mirror of
https://github.com/Kong/insomnia
synced 2024-11-08 23:00:30 +00:00
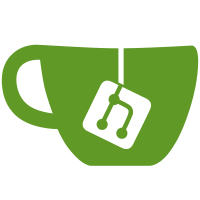
* New data models for hotkeys and store the key bindings in settings * Merge win and linux key bindings, remove generic key combinations, and add documents
39 lines
985 B
JavaScript
39 lines
985 B
JavaScript
// @flow
|
|
import * as React from 'react';
|
|
import classnames from 'classnames';
|
|
import { ALT_SYM, CTRL_SYM, isMac, joinHotKeys, META_SYM, SHIFT_SYM } from '../../common/constants';
|
|
import type { KeyBindings } from '../../common/hotkeys';
|
|
import * as hotkeys from '../../common/hotkeys';
|
|
|
|
type Props = {
|
|
keyBindings: KeyBindings,
|
|
|
|
// Optional
|
|
className?: string,
|
|
};
|
|
|
|
class Hotkey extends React.PureComponent<Props> {
|
|
render() {
|
|
const { keyBindings, className } = this.props;
|
|
|
|
const keyComb = hotkeys.getPlatformKeyCombinations(keyBindings)[0];
|
|
const { ctrl, alt, shift, meta, keyCode } = keyComb;
|
|
const chars = [];
|
|
|
|
alt && chars.push(ALT_SYM);
|
|
shift && chars.push(SHIFT_SYM);
|
|
ctrl && chars.push(CTRL_SYM);
|
|
meta && chars.push(META_SYM);
|
|
|
|
chars.push(hotkeys.getChar(keyCode));
|
|
|
|
return (
|
|
<span className={classnames(className, { 'font-normal': isMac() })}>
|
|
{joinHotKeys(chars)}
|
|
</span>
|
|
);
|
|
}
|
|
}
|
|
|
|
export default Hotkey;
|