|
"chmod -R 755 wifipumpkin3 && cd wifipumpkin3",
|
|
"sudo apt install python3-pyqt5",
|
|
"sudo python3 setup.py install"
|
|
]
|
|
RUN_COMMANDS = ["sudo wifipumpkin3"]
|
|
PROJECT_URL = "https://github.com/P0cL4bs/wifipumpkin3"
|
|
|
|
|
|
class pixiewps(HackingTool):
|
|
TITLE = "pixiewps"
|
|
DESCRIPTION = "Pixiewps is a tool written in C used to bruteforce offline " \
|
|
"the WPS pin\n " \
|
|
"exploiting the low or non-existing entropy of some Access " \
|
|
"Points, the so-called pixie dust attack"
|
|
INSTALL_COMMANDS = [
|
|
"sudo git clone https://github.com/wiire/pixiewps.git && apt-get -y install build-essential",
|
|
"cd pixiewps*/ && make",
|
|
"cd pixiewps*/ && sudo make install && wget https://pastebin.com/y9Dk1Wjh"
|
|
]
|
|
PROJECT_URL = "https://github.com/wiire/pixiewps"
|
|
|
|
def run(self):
|
|
os.system(
|
|
'echo "'
|
|
'1.> Put your interface into monitor mode using '
|
|
'\'airmon-ng start {wireless interface}\n'
|
|
'2.> wash -i {monitor-interface like mon0}\'\n'
|
|
'3.> reaver -i {monitor interface} -b {BSSID of router} -c {router channel} -vvv -K 1 -f"'
|
|
'| boxes -d boy')
|
|
print("You Have To Run Manually By USing >>pixiewps -h ")
|
|
|
|
|
|
class BluePot(HackingTool):
|
|
TITLE = "Bluetooth Honeypot GUI Framework"
|
|
DESCRIPTION = "You need to have at least 1 bluetooh receiver " \
|
|
"(if you have many it will work with those, too).\n" \
|
|
"You must install/libbluetooth-dev on " \
|
|
"Ubuntu/bluez-libs-devel on Fedora/bluez-devel on openSUSE"
|
|
INSTALL_COMMANDS = [
|
|
"wget https://github.com/andrewmichaelsmith/bluepot/raw/master/bin/bluepot-0.1.tar.gz "
|
|
"&& tar xfz bluepot-0.1.tar.gz && sudo java -jar bluepot/BluePot-0.1.jar"
|
|
]
|
|
RUN_COMMANDS = ["cd bluepot-0.1 && sudo java -jar bluepot/BluePot-0.1.jar"]
|
|
PROJECT_URL = "https://github.com/andrewmichaelsmith/bluepot"
|
|
|
|
|
|
class Fluxion(HackingTool):
|
|
TITLE = "Fluxion"
|
|
DESCRIPTION = "Fluxion is a wifi key cracker using evil twin attack..\n" \
|
|
"you need a wireless adaptor for this tool"
|
|
INSTALL_COMMANDS = [
|
|
"git clone https://github.com/thehackingsage/Fluxion.git",
|
|
"cd Fluxion && cd install && sudo chmod +x install.sh && sudo bash install.sh",
|
|
"cd .. ; sudo chmod +x fluxion.sh"
|
|
]
|
|
RUN_COMMANDS = ["cd Fluxion;sudo bash fluxion.sh"]
|
|
PROJECT_URL = "https://github.com/thehackingsage/Fluxion"
|
|
|
|
|
|
class Wifiphisher(HackingTool):
|
|
TITLE = "Wifiphisher"
|
|
DESCRIPTION = """
|
|
Wifiphisher is a rogue Access Point framework for conducting red team engagements or Wi-Fi security testing.
|
|
Using Wifiphisher, penetration testers can easily achieve a man-in-the-middle position against wireless clients by performing
|
|
targeted Wi-Fi association attacks. Wifiphisher can be further used to mount victim-customized web phishing attacks against the
|
|
connected clients in order to capture credentials (e.g. from third party login pages or WPA/WPA2 Pre-Shared Keys) or infect the
|
|
victim stations with malware..\n
|
|
For More Details Visit >> https://github.com/wifiphisher/wifiphisher
|
|
"""
|
|
INSTALL_COMMANDS = [
|
|
"git clone https://github.com/wifiphisher/wifiphisher.git",
|
|
"cd wifiphisher;sudo python3 setup.py install"
|
|
]
|
|
RUN_COMMANDS = ["cd wifiphisher;sudo wifiphisher"]
|
|
PROJECT_URL = "https://github.com/wifiphisher/wifiphisher"
|
|
|
|
|
|
class Wifite(HackingTool):
|
|
TITLE = "Wifite"
|
|
INSTALL_COMMANDS = [
|
|
"sudo git clone https://github.com/derv82/wifite2.git",
|
|
"cd wifite2 && sudo python3 setup.py install"
|
|
";sudo pip3 install -r requirements.txt"
|
|
]
|
|
RUN_COMMANDS = ["cd wifite2; sudo wifite"]
|
|
PROJECT_URL = "https://github.com/derv82/wifite2"
|
|
|
|
|
|
class EvilTwin(HackingTool):
|
|
TITLE = "EvilTwin"
|
|
DESCRIPTION = "Fakeap is a script to perform Evil Twin Attack, by getting" \
|
|
" credentials using a Fake page and Fake Access Point"
|
|
INSTALL_COMMANDS = ["sudo git clone https://github.com/Z4nzu/fakeap.git"]
|
|
RUN_COMMANDS = ["cd fakeap && sudo bash fakeap.sh"]
|
|
PROJECT_URL = "https://github.com/Z4nzu/fakeap"
|
|
|
|
|
|
class Fastssh(HackingTool):
|
|
TITLE = "Fastssh"
|
|
DESCRIPTION = "Fastssh is an Shell Script to perform multi-threaded scan" \
|
|
" \n and brute force attack against SSH protocol using the " \
|
|
"most commonly credentials."
|
|
INSTALL_COMMANDS = [
|
|
"sudo git clone https://github.com/Z4nzu/fastssh.git && cd fastssh && sudo chmod +x fastssh.sh",
|
|
"sudo apt-get install -y sshpass netcat"
|
|
]
|
|
RUN_COMMANDS = ["cd fastssh && sudo bash fastssh.sh --scan"]
|
|
PROJECT_URL = "https://github.com/Z4nzu/fastssh"
|
|
|
|
|
|
class Howmanypeople(HackingTool):
|
|
TITLE = "Howmanypeople"
|
|
DESCRIPTION = "Count the number of people around you by monitoring wifi " \
|
|
"signals.\n" \
|
|
"[@] WIFI ADAPTER REQUIRED* \n[*]" \
|
|
"It may be illegal to monitor networks for MAC addresses, \n" \
|
|
"especially on networks that you do not own. " \
|
|
"Please check your country's laws"
|
|
INSTALL_COMMANDS = [
|
|
"sudo apt-get install tshark"
|
|
";sudo pip install howmanypeoplearearound"
|
|
]
|
|
RUN_COMMANDS = ["sudo howmanypeoplearearound"]
|
|
|
|
|
|
class WirelessAttackTools(HackingToolsCollection):
|
|
TITLE = "Wireless attack tools"
|
|
DESCRIPTION = ""
|
|
TOOLS = [
|
|
WIFIPumpkin(),
|
|
pixiewps(),
|
|
BluePot(),
|
|
Fluxion(),
|
|
Wifiphisher(),
|
|
Wifite(),
|
|
EvilTwin(),
|
|
Fastssh(),
|
|
Howmanypeople()
|
|
]
|