mirror of
https://github.com/Kong/insomnia
synced 2024-11-07 22:30:15 +00:00
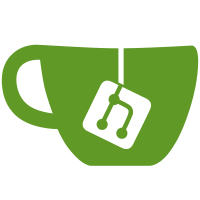
* Start working on insomnia-testing package to generate Mocha code * Moved some things around * Integration tests and ability to run multiple files * Fix integration tests * Rename runSuite to runMochaTests * Add types for test results * Fix lint errors * Play with Chia assertions * insomnia and chai globals, plus a bunch of improvements * Stub chai and axios Flow types * Ability to reference requests by ID * Fix chai flow-typed location * Address PR comments (small tweaks) * Basic UI going * Lots and lots and lots * Pretty-much done with the unit test UI * Minor CSS tweak * Activity bar triple toggle and more * Minor tweak * Unit Test and Suite deletion * Bump Designer version * Fix eslint stuff * Fix insomnia-testing tests * Fix tests * lib@2.2.9 * Remove tests tab from response pane * Hook up Insomnia networking * Fix linting * Bump version for alpha * Remove extra ActivityToggleSwitch * Remove unused import * Add test:pre-release script that excludes CLI tests * Less repetition * Clean some things up * Tweaks after self-cr * Undo request ID tag * Swap out switch for new activity toggle component * Extra check * Remove dead code * Delete dead test * Oops, revert example code * PR feedback * More PR comment addresses
144 lines
3.9 KiB
JavaScript
144 lines
3.9 KiB
JavaScript
const { jarFromCookies, cookiesFromJar } = require('insomnia-cookies');
|
|
const tag = require('..').templateTags[0];
|
|
|
|
describe('plugin', () => {
|
|
describe('RequestExtension cookie', () => {
|
|
it('should get cookie by name', async () => {
|
|
const jar = jarFromCookies([]);
|
|
jar.setCookieSync(
|
|
[
|
|
'foo=bar',
|
|
'path=/',
|
|
'domain=.insomnia.rest',
|
|
'HttpOnly Cache-Control: public, no-cache',
|
|
].join('; '),
|
|
'https://insomnia.rest',
|
|
);
|
|
|
|
const cookies = await cookiesFromJar(jar);
|
|
const requests = [{ _id: 'req_1', parameters: [], url: 'https://insomnia.rest/foo/bar' }];
|
|
const jars = [{ _id: 'jar_1', parentId: 'wrk_1', cookies }];
|
|
const context = _getTestContext([{ _id: 'wrk_1' }], requests, jars);
|
|
const result = await tag.run(context, 'cookie', 'foo');
|
|
|
|
expect(result).toBe('bar');
|
|
});
|
|
});
|
|
|
|
describe('RequestExtension url', () => {
|
|
it('should get url', async () => {
|
|
const requests = [
|
|
{
|
|
_id: 'req_1',
|
|
parameters: [{ name: 'foo', value: 'bar' }],
|
|
url: 'https://insomnia.rest/foo/bar',
|
|
},
|
|
];
|
|
const context = _getTestContext([{ _id: 'wrk_1' }], requests);
|
|
const result = await tag.run(context, 'url');
|
|
|
|
expect(result).toBe('https://insomnia.rest/foo/bar?foo=bar');
|
|
});
|
|
|
|
it('should get rendered url', async () => {
|
|
const requests = [
|
|
{
|
|
_id: 'req_1',
|
|
parameters: [{ name: 'foo', value: '{{ foo }}' }],
|
|
url: 'https://insomnia.rest/foo/bar',
|
|
},
|
|
];
|
|
const context = _getTestContext([{ _id: 'wrk_1' }], requests);
|
|
const result = await tag.run(context, 'url');
|
|
|
|
expect(result).toBe('https://insomnia.rest/foo/bar?foo=bar');
|
|
});
|
|
});
|
|
|
|
describe('RequestExtension header', () => {
|
|
it('should get url', async () => {
|
|
const requests = [
|
|
{
|
|
_id: 'req_1',
|
|
headers: [{ name: 'foo', value: '{{ foo }}' }],
|
|
url: 'https://insomnia.rest/foo/bar',
|
|
},
|
|
];
|
|
const context = _getTestContext([{ _id: 'wrk_1' }], requests);
|
|
const result = await tag.run(context, 'header', 'foo');
|
|
|
|
expect(result).toBe('bar');
|
|
});
|
|
});
|
|
|
|
describe('RequestExtension parameter', () => {
|
|
it('should get parameter', async () => {
|
|
const requests = [
|
|
{
|
|
_id: 'req_1',
|
|
parameters: [{ name: 'foo', value: '{{ foo }}' }],
|
|
url: 'https://insomnia.rest/foo/bar',
|
|
},
|
|
];
|
|
const context = _getTestContext([{ _id: 'wrk_1' }], requests);
|
|
const result = await tag.run(context, 'parameter', 'foo');
|
|
|
|
expect(result).toBe('bar');
|
|
});
|
|
});
|
|
|
|
describe('RequestExtension name', () => {
|
|
it('should get name', async () => {
|
|
const requests = [
|
|
{
|
|
_id: 'req_1',
|
|
name: 'Foo',
|
|
parameters: [{ name: 'foo', value: '{{ foo }}' }],
|
|
url: 'https://insomnia.rest/foo/bar',
|
|
},
|
|
];
|
|
const context = _getTestContext([{ _id: 'wrk_1' }], requests);
|
|
const result = await tag.run(context, 'name');
|
|
|
|
expect(result).toBe('Foo');
|
|
});
|
|
});
|
|
});
|
|
|
|
function _getTestContext(workspaces, requests, jars) {
|
|
jars = jars || [];
|
|
return {
|
|
meta: {
|
|
requestId: requests[0]._id,
|
|
workspaceId: workspaces[0]._id,
|
|
},
|
|
util: {
|
|
render(str) {
|
|
return str.replace(/{{ foo }}/g, 'bar');
|
|
},
|
|
models: {
|
|
request: {
|
|
getById(id) {
|
|
return requests.find(r => r._id === id);
|
|
},
|
|
},
|
|
workspace: {
|
|
getById(id) {
|
|
return workspaces.find(w => w._id === id);
|
|
},
|
|
},
|
|
cookieJar: {
|
|
getOrCreateForWorkspace(workspace) {
|
|
return (
|
|
jars.find(j => j.parentId === workspace._id) || {
|
|
parentId: workspace._id,
|
|
cookies: [],
|
|
}
|
|
);
|
|
},
|
|
},
|
|
},
|
|
},
|
|
};
|
|
}
|