mirror of
https://github.com/Kong/insomnia
synced 2024-11-08 14:49:53 +00:00
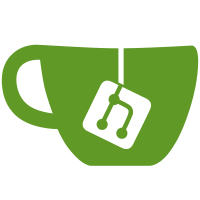
* Maybe working POC * Change to use remote url * Other URL too * Some logic * Got the push part working * Made some updates * Fix * Update * Add status code check * Stuff * Implemented new sync api * A bit more robust * Debounce changes * Change timeout * Some fixes * Remove .less * Better error handling * Fix base url * Support for created vs updated docs * Try silent * Silence removal too * Small fix after merge * Fix test * Stuff * Implement key generation algorithm * Tidy * stuff * A bunch of stuff for the new API * Integrated the session stuff * Stuff * Just started on encryption * Lots of updates to encryption * Finished createResourceGroup function * Full encryption/decryption working (I think) * Encrypt localstorage with sessionID * Some more * Some extra checks * Now uses separate DB. Still needs to be simplified a LOT * Fix deletion bug * Fixed unicode bug with encryption * Simplified and working * A bunch of polish * Some stuff * Removed some workspace meta properties * Migrated a few more meta properties * Small changes * Fix body scrolling and url cursor jumping * Removed duplication of webpack port * Remove workspaces reduces * Some small fixes * Added sync modal and opt-in setting * Good start to sync flow * Refactored modal footer css * Update sync status * Sync logger * A bit better logging * Fixed a bunch of sync-related bugs * Fixed signup form button * Gravatar component * Split sync modal into tabs * Tidying * Some more error handling * start sending 'user agent * Login/signup error handling * Use real UUIDs * Fixed tests * Remove unused function * Some extra checks * Moved cloud sync setting to about page * Some small changes * Some things
146 lines
2.8 KiB
JavaScript
146 lines
2.8 KiB
JavaScript
import electron from 'electron';
|
|
import NeDB from 'nedb';
|
|
import fsPath from 'path';
|
|
import crypto from 'crypto';
|
|
|
|
/**
|
|
* Get all Resources
|
|
*
|
|
* @returns {Promise}
|
|
*/
|
|
export function allResources () {
|
|
return new Promise((resolve, reject) => {
|
|
_getDB().find({}, (err, rawDocs) => {
|
|
if (err) {
|
|
reject(err);
|
|
} else {
|
|
resolve(rawDocs);
|
|
}
|
|
})
|
|
});
|
|
}
|
|
|
|
/**
|
|
* Find resources by query
|
|
*
|
|
* @returns {Promise}
|
|
*/
|
|
export function findResources (query) {
|
|
return new Promise((resolve, reject) => {
|
|
_getDB().find(query, (err, rawDocs) => {
|
|
if (err) {
|
|
reject(err);
|
|
} else {
|
|
resolve(rawDocs);
|
|
}
|
|
})
|
|
});
|
|
}
|
|
|
|
/**
|
|
* Get all dirty resources
|
|
* @returns {Promise}
|
|
*/
|
|
export function findDirtyResources () {
|
|
return findResources({dirty: true});
|
|
}
|
|
|
|
/**
|
|
* Get Resource by resourceID
|
|
*
|
|
* @param id
|
|
* @returns {Promise}
|
|
*/
|
|
export function getResourceById (id) {
|
|
return new Promise((resolve, reject) => {
|
|
// TODO: this query should probably include resourceGroupId as well
|
|
_getDB().find({id}, (err, rawDocs) => {
|
|
if (err) {
|
|
reject(err);
|
|
} else {
|
|
resolve(rawDocs.length >= 1 ? rawDocs[0] : null);
|
|
}
|
|
});
|
|
})
|
|
}
|
|
|
|
/**
|
|
* Create a new Resource
|
|
*
|
|
* @param resource
|
|
*/
|
|
export function insertResource (resource) {
|
|
return new Promise((resolve, reject) => {
|
|
const h = crypto.createHash('md5');
|
|
h.update(resource.resourceGroupId);
|
|
h.update(resource.id);
|
|
resource._id = `rs_${h.digest('hex')}`;
|
|
_getDB().insert(resource, (err, newDoc) => {
|
|
if (err) {
|
|
reject(err);
|
|
} else {
|
|
resolve(newDoc);
|
|
}
|
|
});
|
|
});
|
|
}
|
|
|
|
/**
|
|
* Update an existing resource
|
|
*
|
|
* @param resource
|
|
* @param patches
|
|
* @returns {Promise}
|
|
*/
|
|
export function updateResource (resource, ...patches) {
|
|
return new Promise((resolve, reject) => {
|
|
const updatedResource = Object.assign(resource, ...patches);
|
|
_getDB().update({_id: resource._id}, updatedResource, err => {
|
|
if (err) {
|
|
reject(err);
|
|
} else {
|
|
resolve(resource);
|
|
}
|
|
});
|
|
});
|
|
}
|
|
|
|
/**
|
|
* Remove an existing resource
|
|
*
|
|
* @param resource
|
|
* @returns {Promise}
|
|
*/
|
|
export function removeResource (resource) {
|
|
return new Promise((resolve, reject) => {
|
|
_getDB().remove({_id: resource._id}, err => {
|
|
if (err) {
|
|
reject(err);
|
|
} else {
|
|
resolve();
|
|
}
|
|
});
|
|
});
|
|
}
|
|
|
|
// ~~~~~~~ //
|
|
// Helpers //
|
|
// ~~~~~~~ //
|
|
|
|
let _database = null;
|
|
function _getDB () {
|
|
if (!_database) {
|
|
// NOTE: Do not EVER change this. EVER!
|
|
const basePath = electron.remote.app.getPath('userData');
|
|
const filePath = fsPath.join(basePath, `insomnia.sync.resources.db`);
|
|
|
|
// Fill in the defaults
|
|
_database = new NeDB({filename: filePath, autoload: true});
|
|
|
|
// Done
|
|
console.log(`-- Initialize Sync DB at ${filePath} --`);
|
|
}
|
|
|
|
return _database;
|
|
}
|