mirror of
https://github.com/Kong/insomnia
synced 2024-11-08 23:00:30 +00:00
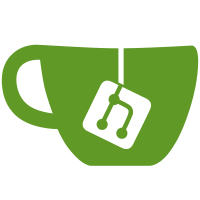
* Lifting path clicked up to sidebar root * Abstracting out path click to story (and app) * Adding click to scroll, updating sidebar components * Scroll fix for sidebar * Cleaning up log * PR feedback * PR Feedback, fix schemas & request body rendering * PR feedback & cleanup * Prop value checking, selection positioning * Pulling remote import * Styled components into insomnia-app, obj destructuring, typing * Refactoring item path mapping * Abstracting the mapping of specs for position, bumping down styled components version. * Directly passing ... rest args initial work * Pulling logs, removing array concatenation * Pulling click handler out of render method * Rolling position mapping into scroll positining method * Opening up type, could be number/string/array * Update package-lock.json * Grabbing height from window, typing API vars, checking path * Creating scroll method dedicated to design mode * Moving typing of spec related content to Props def. * Type checking on sections, invalid section component
51 lines
1.5 KiB
JavaScript
51 lines
1.5 KiB
JavaScript
// @flow
|
|
import * as React from 'react';
|
|
import SidebarItem from './sidebar-item';
|
|
import SvgIcon, { IconEnum } from '../svg-icon';
|
|
import SidebarSection from './sidebar-section';
|
|
import StyledInvalidSection from './sidebar-invalid-section';
|
|
|
|
type Props = {
|
|
security: Object,
|
|
onClick: (section: string, ...args: any) => void,
|
|
};
|
|
|
|
// Implemented as a class component because of a caveat with render props
|
|
// https://reactjs.org/docs/render-props.html#be-careful-when-using-render-props-with-reactpurecomponent
|
|
export default class SidebarSecurity extends React.Component<Props> {
|
|
renderBody = (filter: string): null | React.Node => {
|
|
const { security, onClick } = this.props;
|
|
|
|
if (Object.prototype.toString.call(security) !== '[object Object]') {
|
|
return <StyledInvalidSection name={'security'} />;
|
|
}
|
|
|
|
const filteredValues = Object.keys(security).filter(scheme =>
|
|
scheme.toLowerCase().includes(filter.toLocaleLowerCase()),
|
|
);
|
|
|
|
if (!filteredValues.length) {
|
|
return null;
|
|
}
|
|
|
|
return (
|
|
<div>
|
|
{filteredValues.map(scheme => (
|
|
<React.Fragment key={scheme}>
|
|
<SidebarItem>
|
|
<div>
|
|
<SvgIcon icon={IconEnum.key} />
|
|
</div>
|
|
<span onClick={() => onClick('components', 'securitySchemes', scheme)}>{scheme}</span>
|
|
</SidebarItem>
|
|
</React.Fragment>
|
|
))}
|
|
</div>
|
|
);
|
|
};
|
|
|
|
render() {
|
|
return <SidebarSection title="SECURITY" renderBody={this.renderBody} />;
|
|
}
|
|
}
|