mirror of
https://github.com/Kong/insomnia
synced 2024-11-08 14:49:53 +00:00
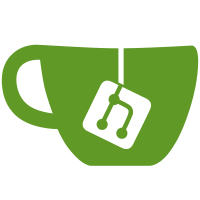
* All projects into monorepo * Update CI * More CI updates * Extracted a bunch of things into packages * Publish - insomnia-plugin-base64@1.0.1 - insomnia-plugin-default-headers@1.0.2 - insomnia-plugin-file@1.0.1 - insomnia-plugin-hash@1.0.1 - insomnia-plugin-now@1.0.1 - insomnia-plugin-request@1.0.1 - insomnia-plugin-response@1.0.1 - insomnia-plugin-uuid@1.0.1 - insomnia-cookies@0.0.2 - insomnia-importers@1.5.2 - insomnia-prettify@0.0.3 - insomnia-url@0.0.2 - insomnia-xpath@0.0.2 * A bunch of small fixes * Improved build script * Fixed * Merge dangling files * Usability refactor * Handle duplicate plugin names
72 lines
1.8 KiB
JavaScript
72 lines
1.8 KiB
JavaScript
const tag = require('..').templateTags[0];
|
||
|
||
function assertTemplate (args, expected) {
|
||
return async function () {
|
||
const result = await tag.run(null, ...args);
|
||
expect(result).toBe(expected);
|
||
};
|
||
}
|
||
|
||
function assertTemplateFails (args, expected) {
|
||
return async function () {
|
||
try {
|
||
await tag.run(null, ...args);
|
||
fail(`Render should have thrown ${expected}`);
|
||
} catch (err) {
|
||
expect(err.message).toContain(expected);
|
||
}
|
||
};
|
||
}
|
||
|
||
describe('Plugin', () => {
|
||
// Algorithms
|
||
it('hashes sha1', assertTemplate(
|
||
['sha1', 'hex', 'foo'],
|
||
'0beec7b5ea3f0fdbc95d0dd47f3c5bc275da8a33'
|
||
));
|
||
it('hashes sha256', assertTemplate(
|
||
['sha256', 'hex', 'foo'],
|
||
'2c26b46b68ffc68ff99b453c1d30413413422d706483bfa0f98a5e886266e7ae'
|
||
));
|
||
it('hashes md5', assertTemplate(
|
||
['md5', 'hex', 'foo'],
|
||
'acbd18db4cc2f85cedef654fccc4a4d8'
|
||
));
|
||
it('fails to hash invalid algorithm', assertTemplateFails(
|
||
['bad', 'hex', 'foo'],
|
||
'Digest method not supported'
|
||
));
|
||
|
||
// Digests
|
||
it('hashes to latin1', assertTemplate(
|
||
['md5', 'latin1', 'foo'],
|
||
'¬½ÛLÂø\\íïeOÌĤØ'
|
||
));
|
||
it('hashes to hex', assertTemplate(
|
||
['md5', 'hex', 'foo'],
|
||
'acbd18db4cc2f85cedef654fccc4a4d8'
|
||
));
|
||
it('hashes to base64', assertTemplate(
|
||
['md5', 'base64', 'foo'],
|
||
'rL0Y20zC+Fzt72VPzMSk2A=='
|
||
));
|
||
it('fails to hash to invalid', assertTemplateFails(
|
||
['md5', 'bad', 'foo'],
|
||
'Invalid encoding bad. Choices are hex, latin1, base64'
|
||
));
|
||
|
||
// Values
|
||
it('hashes empty string', assertTemplate(
|
||
['md5', 'hex', ''],
|
||
'd41d8cd98f00b204e9800998ecf8427e'
|
||
));
|
||
it('hashes no string', assertTemplate(
|
||
['md5', 'hex'],
|
||
'd41d8cd98f00b204e9800998ecf8427e'
|
||
));
|
||
it('fails to hash non string', assertTemplateFails(
|
||
['md5', 'hex', true],
|
||
'Cannot hash value of type "boolean"'
|
||
));
|
||
});
|