mirror of
https://github.com/Kong/insomnia
synced 2024-11-08 06:39:48 +00:00
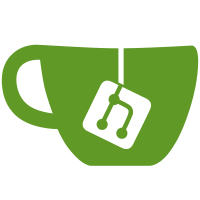
* Proof of concept authorize call * Authorize and Refresh endpoints done * OAuth2 editor started * Some small fixes * Set OAuth headers on request * Started on some OAuth tests * Updated network logic with new OAuth API * OAuth forms and refactor flows * Fix grant type handling * Moved auth handling out of render pipeline * Fixed legacy auth header * Fix vertical center * Prompt user on auth type change * Refresh tokens working (I think) and better UI * Catch same type auth change * POC refresh token and small refactor * Better token handling * LOading state to token refresh * Show o-auth-2 errors * Some minor updates
59 lines
1.1 KiB
JavaScript
59 lines
1.1 KiB
JavaScript
import * as db from '../common/database';
|
|
|
|
export const name = 'OAuth 2.0 Token';
|
|
export const type = 'OAuth2Token';
|
|
export const prefix = 'oa2';
|
|
export const canDuplicate = false;
|
|
|
|
export function init () {
|
|
return {
|
|
parentId: null,
|
|
refreshToken: null,
|
|
accessToken: null,
|
|
expiresAt: null, // Should be Date.now() if valid
|
|
|
|
// Error handling
|
|
error: null,
|
|
errorDescription: null,
|
|
errorUri: null
|
|
};
|
|
}
|
|
|
|
export function migrate (doc) {
|
|
return doc;
|
|
}
|
|
|
|
export function create (patch = {}) {
|
|
if (!patch.parentId) {
|
|
throw new Error('New OAuth2Token missing `parentId`', patch);
|
|
}
|
|
|
|
return db.docCreate(type, patch);
|
|
}
|
|
|
|
export function update (token, patch) {
|
|
return db.docUpdate(token, patch);
|
|
}
|
|
|
|
export function remove (token) {
|
|
return db.remove(token);
|
|
}
|
|
|
|
export function getByParentId (parentId) {
|
|
return db.getWhere(type, {parentId});
|
|
}
|
|
|
|
export async function getOrCreateByParentId (parentId, patch = {}) {
|
|
let token = await db.getWhere(type, {parentId});
|
|
|
|
if (!token) {
|
|
token = await create({parentId}, patch);
|
|
}
|
|
|
|
return token;
|
|
}
|
|
|
|
export function all () {
|
|
return db.all(type);
|
|
}
|