mirror of
https://github.com/Kong/insomnia
synced 2024-11-08 14:49:53 +00:00
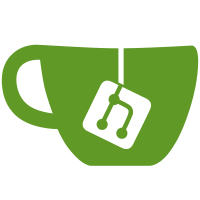
* Maybe working POC * Change to use remote url * Other URL too * Some logic * Got the push part working * Made some updates * Fix * Update * Add status code check * Stuff * Implemented new sync api * A bit more robust * Debounce changes * Change timeout * Some fixes * Remove .less * Better error handling * Fix base url * Support for created vs updated docs * Try silent * Silence removal too * Small fix after merge * Fix test * Stuff * Implement key generation algorithm * Tidy * stuff * A bunch of stuff for the new API * Integrated the session stuff * Stuff * Just started on encryption * Lots of updates to encryption * Finished createResourceGroup function * Full encryption/decryption working (I think) * Encrypt localstorage with sessionID * Some more * Some extra checks * Now uses separate DB. Still needs to be simplified a LOT * Fix deletion bug * Fixed unicode bug with encryption * Simplified and working * A bunch of polish * Some stuff * Removed some workspace meta properties * Migrated a few more meta properties * Small changes * Fix body scrolling and url cursor jumping * Removed duplication of webpack port * Remove workspaces reduces * Some small fixes * Added sync modal and opt-in setting * Good start to sync flow * Refactored modal footer css * Update sync status * Sync logger * A bit better logging * Fixed a bunch of sync-related bugs * Fixed signup form button * Gravatar component * Split sync modal into tabs * Tidying * Some more error handling * start sending 'user agent * Login/signup error handling * Use real UUIDs * Fixed tests * Remove unused function * Some extra checks * Moved cloud sync setting to about page * Some small changes * Some things
94 lines
2.3 KiB
JavaScript
94 lines
2.3 KiB
JavaScript
import React, {Component} from 'react';
|
|
import Modal from '../base/Modal';
|
|
import ModalBody from '../base/ModalBody';
|
|
import ModalHeader from '../base/ModalHeader';
|
|
import ModalFooter from '../base/ModalFooter';
|
|
|
|
class PromptModal extends Component {
|
|
constructor (props) {
|
|
super(props);
|
|
|
|
this.state = {
|
|
headerName: 'Not Set',
|
|
defaultValue: '',
|
|
submitName: 'Not Set',
|
|
selectText: false,
|
|
hint: null
|
|
};
|
|
}
|
|
|
|
_onSubmit (e) {
|
|
e.preventDefault();
|
|
|
|
this._onSubmitCallback && this._onSubmitCallback(this._input.value);
|
|
this.modal.hide();
|
|
}
|
|
|
|
_setDefaultValueFromState () {
|
|
if (this.state.defaultValue) {
|
|
this._input.value = this.state.defaultValue;
|
|
}
|
|
|
|
// Need to do this after render because modal focuses itself too
|
|
setTimeout(() => {
|
|
this._input.focus();
|
|
if (this.state.selectText) {
|
|
this._input.select();
|
|
}
|
|
}, 100);
|
|
}
|
|
|
|
show ({headerName, defaultValue, submitName, selectText, hint}) {
|
|
this.modal.show();
|
|
|
|
return new Promise(resolve => {
|
|
this._onSubmitCallback = resolve;
|
|
|
|
this.setState({
|
|
headerName,
|
|
defaultValue,
|
|
submitName,
|
|
selectText,
|
|
hint
|
|
})
|
|
});
|
|
}
|
|
|
|
componentDidUpdate () {
|
|
this._setDefaultValueFromState();
|
|
}
|
|
|
|
render () {
|
|
const {extraProps} = this.props;
|
|
const {submitName, headerName, hint} = this.state;
|
|
|
|
return (
|
|
<Modal ref={m => this.modal = m} {...extraProps}>
|
|
<ModalHeader>{headerName}</ModalHeader>
|
|
<ModalBody className="wide">
|
|
<form onSubmit={e => this._onSubmit(e)} className="wide pad">
|
|
<div className="form-control form-control--outlined form-control--wide">
|
|
<input ref={n => this._input = n} type="text"/>
|
|
</div>
|
|
</form>
|
|
</ModalBody>
|
|
<ModalFooter>
|
|
<div className="margin-left faint italic txt-sm tall">{hint ? `* ${hint}` : ''}</div>
|
|
<div>
|
|
<button className="btn" onClick={() => this.modal.hide()}>
|
|
Cancel
|
|
</button>
|
|
<button className="btn" onClick={this._onSubmit.bind(this)}>
|
|
{submitName || 'Save'}
|
|
</button>
|
|
</div>
|
|
</ModalFooter>
|
|
</Modal>
|
|
)
|
|
}
|
|
}
|
|
|
|
PromptModal.propTypes = {};
|
|
|
|
export default PromptModal;
|