mirror of
https://github.com/Kong/insomnia
synced 2024-11-08 14:49:53 +00:00
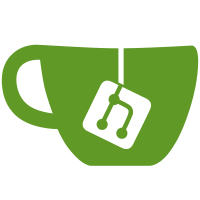
* Fixed duplication kve bug * Some changes * Add proptypes linting * Fixed proptypes even more * Filename linting
73 lines
2.1 KiB
JavaScript
73 lines
2.1 KiB
JavaScript
import React, {PureComponent} from 'react';
|
|
import autobind from 'autobind-decorator';
|
|
import PromptButton from '../base/prompt-button';
|
|
import Link from '../base/link';
|
|
import Modal from '../base/modal';
|
|
import ModalBody from '../base/modal-body';
|
|
import ModalHeader from '../base/modal-header';
|
|
import {trackEvent} from '../../../analytics';
|
|
import * as session from '../../../sync/session';
|
|
import * as sync from '../../../sync/index';
|
|
|
|
let hidePaymentNotificationUntilNextLaunch = false;
|
|
|
|
@autobind
|
|
class PaymentNotificationModal extends PureComponent {
|
|
async _handleCancel () {
|
|
await sync.cancelTrial();
|
|
this.hide();
|
|
}
|
|
|
|
_setModalRef (n) {
|
|
this.modal = n;
|
|
}
|
|
|
|
show () {
|
|
// Don't trigger automatically if user has dismissed it already
|
|
if (hidePaymentNotificationUntilNextLaunch) {
|
|
return;
|
|
}
|
|
|
|
hidePaymentNotificationUntilNextLaunch = true;
|
|
this.modal.show();
|
|
}
|
|
|
|
hide () {
|
|
trackEvent('Billing', 'Trial Ended', 'Cancel');
|
|
this.modal.hide();
|
|
}
|
|
|
|
render () {
|
|
return (
|
|
<Modal ref={this._setModalRef}>
|
|
<ModalHeader>Insomnia Plus Trial Ended</ModalHeader>
|
|
<ModalBody className="pad changelog">
|
|
<div className="text-center pad">
|
|
<h1>Hi {session.getFirstName()},</h1>
|
|
<p style={{maxWidth: '30rem', margin: 'auto'}}>
|
|
Your Insomnia Plus trial has come to an end. Please enter your billing info
|
|
to continue using Plus features like encrypted data synchronization and backup.
|
|
</p>
|
|
<br/>
|
|
<p className="pad-top">
|
|
<PromptButton onClick={this._handleCancel} className="btn btn--compact faint">
|
|
Cancel Subscription
|
|
</PromptButton>
|
|
|
|
<Link button
|
|
href="https://insomnia.rest/app/subscribe/"
|
|
className="btn btn--compact btn--outlined">
|
|
Update Billing
|
|
</Link>
|
|
</p>
|
|
</div>
|
|
</ModalBody>
|
|
</Modal>
|
|
);
|
|
}
|
|
}
|
|
|
|
PaymentNotificationModal.propTypes = {};
|
|
|
|
export default PaymentNotificationModal;
|