mirror of
https://github.com/Kong/insomnia
synced 2024-11-08 14:49:53 +00:00
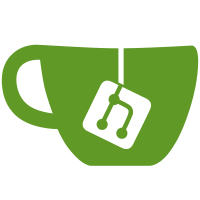
* Got request moving working but is pretty messy * Can now drag between groups * Minor stuff * Prevent deletion of last workspace * Fixed some things * Copy-pasta RequestGroup drag-n-drop * Closes #2
40 lines
873 B
JavaScript
40 lines
873 B
JavaScript
/**
|
|
* Persist all state children that don't have the property "dontPersist"
|
|
*
|
|
* @param key localStorage key to persist to
|
|
* @returns {function()}
|
|
*/
|
|
export default function (key) {
|
|
let timeout = null;
|
|
return _ref => {
|
|
const {getState} = _ref;
|
|
|
|
return next => action => {
|
|
clearTimeout(timeout);
|
|
timeout = setTimeout(() => {
|
|
const state = getState();
|
|
const stateToSave = {};
|
|
|
|
// Check for a `doNotPersist` property on the state
|
|
Object.keys(state)
|
|
.filter(k => !state[k].doNotPersist)
|
|
.map(k => {
|
|
stateToSave[k] = state[k]
|
|
});
|
|
|
|
localStorage[key] = JSON.stringify(stateToSave);
|
|
}, 1000);
|
|
|
|
return next(action);
|
|
};
|
|
}
|
|
}
|
|
|
|
export function getState (key) {
|
|
if (!localStorage[key]) {
|
|
return undefined;
|
|
}
|
|
|
|
return JSON.parse(localStorage[key]);
|
|
}
|