mirror of
https://github.com/Kong/insomnia
synced 2024-11-08 14:49:53 +00:00
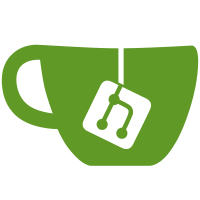
* VCS proof of concept underway! * Stuff * Some things * Replace deprecated Electron makeSingleInstance * Rename `window` variables so not to be confused with window object * Don't unnecessarily update request when URL does not change * Regenerate package-lock * Fix tests + ESLint * Publish - insomnia-app@1.0.49 - insomnia-cookies@0.0.12 - insomnia-httpsnippet@1.16.18 - insomnia-importers@2.0.13 - insomnia-libcurl@0.0.23 - insomnia-prettify@0.1.7 - insomnia-url@0.1.6 - insomnia-xpath@1.0.9 - insomnia-plugin-base64@1.0.6 - insomnia-plugin-cookie-jar@1.0.8 - insomnia-plugin-core-themes@1.0.5 - insomnia-plugin-default-headers@1.1.9 - insomnia-plugin-file@1.0.7 - insomnia-plugin-hash@1.0.7 - insomnia-plugin-jsonpath@1.0.12 - insomnia-plugin-now@1.0.11 - insomnia-plugin-os@1.0.13 - insomnia-plugin-prompt@1.1.9 - insomnia-plugin-request@1.0.18 - insomnia-plugin-response@1.0.16 - insomnia-plugin-uuid@1.0.10 * Broken but w/e * Some tweaks * Big refactor. Create local snapshots and push done * POC merging and a lot of improvements * Lots of work done on initial UI/UX * Fix old tests * Atomic writes and size-based batches * Update StageEntry definition once again to be better * Factor out GraphQL query logic * Merge algorithm, history modal, other minor things * Fix test * Merge, checkout, revert w/ user changes now work * Force UI to refresh when switching branches changes active request * Rough draft pull() and some cleanup * E2EE stuff and some refactoring * Add ability to share project with team and fixed tests * VCS now created in root component and better remote project handling * Remove unused definition * Publish - insomnia-account@0.0.2 - insomnia-app@1.1.1 - insomnia-cookies@0.0.14 - insomnia-httpsnippet@1.16.20 - insomnia-importers@2.0.15 - insomnia-libcurl@0.0.25 - insomnia-prettify@0.1.9 - insomnia-sync@0.0.2 - insomnia-url@0.1.8 - insomnia-xpath@1.0.11 - insomnia-plugin-base64@1.0.8 - insomnia-plugin-cookie-jar@1.0.10 - insomnia-plugin-core-themes@1.0.7 - insomnia-plugin-file@1.0.9 - insomnia-plugin-hash@1.0.9 - insomnia-plugin-jsonpath@1.0.14 - insomnia-plugin-now@1.0.13 - insomnia-plugin-os@1.0.15 - insomnia-plugin-prompt@1.1.11 - insomnia-plugin-request@1.0.20 - insomnia-plugin-response@1.0.18 - insomnia-plugin-uuid@1.0.12 * Move some deps around * Fix Flow errors * Update package.json * Fix eslint errors * Fix tests * Update deps * bootstrap insomnia-sync * TRy fixing appveyor * Try something else * Bump lerna * try powershell * Try again * Fix imports * Fixed errors * sync types refactor * Show remote projects in workspace dropdown * Improved pulling of non-local workspaces * Loading indicators and some tweaks * Clean up sync staging modal * Some sync improvements: - No longer store stage - Upgrade Electron - Sync UI/UX improvements * Fix snyc tests * Upgraded deps and hot loader tweaks (it's broken for some reason) * Fix tests * Branches dialog, network refactoring, some tweaks * Fixed merging when other branch is empty * A bunch of small fixes from real testing * Fixed pull merge logic * Fix tests * Some bug fixes * A few small tweaks * Conflict resolution and other improvements * Fix tests * Add revert changes * Deal with duplicate projects per workspace * Some tweaks and accessibility improvements * Tooltip accessibility * Fix API endpoint * Fix tests * Remove jest dep from insomnia-importers
