mirror of
https://github.com/Kong/insomnia
synced 2024-11-08 14:49:53 +00:00
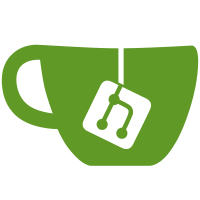
* Add digest auth and response timeline * Some css fixes * Fixed Button * More auth methods, fixed URL regex, and fix gzip * Get rid of negotiate auth * Fix proxy * Fixed GA tracking * Some very small changes and fixes * Fix content type names * Even better auth switching and timeline syntax * Some auth tweaks * CSS tweaks * Reworked toasts * Fixed timer rounding quirk * Request settings and render errors * Fixed tests * Vertical dragging and stuff * Remove beta
32 lines
709 B
JavaScript
32 lines
709 B
JavaScript
import React, {PropTypes, PureComponent} from 'react';
|
|
import {ALT_SYM, isMac, joinHotKeys, MOD_SYM, SHIFT_SYM} from '../../common/constants';
|
|
|
|
class Hotkey extends PureComponent {
|
|
render () {
|
|
const {char, shift, alt, className} = this.props;
|
|
const chars = [ ];
|
|
|
|
alt && chars.push(ALT_SYM);
|
|
shift && chars.push(SHIFT_SYM);
|
|
chars.push(MOD_SYM);
|
|
chars.push(char);
|
|
|
|
return (
|
|
<span className={`${isMac() ? 'font-normal' : ''} ${className}`}>
|
|
{joinHotKeys(chars)}
|
|
</span>
|
|
);
|
|
}
|
|
}
|
|
|
|
Hotkey.propTypes = {
|
|
char: PropTypes.string.isRequired,
|
|
|
|
// Optional
|
|
alt: PropTypes.bool,
|
|
shift: PropTypes.bool,
|
|
className: PropTypes.string
|
|
};
|
|
|
|
export default Hotkey;
|