mirror of
https://github.com/Kong/insomnia
synced 2024-11-08 14:49:53 +00:00
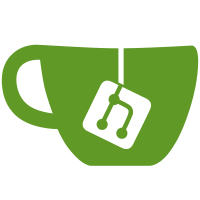
* Move basic auth routes to a separate file * Stop slowing requests down artificially * Add initial oauth routes * Mount oidc routes under /oidc * Enable all forms of oauth that Insomnia supports * Add oauth request collection fixture * Update playwright config * Use 127.0.0.1 instead of localhost * simple oauth2 test * Make the playwright extension work * Move oauth tests to a separate file * Test all oauth flows * Mark test as slow * Wait for load state for new pages * Use locators consistently * Add playwright to recommended extensions * Add instructions for how to use the playwright extension * update selectors and use fill * Fix markdown lint Co-authored-by: jackkav <jackkav@gmail.com> Co-authored-by: gatzjames <jamesgatzos@gmail.com>
41 lines
964 B
TypeScript
41 lines
964 B
TypeScript
import express from 'express';
|
|
|
|
import { basicAuthRouter } from './basic-auth';
|
|
import { oauthRoutes } from './oauth';
|
|
|
|
const app = express();
|
|
const port = 4010;
|
|
|
|
app.get('/pets/:id', (req, res) => {
|
|
res.status(200).send({ id: req.params.id });
|
|
});
|
|
|
|
app.get('/sleep', (_req, res) => {
|
|
res.status(200).send({ sleep: true });
|
|
});
|
|
|
|
app.get('/cookies', (_req, res) => {
|
|
res
|
|
.status(200)
|
|
.header('content-type', 'text/plain')
|
|
.cookie('insomnia-test-cookie', 'value123')
|
|
.send(`${_req.headers['cookie']}`);
|
|
});
|
|
|
|
app.use('/file', express.static('fixtures/files'));
|
|
|
|
app.use('/auth/basic', basicAuthRouter);
|
|
|
|
app.get('/delay/seconds/:duration', (req, res) => {
|
|
const delaySec = Number.parseInt(req.params.duration || '2');
|
|
setTimeout(function() {
|
|
res.send(`Delayed by ${delaySec} seconds`);
|
|
}, delaySec * 1000);
|
|
});
|
|
|
|
app.use('/oidc', oauthRoutes(port));
|
|
|
|
app.listen(port, () => {
|
|
console.log(`Listening at http://localhost:${port}`);
|
|
});
|