mirror of
https://github.com/Kong/insomnia
synced 2024-11-08 23:00:30 +00:00
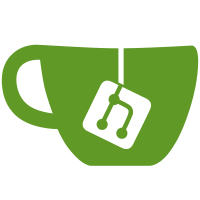
* Most things are working * Cookies and lots of improvements * Hoist regex * Appveyor test * Fix appveyor file * Remove yarn * Test deps * Fix * Remove all git deps * fix * Fix * Add cash rm * Fix command * Fix * Production * try * fix * fix? * Done * Remove cache * tweak * Shortcut * Fix * Attempt * try * Try clone depth * Try again * back to shallow_clone * Some debugging * Another try * Try something else * Another * try curl only * Another * fix * Update node-gyp * Try again * Try * Again * Some pruning * Back to the start * Again * try config * Reorder test * Try without cache * Update dep * Try again * remove shallow_clone * don't run tests * Implement CA fetching * Fix * Use CAs * Fix? * test * Remove build dir * Fix dir path? * Try again * Aagain * Try * Change dep * Add comment * Invalidate cache * Remove yarn * Fix cookie expiry * fixed tests * Some modal fixes and unrelated stuff * Debounce autocomplete * Got client certificates working * Fixed binary files * Fixed cookies * Got proxy and debug info working * Got CA certs working * Update appveyor build * Fixed cookie date parsing and overlay z-index * Test updates * Autocomplete to bulk header editor * Small CSS tweak
117 lines
2.8 KiB
JavaScript
117 lines
2.8 KiB
JavaScript
import React, {PropTypes, PureComponent} from 'react';
|
|
import autobind from 'autobind-decorator';
|
|
import EnvironmentEditor from '../editors/environment-editor';
|
|
import Modal from '../base/modal';
|
|
import ModalBody from '../base/modal-body';
|
|
import ModalHeader from '../base/modal-header';
|
|
import ModalFooter from '../base/modal-footer';
|
|
|
|
@autobind
|
|
class EnvironmentEditModal extends PureComponent {
|
|
constructor (props) {
|
|
super(props);
|
|
this.state = {
|
|
requestGroup: null,
|
|
isValid: true
|
|
};
|
|
}
|
|
|
|
_setModalRef (n) {
|
|
this.modal = n;
|
|
}
|
|
|
|
_setEditorRef (n) {
|
|
this._envEditor = n;
|
|
}
|
|
|
|
_saveChanges () {
|
|
if (!this._envEditor.isValid()) {
|
|
return;
|
|
}
|
|
|
|
const environment = this._envEditor.getValue();
|
|
const {requestGroup} = this.state;
|
|
|
|
this.props.onChange(Object.assign({}, requestGroup, {environment}));
|
|
}
|
|
|
|
_didChange () {
|
|
this._saveChanges();
|
|
|
|
const isValid = this._envEditor.isValid();
|
|
|
|
if (this.state.isValid !== isValid) {
|
|
this.setState({isValid});
|
|
}
|
|
}
|
|
|
|
show (requestGroup) {
|
|
this.modal.show();
|
|
this.setState({requestGroup});
|
|
}
|
|
|
|
hide () {
|
|
this.modal.hide();
|
|
}
|
|
|
|
toggle (requestGroup) {
|
|
this.modal.toggle();
|
|
this.setState({requestGroup});
|
|
}
|
|
|
|
render () {
|
|
const {
|
|
editorKeyMap,
|
|
editorFontSize,
|
|
lineWrapping,
|
|
render,
|
|
getRenderContext,
|
|
...extraProps
|
|
} = this.props;
|
|
|
|
const {
|
|
requestGroup,
|
|
isValid
|
|
} = this.state;
|
|
|
|
return (
|
|
<Modal ref={this._setModalRef} tall {...extraProps}>
|
|
<ModalHeader>Environment Overrides (JSON Format)</ModalHeader>
|
|
<ModalBody noScroll>
|
|
<EnvironmentEditor
|
|
lightTheme
|
|
editorFontSize={editorFontSize}
|
|
editorKeyMap={editorKeyMap}
|
|
ref={this._setEditorRef}
|
|
key={requestGroup ? requestGroup._id : 'n/a'}
|
|
lineWrapping={lineWrapping}
|
|
environment={requestGroup ? requestGroup.environment : {}}
|
|
didChange={this._didChange}
|
|
render={render}
|
|
getRenderContext={getRenderContext}
|
|
/>
|
|
</ModalBody>
|
|
<ModalFooter>
|
|
<div className="margin-left faint italic txt-sm">
|
|
* this can be used to override data in the global environment
|
|
</div>
|
|
<button className="btn" disabled={!isValid} onClick={this.hide}>
|
|
Done
|
|
</button>
|
|
</ModalFooter>
|
|
</Modal>
|
|
);
|
|
}
|
|
}
|
|
|
|
EnvironmentEditModal.propTypes = {
|
|
onChange: PropTypes.func.isRequired,
|
|
editorFontSize: PropTypes.number.isRequired,
|
|
editorKeyMap: PropTypes.string.isRequired,
|
|
render: PropTypes.func.isRequired,
|
|
getRenderContext: PropTypes.func.isRequired,
|
|
lineWrapping: PropTypes.bool.isRequired
|
|
};
|
|
|
|
export default EnvironmentEditModal;
|