mirror of
https://github.com/Kong/insomnia
synced 2024-11-08 06:39:48 +00:00
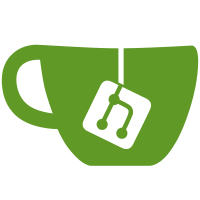
* project ui changes * project ui changes * create mock server model * model mock server similarly to design doc * use spec modelling and nav * layout pass * rename requestbin to mock-route * sidebar ui pass * load mock server * add url bar * can navigate to headers * Refactor mock server and mock route creation and retrieval * route crud * sidebar layout * add delete guuard * patch route * Add mock response tab to request pane * wire up mock servers in requests * Update mock server and route selection in RequestPane * make it work without internet * can create bin * pass body and headers to bin * can fetch logs but cant see em * split out response pane for hmr * basic table * extract mock url bar * add header tab * made a dumb cache * url bar pass * send request and create response * wire up timeline * wire up preview * timeline useeffect * move to action * fix types * empty states * rebase updating aria * use har type * can edit bins * cookie support * wire up status * status text * magic status text * ui * always use put rather than create bin * add url to mock route * scroll bar * add content types * validation * fix flake * improve logs * fix outlet warning * fix send to mock endpoint * switch table to grid * handle errors * rotate log * create mockbin on open if needed * add full url ux * reverse log order * binId from store * remove http method * rename prefix * use server Id for bin id * fix copy * show log har * fix url bar * fix button padding * tailwind * method select * remove default status text * full tailwind * fix breadcrumb * default to json * move copy to end, remove save * error msg * only patch when needed * fix ws colors * fix command palette * add isMock helper * revert local storage mechanism * fix redirect * fix ignore upsert * extract to constant * ui test * hide actions from mock-server * fix code editor onBlur * lift update to route * refactor to return only errors * add url to mock server model * select mock ui pass * can modify url in settings * use server url from db if selected * hide url option * fix lint error * extract to file * remove binResponse * can sync * move things around * rename name path sync * fix type check * capture kvp onBlur * fix error message * basic mock test * wire up mock patcher and navigate * rename component * remove url prop of route * fix lint * fix test * temporary skip e2e test * fix constant url * fix migration * remove console logs * rename function * only create a single hidden request --------- Co-authored-by: gatzjames <jamesgatzos@gmail.com>
159 lines
7.8 KiB
TypeScript
159 lines
7.8 KiB
TypeScript
import { expect } from '@playwright/test';
|
|
|
|
import { loadFixture } from '../../playwright/paths';
|
|
import { test } from '../../playwright/test';
|
|
|
|
test.describe('Dashboard', async () => {
|
|
test.slow(process.platform === 'darwin' || process.platform === 'win32', 'Slow app start on these platforms');
|
|
test.describe('Projects', async () => {
|
|
test('Can create, rename and delete new project', async ({ page }) => {
|
|
await page.getByLabel('All Files (0)').click();
|
|
await expect(page.locator('.app')).not.toContainText('Git Sync');
|
|
await expect(page.locator('.app')).not.toContainText('Setup Git Sync');
|
|
|
|
// Create new project
|
|
await page.getByRole('button', { name: 'Create new Project' }).click();
|
|
await page.getByRole('button', { name: 'Create', exact: true }).click();
|
|
|
|
// Check empty project
|
|
await expect(page.locator('.app')).toContainText('This is an empty project, to get started create your first resource:');
|
|
|
|
// Rename Project
|
|
await page.getByRole('row', { name: 'My Project' }).getByRole('button', { name: 'Project Actions' }).click();
|
|
await page.getByRole('menuitemradio', { name: 'Settings' }).click();
|
|
await page.getByPlaceholder('My Project').click();
|
|
await page.getByPlaceholder('My Project').fill('My Project123');
|
|
await page.getByRole('button', { name: 'Update' }).click();
|
|
|
|
// Check that the project name is updated on modal
|
|
await expect(page.locator('.app')).toContainText('My Project123');
|
|
|
|
// Close project settings modal
|
|
await page.locator('.app').press('Escape');
|
|
await expect(page.locator('.app')).toContainText('My Project123');
|
|
|
|
// Delete project
|
|
await page.getByRole('row', { name: 'My Project' }).getByRole('button', { name: 'Project Actions' }).click();
|
|
await page.getByRole('menuitemradio', { name: 'Delete' }).click();
|
|
await page.getByRole('button', { name: 'Delete' }).click();
|
|
|
|
// After deleting project, return to default Insomnia Dashboard
|
|
await expect(page.locator('.app')).toContainText('Personal Workspace');
|
|
await expect(page.locator('.app')).not.toContainText('My Project123');
|
|
await expect(page.locator('.app')).toContainText('New Document');
|
|
await page.getByLabel('All Files (0)').click();
|
|
await expect(page.locator('.app')).not.toContainText('Setup Git Sync');
|
|
});
|
|
});
|
|
test.describe('Interactions', async () => { // Not sure about the name here
|
|
// TODO(INS-2504) - we don't support importing multiple collections at this time
|
|
test.skip('Can filter through multiple collections', async ({ app, page }) => {