118 lines
4.0 KiB
JavaScript
118 lines
4.0 KiB
JavaScript
// @flow
|
|
import React from 'react';
|
|
import uuid from 'uuid';
|
|
import * as toughCookie from 'tough-cookie';
|
|
import autobind from 'autobind-decorator';
|
|
import { cookieToString } from 'insomnia-cookies';
|
|
import PromptButton from './base/prompt-button';
|
|
import RenderedText from './rendered-text';
|
|
import type { Cookie } from '../../models/cookie-jar';
|
|
import { Dropdown, DropdownButton, DropdownItem } from './base/dropdown/index';
|
|
|
|
type Props = {
|
|
handleCookieAdd: Function,
|
|
handleCookieDelete: Function,
|
|
handleDeleteAll: Function,
|
|
cookies: Array<Cookie>,
|
|
newCookieDomainName: string,
|
|
handleShowModifyCookieModal: Function,
|
|
handleRender: Function,
|
|
};
|
|
|
|
@autobind
|
|
class CookieList extends React.PureComponent<Props> {
|
|
_handleCookieAdd() {
|
|
const newCookie: Cookie = {
|
|
id: uuid.v4(),
|
|
key: 'foo',
|
|
value: 'bar',
|
|
domain: this.props.newCookieDomainName,
|
|
expires: null,
|
|
path: '/',
|
|
secure: false,
|
|
httpOnly: false,
|
|
};
|
|
|
|
this.props.handleCookieAdd(newCookie);
|
|
}
|
|
|
|
_handleDeleteCookie(cookie: Cookie) {
|
|
this.props.handleCookieDelete(cookie);
|
|
}
|
|
|
|
render() {
|
|
const { cookies, handleDeleteAll, handleShowModifyCookieModal, handleRender } = this.props;
|
|
|
|
return (
|
|
<div>
|
|
<table className="table--fancy cookie-table table--striped">
|
|
<thead>
|
|
<tr>
|
|
<th style={{ minWidth: '10rem' }}>Domain</th>
|
|
<th style={{ width: '90%' }}>Cookie</th>
|
|
<th style={{ width: '2rem' }} className="text-right">
|
|
<Dropdown right>
|
|
<DropdownButton
|
|
title="Add cookie"
|
|
className="btn btn--super-duper-compact btn--outlined txt-md">
|
|
Actions <i className="fa fa-caret-down" />
|
|
</DropdownButton>
|
|
<DropdownItem onClick={this._handleCookieAdd}>
|
|
<i className="fa fa-plus-circle" /> Add Cookie
|
|
</DropdownItem>
|
|
<DropdownItem onClick={handleDeleteAll} buttonClass={PromptButton}>
|
|
<i className="fa fa-trash-o" /> Delete All
|
|
</DropdownItem>
|
|
</Dropdown>
|
|
</th>
|
|
</tr>
|
|
</thead>
|
|
<tbody key={cookies.length}>
|
|
{cookies.map((cookie, i) => {
|
|
const cookieString = cookieToString(toughCookie.Cookie.fromJSON(cookie));
|
|
return (
|
|
<tr className="selectable" key={i}>
|
|
<td>
|
|
<RenderedText render={handleRender}>{cookie.domain || ''}</RenderedText>
|
|
</td>
|
|
<td className="force-wrap wide">
|
|
<RenderedText render={handleRender}>{cookieString || ''}</RenderedText>
|
|
</td>
|
|
<td onClick={null} className="text-right no-wrap">
|
|
<button
|
|
className="btn btn--super-compact btn--outlined"
|
|
onClick={e => handleShowModifyCookieModal(cookie)}
|
|
title="Edit cookie properties">
|
|
Edit
|
|
</button>{' '}
|
|
<PromptButton
|
|
className="btn btn--super-compact btn--outlined"
|
|
addIcon
|
|
confirmMessage=""
|
|
onClick={e => this._handleDeleteCookie(cookie)}
|
|
title="Delete cookie">
|
|
<i className="fa fa-trash-o" />
|
|
</PromptButton>
|
|
</td>
|
|
</tr>
|
|
);
|
|
})}
|
|
</tbody>
|
|
</table>
|
|
{cookies.length === 0 && (
|
|
<div className="pad faint italic text-center">
|
|
<p>I couldn't find any cookies for you.</p>
|
|
<p>
|
|
<button className="btn btn--clicky" onClick={e => this._handleCookieAdd()}>
|
|
Add Cookie <i className="fa fa-plus-circle" />
|
|
</button>
|
|
</p>
|
|
</div>
|
|
)}
|
|
</div>
|
|
);
|
|
}
|
|
}
|
|
|
|
export default CookieList;
|