mirror of
https://github.com/Kong/insomnia
synced 2024-11-08 14:49:53 +00:00
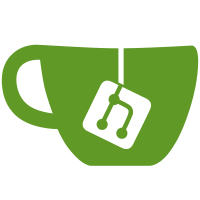
* Started on workspace settings modal * Added keyboard shortcut for workspace settings * More work on workspace settings * Actually use client certificates * Only add cmd+w on mac closes #64
32 lines
764 B
JavaScript
32 lines
764 B
JavaScript
import React, {Component, PropTypes} from 'react';
|
|
import {debounce} from '../../../common/misc';
|
|
|
|
class DebouncedInput extends Component {
|
|
_handleValueChange = debounce(this.props.onChange, 500);
|
|
_handleChange = e => this._handleValueChange(e.target.value);
|
|
|
|
render () {
|
|
// NOTE: Strip out onChange because we're overriding it
|
|
const {onChange, textarea, ...props} = this.props;
|
|
if (textarea) {
|
|
return (
|
|
<textarea {...props} onChange={this._handleChange}/>
|
|
)
|
|
} else {
|
|
return (
|
|
<input {...props} onChange={this._handleChange}/>
|
|
)
|
|
}
|
|
}
|
|
}
|
|
|
|
DebouncedInput.propTypes = {
|
|
// Required
|
|
onChange: PropTypes.func.isRequired,
|
|
|
|
// Optional
|
|
textarea: PropTypes.bool,
|
|
};
|
|
|
|
export default DebouncedInput;
|