mirror of
https://github.com/Kong/insomnia
synced 2024-11-08 06:39:48 +00:00
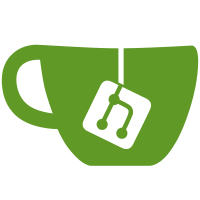
* ⚡️ vite
* replace webpack with esbuild in build script
* move build sr to esbuild
* esbuild send-request shim
* remove main externals
* fix lint
* remove webpack from insomnia-testing
* removes more webpack stuff
TODO after this PR: make debugging work again
* pin swagger-ui-react to version before esm change
* restore prepare script to build in bootstrap
* use default tsconfig for eslint and apply fixes
* bundle insomnia-components as cjs/esm
* makes ca_certs.ts pass linting
* builds types for insomnia-components
* improve build script for production
* skip typechecking insomnia-components
* separate package from build
* add electron to externals
* add preload bundling and fix build output
* exclude grpc/proto-loader from the bundle
* move node packages to commonjs
* don't bundle grpc since it's a node module
* fix content security error
* use vite lib mode for insomnia-components
* tidy up vite config and tsconfig options
* update package-locks
* use process.env. for static build time variables
* fix vscode debugging
Co-authored-by: jackkav <jackkav@gmail.com>
Co-authored-by: Dimitri Mitropoulos <dimitrimitropoulos@gmail.com>
68 lines
1.9 KiB
TypeScript
68 lines
1.9 KiB
TypeScript
import esbuild from 'esbuild';
|
|
import { builtinModules } from 'module';
|
|
import path from 'path';
|
|
|
|
import pkg from './package.json';
|
|
|
|
interface Options {
|
|
mode?: 'development' | 'production';
|
|
}
|
|
|
|
export default async function build(options: Options) {
|
|
const mode = options.mode || 'production';
|
|
// The list of packages that will be included in the node_modules folder of the packaged app.
|
|
// Exclude all package.json dependencies except from the ones in the packedDependencies list
|
|
const unpackedDependencies = [
|
|
...Object.keys(pkg.dependencies).filter(
|
|
name => !pkg.packedDependencies.includes(name)
|
|
),
|
|
];
|
|
|
|
const __DEV__ = mode !== 'production';
|
|
const PORT = pkg.dev['dev-server-port'];
|
|
|
|
const outdir = __DEV__
|
|
? path.join(__dirname, 'app')
|
|
: path.join(__dirname, 'build');
|
|
|
|
const env: Record<string, string> = __DEV__
|
|
? {
|
|
'process.env.APP_RENDER_URL': JSON.stringify(
|
|
`http://localhost:${PORT}/index.html`
|
|
),
|
|
'process.env.NODE_ENV': JSON.stringify('development'),
|
|
'process.env.INSOMNIA_ENV': JSON.stringify('development'),
|
|
'process.env.BUILD_DATE': JSON.stringify(new Date()),
|
|
}
|
|
: {
|
|
'process.env.NODE_ENV': JSON.stringify('production'),
|
|
'process.env.INSOMNIA_ENV': JSON.stringify('production'),
|
|
'process.env.BUILD_DATE': JSON.stringify(new Date()),
|
|
};
|
|
|
|
return esbuild.build({
|
|
entryPoints: ['./app/main.development.ts'],
|
|
outfile: path.join(outdir, 'main.min.js'),
|
|
bundle: true,
|
|
platform: 'node',
|
|
sourcemap: true,
|
|
format: 'cjs',
|
|
define: env,
|
|
external: [
|
|
'electron',
|
|
'@getinsomnia/node-libcurl',
|
|
...Object.keys(unpackedDependencies),
|
|
...Object.keys(builtinModules),
|
|
],
|
|
});
|
|
}
|
|
|
|
// Build if ran as a cli script
|
|
const isMain = require.main === module;
|
|
|
|
if (isMain) {
|
|
const mode =
|
|
process.env.NODE_ENV === 'development' ? 'development' : 'production';
|
|
build({ mode });
|
|
}
|