mirror of
https://github.com/Kong/insomnia
synced 2024-11-08 14:49:53 +00:00
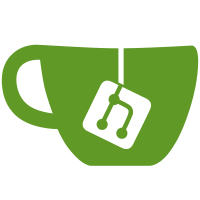
* Fixed duplication kve bug * Some minor css * Fix modal refs * Got variable modal showing * A bit more * Errors and debounced render * Finished naive editors * Some key value editor fixes * Yet even more one-line fixes * Added some transitions * Small fixes * Added key flattening util
50 lines
1020 B
JavaScript
50 lines
1020 B
JavaScript
import React, {PureComponent, PropTypes} from 'react';
|
|
import autobind from 'autobind-decorator';
|
|
|
|
@autobind
|
|
class Button extends PureComponent {
|
|
_handleClick (e) {
|
|
const {onClick, onDisabledClick, disabled} = this.props;
|
|
const fn = disabled ? onDisabledClick : onClick;
|
|
|
|
if (this.props.hasOwnProperty('value')) {
|
|
fn && fn(this.props.value, e);
|
|
} else {
|
|
fn && fn(e);
|
|
}
|
|
}
|
|
|
|
render () {
|
|
const {
|
|
children,
|
|
disabled,
|
|
tabIndex,
|
|
className
|
|
} = this.props;
|
|
|
|
return (
|
|
<button disabled={disabled}
|
|
tabIndex={tabIndex}
|
|
className={className}
|
|
onClick={this._handleClick}>
|
|
{children}
|
|
</button>
|
|
);
|
|
}
|
|
}
|
|
|
|
Button.propTypes = {
|
|
// Required
|
|
children: PropTypes.node.isRequired,
|
|
|
|
// Optional
|
|
value: PropTypes.any,
|
|
className: PropTypes.string,
|
|
onDisabledClick: PropTypes.func,
|
|
onClick: PropTypes.func,
|
|
disabled: PropTypes.bool,
|
|
tabIndex: PropTypes.number
|
|
};
|
|
|
|
export default Button;
|