mirror of
https://github.com/Kong/insomnia
synced 2024-11-08 14:49:53 +00:00
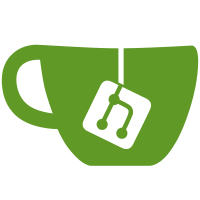
* Maybe working POC * Change to use remote url * Other URL too * Some logic * Got the push part working * Made some updates * Fix * Update * Add status code check * Stuff * Implemented new sync api * A bit more robust * Debounce changes * Change timeout * Some fixes * Remove .less * Better error handling * Fix base url * Support for created vs updated docs * Try silent * Silence removal too * Small fix after merge * Fix test * Stuff * Implement key generation algorithm * Tidy * stuff * A bunch of stuff for the new API * Integrated the session stuff * Stuff * Just started on encryption * Lots of updates to encryption * Finished createResourceGroup function * Full encryption/decryption working (I think) * Encrypt localstorage with sessionID * Some more * Some extra checks * Now uses separate DB. Still needs to be simplified a LOT * Fix deletion bug * Fixed unicode bug with encryption * Simplified and working * A bunch of polish * Some stuff * Removed some workspace meta properties * Migrated a few more meta properties * Small changes * Fix body scrolling and url cursor jumping * Removed duplication of webpack port * Remove workspaces reduces * Some small fixes * Added sync modal and opt-in setting * Good start to sync flow * Refactored modal footer css * Update sync status * Sync logger * A bit better logging * Fixed a bunch of sync-related bugs * Fixed signup form button * Gravatar component * Split sync modal into tabs * Tidying * Some more error handling * start sending 'user agent * Login/signup error handling * Use real UUIDs * Fixed tests * Remove unused function * Some extra checks * Moved cloud sync setting to about page * Some small changes * Some things
146 lines
4.3 KiB
JavaScript
146 lines
4.3 KiB
JavaScript
import React, {Component} from 'react';
|
|
import HTTPSnippet, {availableTargets} from 'httpsnippet';
|
|
|
|
import CopyButton from '../base/CopyButton';
|
|
import Dropdown from '../base/Dropdown';
|
|
import Editor from '../base/Editor';
|
|
import Modal from '../base/Modal';
|
|
import ModalBody from '../base/ModalBody';
|
|
import ModalHeader from '../base/ModalHeader';
|
|
import ModalFooter from '../base/ModalFooter';
|
|
import {exportHar} from '../../../backend/export/har';
|
|
|
|
const DEFAULT_TARGET = availableTargets().find(t => t.key === 'shell');
|
|
const DEFAULT_CLIENT = DEFAULT_TARGET.clients.find(t => t.key === 'curl');
|
|
const MODE_MAP = {
|
|
c: 'clike',
|
|
java: 'clike',
|
|
csharp: 'clike',
|
|
node: 'javascript',
|
|
objc: 'clike',
|
|
ocaml: 'mllike'
|
|
};
|
|
|
|
const TO_ADD_CONTENT_LENGTH = {
|
|
node: ['native']
|
|
};
|
|
|
|
|
|
class GenerateCodeModal extends Component {
|
|
constructor (props) {
|
|
super(props);
|
|
this.state = {
|
|
cmd: '',
|
|
request: null,
|
|
target: DEFAULT_TARGET,
|
|
client: DEFAULT_CLIENT
|
|
};
|
|
}
|
|
|
|
_handleClientChange (client) {
|
|
const {target, request} = this.state;
|
|
this._generateCode(request, target, client);
|
|
}
|
|
|
|
_handleTargetChange (target) {
|
|
const {target: currentTarget} = this.state;
|
|
if (currentTarget.key === target.key) {
|
|
// No change
|
|
return;
|
|
}
|
|
|
|
const client = target.clients.find(c => c.key === target.default);
|
|
this._generateCode(this.state.request, target, client);
|
|
}
|
|
|
|
async _generateCode (request, target, client) {
|
|
// Some clients need a content-length for the request to succeed
|
|
const addContentLength = (TO_ADD_CONTENT_LENGTH[target.key] || []).find(c => c === client.key);
|
|
|
|
const har = await exportHar(request._id, addContentLength);
|
|
const snippet = new HTTPSnippet(har);
|
|
const cmd = snippet.convert(target.key, client.key);
|
|
|
|
this.setState({request, cmd, client, target});
|
|
}
|
|
|
|
show (request) {
|
|
this.modal.show();
|
|
this._generateCode(request, DEFAULT_TARGET, DEFAULT_CLIENT);
|
|
}
|
|
|
|
render () {
|
|
const {cmd, target, client} = this.state;
|
|
const targets = availableTargets();
|
|
|
|
return (
|
|
<Modal ref={m => this.modal = m} tall={true} {...this.props}>
|
|
<ModalHeader>Generate Client Code</ModalHeader>
|
|
<ModalBody noScroll={true} style={{
|
|
display: 'grid',
|
|
gridTemplateColumns: 'minmax(0, 1fr)',
|
|
gridTemplateRows: 'auto minmax(0, 1fr)'
|
|
}}>
|
|
<div className="pad">
|
|
<Dropdown outline={true}>
|
|
<button className="btn btn--super-compact btn--outlined">
|
|
{target.title}
|
|
<i className="fa fa-caret-down"></i>
|
|
</button>
|
|
<ul>
|
|
{targets.map(target => (
|
|
<li key={target.key}>
|
|
<button onClick={() => this._handleTargetChange(target)}>
|
|
{target.title}
|
|
</button>
|
|
</li>
|
|
))}
|
|
</ul>
|
|
</Dropdown>
|
|
|
|
<Dropdown outline={true}>
|
|
<button className="btn btn--super-compact btn--outlined">
|
|
{client.title}
|
|
<i className="fa fa-caret-down"></i>
|
|
</button>
|
|
<ul>
|
|
{target.clients.map(client => (
|
|
<li key={client.key}>
|
|
<button onClick={() => this._handleClientChange(client)}>
|
|
{client.title}
|
|
</button>
|
|
</li>
|
|
))}
|
|
</ul>
|
|
</Dropdown>
|
|
|
|
<CopyButton content={cmd}
|
|
className="pull-right btn btn--super-compact btn--outlined"/>
|
|
</div>
|
|
<Editor
|
|
className="border-top"
|
|
key={Date.now()}
|
|
mode={MODE_MAP[target.key] || target.key}
|
|
ref={n => this._editor = n}
|
|
lightTheme={true}
|
|
lineWrapping={true}
|
|
value={cmd}
|
|
/>
|
|
</ModalBody>
|
|
<ModalFooter>
|
|
<div className="margin-left faint italic txt-sm tall">
|
|
* copy/paste this command into a Unix terminal
|
|
</div>
|
|
<button className="btn" onClick={e => this.modal.hide()}>
|
|
Done
|
|
</button>
|
|
</ModalFooter>
|
|
</Modal>
|
|
);
|
|
}
|
|
}
|
|
|
|
GenerateCodeModal.propTypes = {};
|
|
|
|
export default GenerateCodeModal;
|