mirror of
https://github.com/Kong/insomnia
synced 2024-11-08 14:49:53 +00:00
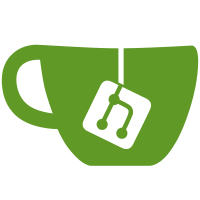
* add gitlab oauth provider and ui * add gitlab remaining * add graphql field in query * add gitlab oauth provider and ui * add gitlab remaining * add graphql field in query * add some changes for testing * add gitlab oauth provider and ui * add gitlab remaining * add graphql field in query * add some changes for testing * add some changes * modify test * try to refresh the token on git auth failure * use localStorage to retrieve the session token * simplify e2e tests for gitlab * read the gitlab config from the api * refresh the token if unauthorised * use the rest api to fetch the user's data * add loading state for config and handle 4xx errors in the ui Co-authored-by: jackkav <jackkav@gmail.com> * improve config fetching * fix(e2e): add mock route for config * Fix fetching gitlab config from the API * add src as dep to avatar component hook Co-authored-by: Mark Kim <yowmark613@gmail.com> Co-authored-by: jackkav <jackkav@gmail.com> Co-authored-by: David Marby <david@dmarby.se>
77 lines
2.2 KiB
TypeScript
77 lines
2.2 KiB
TypeScript
// Read more about creating fixtures https://playwright.dev/docs/test-fixtures
|
|
import { ElectronApplication, test as baseTest, TraceMode } from '@playwright/test';
|
|
import path from 'path';
|
|
|
|
import {
|
|
bundleType,
|
|
cwd,
|
|
executablePath,
|
|
mainPath,
|
|
randomDataPath,
|
|
} from './paths';
|
|
|
|
interface EnvOptions {
|
|
INSOMNIA_DATA_PATH: string;
|
|
INSOMNIA_API_URL: string;
|
|
INSOMNIA_APP_WEBSITE_URL: string;
|
|
INSOMNIA_GITHUB_API_URL: string;
|
|
INSOMNIA_GITLAB_API_URL: string;
|
|
}
|
|
|
|
export const test = baseTest.extend<{
|
|
app: ElectronApplication;
|
|
}>({
|
|
app: async ({ playwright, trace }, use, testInfo) => {
|
|
const webServerUrl = testInfo.config.webServer?.url;
|
|
|
|
const options: EnvOptions = {
|
|
INSOMNIA_DATA_PATH: randomDataPath(),
|
|
INSOMNIA_API_URL: webServerUrl + '/api',
|
|
INSOMNIA_APP_WEBSITE_URL: webServerUrl + '/website',
|
|
INSOMNIA_GITHUB_API_URL: webServerUrl + '/github-api/graphql',
|
|
INSOMNIA_GITLAB_API_URL: webServerUrl + '/gitlab-api',
|
|
};
|
|
|
|
const electronApp = await playwright._electron.launch({
|
|
cwd,
|
|
executablePath,
|
|
args: bundleType() === 'package' ? [] : [mainPath],
|
|
env: {
|
|
...process.env,
|
|
...options,
|
|
PLAYWRIGHT: 'true',
|
|
},
|
|
});
|
|
|
|
const appContext = electronApp.context();
|
|
const traceMode: TraceMode = typeof trace === 'string' ? trace as TraceMode : trace.mode;
|
|
|
|
const defaultTraceOptions = { screenshots: true, snapshots: true, sources: true };
|
|
const traceOptions = typeof trace === 'string' ? defaultTraceOptions : { ...defaultTraceOptions, ...trace, mode: undefined };
|
|
const captureTrace = (traceMode === 'on' || traceMode === 'retain-on-failure' || (traceMode === 'on-first-retry' && testInfo.retry === 1));
|
|
|
|
if (captureTrace) {
|
|
await appContext.tracing.start(traceOptions);
|
|
}
|
|
|
|
await use(electronApp);
|
|
|
|
if (captureTrace) {
|
|
await appContext.tracing.stop({
|
|
path: path.join(testInfo.outputDir, 'trace.zip'),
|
|
});
|
|
}
|
|
|
|
await electronApp.close();
|
|
},
|
|
page: async ({ app }, use) => {
|
|
const page = await app.firstWindow();
|
|
|
|
await page.waitForLoadState();
|
|
|
|
await page.click("text=Don't share usage analytics");
|
|
|
|
await use(page);
|
|
},
|
|
});
|