mirror of
https://github.com/Kong/insomnia
synced 2024-11-08 14:49:53 +00:00
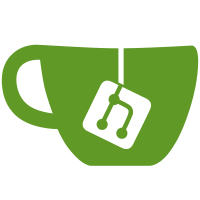
* Slightly smarter preview mode selection * Tweak autocomplete stuff a bit * Switched variable editor to <select> * Reduced lag on nunjucks tags
65 lines
1.3 KiB
JavaScript
65 lines
1.3 KiB
JavaScript
import React, {PureComponent, PropTypes} from 'react';
|
|
import autobind from 'autobind-decorator';
|
|
|
|
@autobind
|
|
class ResponseRaw extends PureComponent {
|
|
constructor (props) {
|
|
super(props);
|
|
this._timeout = null;
|
|
}
|
|
|
|
_setTextAreaRef (n) {
|
|
this._textarea = n;
|
|
}
|
|
|
|
_update (value) {
|
|
clearTimeout(this._timeout);
|
|
this._timeout = setTimeout(() => {
|
|
if (this._textarea) {
|
|
this._textarea.value = value;
|
|
}
|
|
}, 200);
|
|
}
|
|
|
|
componentDidUpdate () {
|
|
this._update(this.props.value);
|
|
}
|
|
|
|
componentDidMount () {
|
|
this._update(this.props.value);
|
|
}
|
|
|
|
shouldComponentUpdate (nextProps) {
|
|
for (let key in nextProps) {
|
|
if (nextProps.hasOwnProperty(key)) {
|
|
if (nextProps[key] !== this.props[key]) {
|
|
return true;
|
|
}
|
|
}
|
|
}
|
|
|
|
return false;
|
|
}
|
|
|
|
render () {
|
|
const {fontSize} = this.props;
|
|
return (
|
|
<textarea
|
|
ref={this._setTextAreaRef}
|
|
placeholder="..."
|
|
className="force-wrap scrollable wide tall selectable monospace pad no-resize"
|
|
readOnly
|
|
defaultValue=""
|
|
style={{fontSize}}>
|
|
</textarea>
|
|
);
|
|
}
|
|
}
|
|
|
|
ResponseRaw.propTypes = {
|
|
value: PropTypes.string.isRequired,
|
|
fontSize: PropTypes.number
|
|
};
|
|
|
|
export default ResponseRaw;
|