mirror of
https://github.com/Kong/insomnia
synced 2024-11-08 06:39:48 +00:00
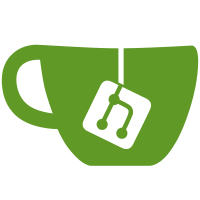
* Upgrade electron-builder * Fix optional dep * Add another dep * Fix travis * Fix 7zip install * Fix icons * Minor fix * Delete changelog * Moved build scripts to JS * Some adjustments * Remove lines * Add 7zip back * Update scripts * Small tweaks * Fix electronbuilder config * snap dependency * Fix pkg * Remove snapd dep * Updated deps and lots of fixes * Travis update * Return package name * Remove snap for now * Remove portable * Try fixing code signing * Bump cache * Disable automatic tests * Fix windows artifacts * package-lock for app/ * Try fix npm install * Try again * Some minor tweaks * Preleases by default
85 lines
1.7 KiB
JavaScript
85 lines
1.7 KiB
JavaScript
// @flow
|
|
import * as db from '../common/database';
|
|
import type {BaseModel} from './index';
|
|
|
|
export const name = 'Cookie Jar';
|
|
export const type = 'CookieJar';
|
|
export const prefix = 'jar';
|
|
export const canDuplicate = true;
|
|
|
|
export type Cookie = {
|
|
id: string,
|
|
key: string,
|
|
value: string,
|
|
expires: Date | string | number | null,
|
|
domain: string,
|
|
path: string,
|
|
secure: boolean,
|
|
httpOnly: boolean,
|
|
|
|
extensions?: Array<any>,
|
|
creation?: Date,
|
|
creationIndex?: number,
|
|
hostOnly?: boolean,
|
|
pathIsDefault?: boolean,
|
|
lastAccessed?: Date
|
|
}
|
|
|
|
type BaseCookieJar = {
|
|
name: string,
|
|
cookies: Array<Cookie>
|
|
};
|
|
|
|
export type CookieJar = BaseModel & BaseCookieJar;
|
|
|
|
export function init () {
|
|
return {
|
|
name: 'Default Jar',
|
|
cookies: []
|
|
};
|
|
}
|
|
|
|
export function migrate (doc: CookieJar): CookieJar {
|
|
doc = migrateCookieId(doc);
|
|
return doc;
|
|
}
|
|
|
|
export function create (patch: Object = {}) {
|
|
if (!patch.parentId) {
|
|
throw new Error(`New CookieJar missing \`parentId\`: ${JSON.stringify(patch)}`);
|
|
}
|
|
|
|
return db.docCreate(type, patch);
|
|
}
|
|
|
|
export async function getOrCreateForParentId (parentId: string) {
|
|
const cookieJars = await db.find(type, {parentId});
|
|
if (cookieJars.length === 0) {
|
|
return create({parentId});
|
|
} else {
|
|
return cookieJars[0];
|
|
}
|
|
}
|
|
|
|
export function all () {
|
|
return db.all(type);
|
|
}
|
|
|
|
export function getById (id: string) {
|
|
return db.get(type, id);
|
|
}
|
|
|
|
export function update (cookieJar: CookieJar, patch: Object = {}) {
|
|
return db.docUpdate(cookieJar, patch);
|
|
}
|
|
|
|
/** Ensure every cookie has an ID property */
|
|
function migrateCookieId (cookieJar: CookieJar) {
|
|
for (const cookie of cookieJar.cookies) {
|
|
if (!cookie.id) {
|
|
cookie.id = Math.random().toString().replace('0.', '');
|
|
}
|
|
}
|
|
return cookieJar;
|
|
}
|