mirror of
https://github.com/Kong/insomnia
synced 2024-11-08 23:00:30 +00:00
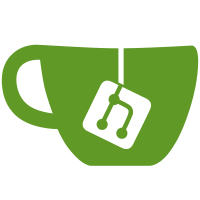
* Most things are working * Cookies and lots of improvements * Hoist regex * Appveyor test * Fix appveyor file * Remove yarn * Test deps * Fix * Remove all git deps * fix * Fix * Add cash rm * Fix command * Fix * Production * try * fix * fix? * Done * Remove cache * tweak * Shortcut * Fix * Attempt * try * Try clone depth * Try again * back to shallow_clone * Some debugging * Another try * Try something else * Another * try curl only * Another * fix * Update node-gyp * Try again * Try * Again * Some pruning * Back to the start * Again * try config * Reorder test * Try without cache * Update dep * Try again * remove shallow_clone * don't run tests * Implement CA fetching * Fix * Use CAs * Fix? * test * Remove build dir * Fix dir path? * Try again * Aagain * Try * Change dep * Add comment * Invalidate cache * Remove yarn * Fix cookie expiry * fixed tests * Some modal fixes and unrelated stuff * Debounce autocomplete * Got client certificates working * Fixed binary files * Fixed cookies * Got proxy and debug info working * Got CA certs working * Update appveyor build * Fixed cookie date parsing and overlay z-index * Test updates * Autocomplete to bulk header editor * Small CSS tweak
103 lines
2.4 KiB
JavaScript
103 lines
2.4 KiB
JavaScript
import React, {PureComponent, PropTypes} from 'react';
|
|
import autobind from 'autobind-decorator';
|
|
import VariableEditor from '../templating/variable-editor';
|
|
import TagEditor from '../templating/tag-editor';
|
|
import Modal from '../base/modal';
|
|
import ModalBody from '../base/modal-body';
|
|
import ModalHeader from '../base/modal-header';
|
|
import ModalFooter from '../base/modal-footer';
|
|
import {trackEvent} from '../../../analytics';
|
|
|
|
@autobind
|
|
class NunjucksModal extends PureComponent {
|
|
constructor (props) {
|
|
super(props);
|
|
this.state = {
|
|
defaultTemplate: ''
|
|
};
|
|
|
|
this._onDone = null;
|
|
this._currentTemplate = null;
|
|
}
|
|
|
|
_setModalRef (n) {
|
|
this.modal = n;
|
|
}
|
|
|
|
_handleTemplateChange (template) {
|
|
this._currentTemplate = template;
|
|
}
|
|
|
|
show ({template, onDone}) {
|
|
this._onDone = onDone;
|
|
|
|
this.setState({
|
|
defaultTemplate: template
|
|
});
|
|
|
|
trackEvent('Nunjucks', 'Editor', 'Show');
|
|
|
|
// NOTE: This must be called after setState() above because show() is going
|
|
// to force refresh the modal
|
|
this.modal.show();
|
|
}
|
|
|
|
hide () {
|
|
this.modal.hide();
|
|
trackEvent('Nunjucks', 'Editor', 'Hide');
|
|
}
|
|
|
|
_handleSubmit (e) {
|
|
e.preventDefault();
|
|
this._onDone && this._onDone(this._currentTemplate);
|
|
this.hide();
|
|
}
|
|
|
|
render () {
|
|
const {handleRender} = this.props;
|
|
const {defaultTemplate} = this.state;
|
|
|
|
let editor = null;
|
|
let title = '';
|
|
if (defaultTemplate.indexOf('{{') === 0) {
|
|
title = 'Variable Reference';
|
|
editor = (
|
|
<VariableEditor
|
|
onChange={this._handleTemplateChange}
|
|
defaultValue={defaultTemplate}
|
|
handleRender={handleRender}
|
|
/>
|
|
);
|
|
} else if (defaultTemplate.indexOf('{%') === 0) {
|
|
title = 'Tag';
|
|
editor = (
|
|
<TagEditor
|
|
onChange={this._handleTemplateChange}
|
|
defaultValue={defaultTemplate}
|
|
handleRender={handleRender}
|
|
/>
|
|
);
|
|
}
|
|
|
|
return (
|
|
<Modal ref={this._setModalRef} freshState>
|
|
<form onSubmit={this._handleSubmit}>
|
|
<ModalHeader>Edit {title}</ModalHeader>
|
|
<ModalBody className="pad">
|
|
{editor}
|
|
</ModalBody>
|
|
<ModalFooter>
|
|
<button className="btn">Done</button>
|
|
</ModalFooter>
|
|
</form>
|
|
</Modal>
|
|
);
|
|
}
|
|
}
|
|
|
|
NunjucksModal.propTypes = {
|
|
handleRender: PropTypes.func.isRequired
|
|
};
|
|
|
|
export default NunjucksModal;
|