mirror of
https://github.com/Kong/insomnia
synced 2024-11-08 14:49:53 +00:00
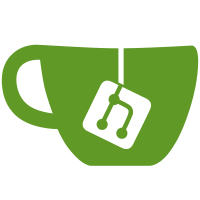
* Start on workspace dropdown and upgrade fontawesome * WorkspaceDropdown start and Elm components! * Lots of CSS shit * Refactor some db stuff and move filter out of sidebar * Adjust dropdown css * Handle duplicate header names, and stuff * Shitty cookies tab * fixed cookie table a bit * Modal refactor * Starteed cookie modal design * Better cookie storage and filter cookie modal * Cookie editor round 1 * Fix kve cursor jumping and form encoding templating * New cookies now show up in filter * Checkpoint * Stuff and fix environments css * Added manage cookies button to cookie pane * Fix accidental sidebar item drag on sidebar resize * Environments modal is looking pretty good now * Pretty much done environments nad cookies * Some changes * Fixed codemirror in modals * Fixed some things * Add basic proxy support * Updated shortcuts * Code snippet generation * Some style * bug fix * Code export now gets cookies for correct domain
83 lines
1.7 KiB
JavaScript
83 lines
1.7 KiB
JavaScript
import {combineReducers} from 'redux';
|
|
|
|
import {ALL_TYPES} from '../../database/index';
|
|
|
|
const ENTITY_INSERT = 'entities/insert';
|
|
const ENTITY_UPDATE = 'entities/update';
|
|
const ENTITY_REMOVE = 'entities/remove';
|
|
|
|
// ~~~~~~~~ //
|
|
// REDUCERS //
|
|
// ~~~~~~~~ //
|
|
|
|
function genericEntityReducer (referenceName) {
|
|
return function (state = {}, action) {
|
|
const doc = action[referenceName];
|
|
|
|
if (!doc) {
|
|
return state;
|
|
}
|
|
|
|
switch (action.type) {
|
|
|
|
case ENTITY_UPDATE:
|
|
case ENTITY_INSERT:
|
|
return {...state, [doc._id]: doc};
|
|
|
|
case ENTITY_REMOVE:
|
|
const newState = Object.assign({}, state);
|
|
delete newState[action[referenceName]._id];
|
|
return newState;
|
|
|
|
default:
|
|
return state;
|
|
}
|
|
}
|
|
}
|
|
|
|
const reducers = {};
|
|
for (const type of ALL_TYPES) {
|
|
// Name example: RequestGroup => requestGroups
|
|
// Add an "s" to the end if there isn't already
|
|
const trailer = type.match(/s$/) ? '' : 's';
|
|
const name = `${type.slice(0, 1).toLowerCase()}${type.slice(1)}${trailer}`;
|
|
reducers[name] = genericEntityReducer(type);
|
|
}
|
|
|
|
export default combineReducers({
|
|
...reducers,
|
|
doNotPersist: () => true
|
|
});
|
|
|
|
|
|
// ~~~~~~~ //
|
|
// ACTIONS //
|
|
// ~~~~~~~ //
|
|
|
|
const insertFns = {};
|
|
for (let type of ALL_TYPES) {
|
|
insertFns[type] = doc => ({type: ENTITY_INSERT, [type]: doc})
|
|
}
|
|
|
|
const updateFns = {};
|
|
for (let type of ALL_TYPES) {
|
|
updateFns[type] = doc => ({type: ENTITY_UPDATE, [type]: doc})
|
|
}
|
|
|
|
const removeFns = {};
|
|
for (let type of ALL_TYPES) {
|
|
removeFns[type] = doc => ({type: ENTITY_REMOVE, [type]: doc})
|
|
}
|
|
|
|
export function insert (doc) {
|
|
return insertFns[doc.type](doc);
|
|
}
|
|
|
|
export function update (doc) {
|
|
return updateFns[doc.type](doc);
|
|
}
|
|
|
|
export function remove (doc) {
|
|
return removeFns[doc.type](doc);
|
|
}
|