|
|
await page.getByLabel('All Files (0)').click();
|
|
await expect(page.locator('.app')).not.toContainText('Git Sync');
|
|
await expect(page.locator('.app')).not.toContainText('Setup Git Sync');
|
|
|
|
await page.getByRole('button', { name: 'Create in project' }).click();
|
|
const text = await loadFixture('multiple-workspaces.yaml');
|
|
await app.evaluate(async ({ clipboard }, text) => clipboard.writeText(text), text);
|
|
await page.getByRole('menuitemradio', { name: 'Import' }).click();
|
|
await page.locator('[data-test-id="import-from-clipboard"]').click();
|
|
await page.getByRole('button', { name: 'Scan' }).click();
|
|
await page.getByRole('dialog').getByRole('button', { name: 'Import' }).click();
|
|
await page.getByText('CollectionSmoke testsjust now').click();
|
|
// Check that 10 new workspaces are imported besides the default one
|
|
const workspaceCards = page.getByLabel('Workspaces').getByRole('gridcell');
|
|
await expect(workspaceCards).toHaveCount(11);
|
|
await expect(page.locator('.app')).toContainText('New Document');
|
|
await expect(page.locator('.app')).toContainText('collection 1');
|
|
await expect(page.locator('.app')).toContainText('design doc 1');
|
|
await expect(page.locator('.app')).toContainText('Swagger Petstore V3 JSON 1.0.0');
|
|
await expect(page.locator('.app')).toContainText('Swagger Petstore V3 YAML 1.0.0');
|
|
|
|
// Filter by collection
|
|
const filter = page.locator('[placeholder="Filter\\.\\.\\."]');
|
|
|
|
// Filter by word with results expected
|
|
await filter.fill('design');
|
|
await expect(page.locator('.card-badge')).toHaveCount(4);
|
|
|
|
// Filter by number
|
|
await filter.fill('3');
|
|
await expect(page.locator('.card-badge')).toHaveCount(2);
|
|
|
|
// Filter by word with no results expected
|
|
await filter.fill('invalid');
|
|
await expect(page.locator('.card-badge')).toHaveCount(0);
|
|
});
|
|
|
|
test('Can create, rename and delete a document', async ({ page }) => {
|
|
await page.getByLabel('All Files (0)').click();
|
|
await expect(page.locator('.app')).not.toContainText('Git Sync');
|
|
await expect(page.locator('.app')).not.toContainText('Setup Git Sync');
|
|
|
|
// Create new document
|
|
await page.getByRole('button', { name: 'Create in project' }).click();
|
|
await page.getByRole('menuitemradio', { name: 'Design Document' }).click();
|
|
await page.getByRole('button', { name: 'Create', exact: true }).click();
|
|
|
|
await page.getByTestId('project').click();
|
|
|
|
// Rename document
|
|
await page.getByLabel('my-spec.yaml').getByRole('button').click();
|
|
await page.getByRole('menuitem', { name: 'Rename' }).click();
|
|
await page.locator('text=Rename DocumentName Rename >> input[type="text"]').fill('test123');
|
|
await page.click('#root button:has-text("Rename")');
|
|
await expect(page.locator('.app')).toContainText('test123');
|
|
|
|
// Duplicate document
|
|
await page.getByLabel('test123').getByRole('button').click();
|
|
await page.getByRole('menuitem', { name: 'Duplicate' }).click();
|
|
await page.locator('input[name="name"]').fill('test123-duplicate');
|
|
await page.click('[role="dialog"] button:has-text("Duplicate")');
|
|
|
|
await page.getByTestId('project').click();
|
|
|
|
// Delete document
|
|
await page.getByLabel('test123-duplicate').getByRole('button').click();
|
|
await page.getByRole('menuitem', { name: 'Delete' }).click();
|
|
await page.getByRole('button', { name: 'Delete' }).click();
|
|
// @TODO: Re-enable - Requires mocking VCS operations
|
|
// await expect(workspaceCards).toHaveCount(1);
|
|
});
|
|
|
|
test('Can create, rename and delete a collection', async ({ page }) => {
|
|
await page.getByLabel('All Files (0)').click();
|
|
await expect(page.locator('.app')).not.toContainText('Git Sync');
|
|
await expect(page.locator('.app')).not.toContainText('Setup Git Sync');
|
|
|
|
// Create new collection
|
|
await page.getByRole('button', { name: 'Create in project' }).click();
|
|
await page.getByRole('menuitemradio', { name: 'Request Collection' }).click();
|
|
await page.getByRole('button', { name: 'Create', exact: true }).click();
|
|
|
|
await page.getByTestId('project').click();
|
|
|
|
// Rename collection
|
|
await page.click('text=CollectionMy Collectionjust now >> button');
|
|
await page.getByRole('menuitem', { name: 'Rename' }).click();
|
|
await page.locator('text=Rename CollectionName Rename >> input[type="text"]').fill('test123');
|
|
await page.click('#root button:has-text("Rename")');
|
|
await expect(page.locator('.app')).toContainText('test123');
|
|
|
|
// Duplicate collection
|
|
await page.getByLabel('test123').getByRole('button').click();
|
|
await page.getByRole('menuitem', { name: 'Duplicate' }).click();
|
|
await page.locator('input[name="name"]').fill('test123-duplicate');
|
|
await page.click('[role="dialog"] button:has-text("Duplicate")');
|
|
|
|
await page.getByTestId('project').click();
|
|
|
|
// Delete collection
|
|
await page.getByLabel('test123-duplicate').getByRole('button').click();
|
|
await page.getByRole('menuitem', { name: 'Delete' }).click();
|
|
await page.getByRole('button', { name: 'Delete' }).click();
|
|
// @TODO: Re-enable - Requires mocking VCS operations
|
|
// await expect(workspaceCards).toHaveCount(1);
|
|
});
|
|
});
|
|
});
|