mirror of
https://github.com/Kong/insomnia
synced 2024-11-08 14:49:53 +00:00
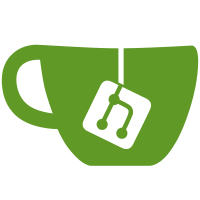
* VCS proof of concept underway! * Stuff * Some things * Replace deprecated Electron makeSingleInstance * Rename `window` variables so not to be confused with window object * Don't unnecessarily update request when URL does not change * Regenerate package-lock * Fix tests + ESLint * Publish - insomnia-app@1.0.49 - insomnia-cookies@0.0.12 - insomnia-httpsnippet@1.16.18 - insomnia-importers@2.0.13 - insomnia-libcurl@0.0.23 - insomnia-prettify@0.1.7 - insomnia-url@0.1.6 - insomnia-xpath@1.0.9 - insomnia-plugin-base64@1.0.6 - insomnia-plugin-cookie-jar@1.0.8 - insomnia-plugin-core-themes@1.0.5 - insomnia-plugin-default-headers@1.1.9 - insomnia-plugin-file@1.0.7 - insomnia-plugin-hash@1.0.7 - insomnia-plugin-jsonpath@1.0.12 - insomnia-plugin-now@1.0.11 - insomnia-plugin-os@1.0.13 - insomnia-plugin-prompt@1.1.9 - insomnia-plugin-request@1.0.18 - insomnia-plugin-response@1.0.16 - insomnia-plugin-uuid@1.0.10 * Broken but w/e * Some tweaks * Big refactor. Create local snapshots and push done * POC merging and a lot of improvements * Lots of work done on initial UI/UX * Fix old tests * Atomic writes and size-based batches * Update StageEntry definition once again to be better * Factor out GraphQL query logic * Merge algorithm, history modal, other minor things * Fix test * Merge, checkout, revert w/ user changes now work * Force UI to refresh when switching branches changes active request * Rough draft pull() and some cleanup * E2EE stuff and some refactoring * Add ability to share project with team and fixed tests * VCS now created in root component and better remote project handling * Remove unused definition * Publish - insomnia-account@0.0.2 - insomnia-app@1.1.1 - insomnia-cookies@0.0.14 - insomnia-httpsnippet@1.16.20 - insomnia-importers@2.0.15 - insomnia-libcurl@0.0.25 - insomnia-prettify@0.1.9 - insomnia-sync@0.0.2 - insomnia-url@0.1.8 - insomnia-xpath@1.0.11 - insomnia-plugin-base64@1.0.8 - insomnia-plugin-cookie-jar@1.0.10 - insomnia-plugin-core-themes@1.0.7 - insomnia-plugin-file@1.0.9 - insomnia-plugin-hash@1.0.9 - insomnia-plugin-jsonpath@1.0.14 - insomnia-plugin-now@1.0.13 - insomnia-plugin-os@1.0.15 - insomnia-plugin-prompt@1.1.11 - insomnia-plugin-request@1.0.20 - insomnia-plugin-response@1.0.18 - insomnia-plugin-uuid@1.0.12 * Move some deps around * Fix Flow errors * Update package.json * Fix eslint errors * Fix tests * Update deps * bootstrap insomnia-sync * TRy fixing appveyor * Try something else * Bump lerna * try powershell * Try again * Fix imports * Fixed errors * sync types refactor * Show remote projects in workspace dropdown * Improved pulling of non-local workspaces * Loading indicators and some tweaks * Clean up sync staging modal * Some sync improvements: - No longer store stage - Upgrade Electron - Sync UI/UX improvements * Fix snyc tests * Upgraded deps and hot loader tweaks (it's broken for some reason) * Fix tests * Branches dialog, network refactoring, some tweaks * Fixed merging when other branch is empty * A bunch of small fixes from real testing * Fixed pull merge logic * Fix tests * Some bug fixes * A few small tweaks * Conflict resolution and other improvements * Fix tests * Add revert changes * Deal with duplicate projects per workspace * Some tweaks and accessibility improvements * Tooltip accessibility * Fix API endpoint * Fix tests * Remove jest dep from insomnia-importers
97 lines
2.6 KiB
JavaScript
97 lines
2.6 KiB
JavaScript
import * as React from 'react';
|
|
import ReactDOM from 'react-dom';
|
|
import { Provider } from 'react-redux';
|
|
import App from './containers/app';
|
|
import * as models from '../models';
|
|
import * as db from '../common/database';
|
|
import { init as initStore } from './redux/modules';
|
|
import * as legacySync from '../sync-legacy';
|
|
import { init as initPlugins } from '../plugins';
|
|
import './css/index.less';
|
|
import { isDevelopment } from '../common/constants';
|
|
import { setFont, setTheme } from '../plugins/misc';
|
|
import { AppContainer } from 'react-hot-loader';
|
|
import { DragDropContext } from 'react-dnd';
|
|
import DNDBackend from './dnd-backend';
|
|
|
|
// Handy little helper
|
|
document.body.setAttribute('data-platform', process.platform);
|
|
|
|
(async function() {
|
|
await db.initClient();
|
|
await initPlugins();
|
|
|
|
const settings = await models.settings.getOrCreate();
|
|
await setTheme(settings.theme);
|
|
await setFont(settings);
|
|
|
|
// Create Redux store
|
|
const store = await initStore();
|
|
|
|
const context = DragDropContext(DNDBackend);
|
|
const render = Component => {
|
|
const DnDComponent = context(Component);
|
|
ReactDOM.render(
|
|
<AppContainer>
|
|
<Provider store={store}>
|
|
<DnDComponent />
|
|
</Provider>
|
|
</AppContainer>,
|
|
document.getElementById('root'),
|
|
);
|
|
};
|
|
|
|
render(App);
|
|
|
|
// Hot Module Replacement API
|
|
if (module.hot) {
|
|
// module.hot.accept('./containers/app', () => {
|
|
// render(App);
|
|
// });
|
|
}
|
|
|
|
// Do things that can wait
|
|
const { enableSyncBeta } = await models.settings.getOrCreate();
|
|
if (enableSyncBeta) {
|
|
console.log('[app] Enabling sync beta');
|
|
legacySync.disableForSession();
|
|
} else {
|
|
process.nextTick(legacySync.init);
|
|
}
|
|
})();
|
|
|
|
// Export some useful things for dev
|
|
if (isDevelopment()) {
|
|
window.models = models;
|
|
window.db = db;
|
|
}
|
|
|
|
// Catch uncaught errors and report them
|
|
if (window && !isDevelopment()) {
|
|
window.addEventListener('error', e => {
|
|
console.error('Uncaught Error', e);
|
|
});
|
|
|
|
window.addEventListener('unhandledRejection', e => {
|
|
console.error('Unhandled Promise', e);
|
|
});
|
|
}
|
|
|
|
function showUpdateNotification() {
|
|
console.log('[app] Update Available');
|
|
|
|
// eslint-disable-next-line no-new
|
|
new window.Notification('Insomnia Update Ready', {
|
|
body: 'Relaunch the app for it to take effect',
|
|
silent: true,
|
|
sticky: true,
|
|
});
|
|
}
|
|
|
|
const { ipcRenderer } = require('electron');
|
|
ipcRenderer.on('update-available', () => {
|
|
// Give it a few seconds before showing this. Sometimes, when
|
|
// you relaunch too soon it doesn't work the first time.
|
|
setTimeout(showUpdateNotification, 1000 * 10);
|
|
});
|