mirror of
https://github.com/Kong/insomnia
synced 2024-11-08 14:49:53 +00:00
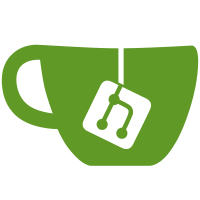
* Move basic auth routes to a separate file * Stop slowing requests down artificially * Add initial oauth routes * Mount oidc routes under /oidc * Enable all forms of oauth that Insomnia supports * Add oauth request collection fixture * Update playwright config * Use 127.0.0.1 instead of localhost * simple oauth2 test * Make the playwright extension work * Move oauth tests to a separate file * Test all oauth flows * Mark test as slow * Wait for load state for new pages * Use locators consistently * Add playwright to recommended extensions * Add instructions for how to use the playwright extension * update selectors and use fill * Fix markdown lint Co-authored-by: jackkav <jackkav@gmail.com> Co-authored-by: gatzjames <jamesgatzos@gmail.com>
56 lines
2.3 KiB
TypeScript
56 lines
2.3 KiB
TypeScript
import fs from 'fs';
|
|
import os from 'os';
|
|
import path from 'path';
|
|
import { exit } from 'process';
|
|
import * as uuid from 'uuid';
|
|
|
|
// Default to dev so that the playwright vscode extension works
|
|
export const bundleType = () => process.env.BUNDLE || 'dev';
|
|
|
|
export const loadFixture = async (fixturePath: string) => {
|
|
const buffer = await fs.promises.readFile(path.join(__dirname, '..', 'fixtures', fixturePath));
|
|
return buffer.toString('utf-8');
|
|
};
|
|
|
|
export const randomDataPath = () => path.join(os.tmpdir(), 'insomnia-smoke-test', `${uuid.v4()}`);
|
|
export const INSOMNIA_DATA_PATH = randomDataPath();
|
|
|
|
const pathLookup = {
|
|
win32: path.join('win-unpacked', 'Insomnia.exe'),
|
|
darwin: path.join('mac', 'Insomnia.app', 'Contents', 'MacOS', 'Insomnia'),
|
|
linux: path.join('linux-unpacked', 'insomnia'),
|
|
};
|
|
const insomniaBinary = path.join('dist', pathLookup[process.platform]);
|
|
const electronBinary = path.join('node_modules', '.bin', process.platform === 'win32' ? 'electron.cmd' : 'electron');
|
|
|
|
export const executablePath = bundleType() === 'package' ? insomniaBinary : electronBinary;
|
|
|
|
// NOTE: main.min.js is built by app-build:smoke in /build and also by the watcher in /app
|
|
export const mainPath = path.join(bundleType() === 'dev' ? 'app' : 'build', 'main.min.js');
|
|
export const cwd = path.resolve(__dirname, '..', '..', 'insomnia-app');
|
|
|
|
const hasMainBeenBuilt = fs.existsSync(path.resolve(cwd, mainPath));
|
|
const hasBinaryBeenBuilt = fs.existsSync(path.resolve(cwd, insomniaBinary));
|
|
|
|
// NOTE: guard against missing build artifacts
|
|
if (bundleType() === 'dev' && !hasMainBeenBuilt) {
|
|
console.error(`ERROR: ${mainPath} not found at ${path.resolve(cwd, mainPath)}
|
|
Have you run "npm run watch:app"?`);
|
|
exit(1);
|
|
}
|
|
if (bundleType() === 'build' && !hasMainBeenBuilt) {
|
|
console.error(`ERROR: ${mainPath} not found at ${path.resolve(cwd, mainPath)}
|
|
Have you run "npm run app-build:smoke"?`);
|
|
exit(1);
|
|
}
|
|
if (bundleType() === 'package' && !hasBinaryBeenBuilt) {
|
|
console.error(`ERROR: ${insomniaBinary} not found at ${path.resolve(cwd, insomniaBinary)}
|
|
Have you run "npm run app-package:smoke"?`);
|
|
exit(1);
|
|
}
|
|
if (process.env.DEBUG) {
|
|
console.log(`Using current working directory at ${cwd}`);
|
|
console.log(`Using executablePath at ${executablePath}`);
|
|
console.log(`Using mainPath at ${mainPath}`);
|
|
}
|