mirror of
https://github.com/Kong/insomnia
synced 2024-11-08 14:49:53 +00:00
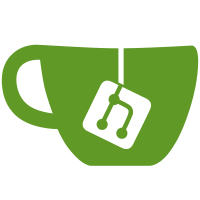
* Proof of concept authorize call * Authorize and Refresh endpoints done * OAuth2 editor started * Some small fixes * Set OAuth headers on request * Started on some OAuth tests * Updated network logic with new OAuth API * OAuth forms and refactor flows * Fix grant type handling * Moved auth handling out of render pipeline * Fixed legacy auth header * Fix vertical center * Prompt user on auth type change * Refresh tokens working (I think) and better UI * Catch same type auth change * POC refresh token and small refactor * Better token handling * LOading state to token refresh * Show o-auth-2 errors * Some minor updates
63 lines
1.3 KiB
JavaScript
63 lines
1.3 KiB
JavaScript
import electron from 'electron';
|
|
import querystring from 'querystring';
|
|
|
|
export function responseToObject (body, keys) {
|
|
let data = null;
|
|
try {
|
|
data = JSON.parse(body);
|
|
} catch (err) {
|
|
}
|
|
|
|
if (!data) {
|
|
try {
|
|
data = querystring.parse(body);
|
|
} catch (err) {
|
|
}
|
|
}
|
|
|
|
let results = {};
|
|
for (const key of keys) {
|
|
const value = typeof data[key] === 'string' ? data[key] : null;
|
|
results[key] = value;
|
|
}
|
|
|
|
return results;
|
|
}
|
|
|
|
export function authorizeUserInWindow (url, urlRegex = /.*/) {
|
|
return new Promise((resolve, reject) => {
|
|
let finalUrl = null;
|
|
|
|
// Create a child window
|
|
const child = new electron.remote.BrowserWindow({
|
|
webPreferences: {
|
|
nodeIntegration: false,
|
|
partition: `persist:oauth2`
|
|
},
|
|
show: false
|
|
});
|
|
|
|
// Finish on close
|
|
child.on('close', () => {
|
|
if (finalUrl) {
|
|
resolve(finalUrl);
|
|
} else {
|
|
reject(new Error('Authorization window closed'));
|
|
}
|
|
});
|
|
|
|
// Catch the redirect after login
|
|
child.webContents.on('did-navigate', () => {
|
|
const url = child.webContents.getURL();
|
|
if (url.match(urlRegex)) {
|
|
finalUrl = url;
|
|
child.close();
|
|
}
|
|
});
|
|
|
|
// Show the window to the user after it loads
|
|
child.on('ready-to-show', child.show.bind(child));
|
|
child.loadURL(url);
|
|
});
|
|
}
|