mirror of
https://github.com/Kong/insomnia
synced 2024-11-08 14:49:53 +00:00
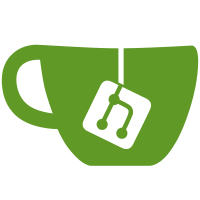
* Start on workspace dropdown and upgrade fontawesome * WorkspaceDropdown start and Elm components! * Lots of CSS shit * Refactor some db stuff and move filter out of sidebar * Adjust dropdown css * Handle duplicate header names, and stuff * Shitty cookies tab * fixed cookie table a bit * Modal refactor * Starteed cookie modal design * Better cookie storage and filter cookie modal * Cookie editor round 1 * Fix kve cursor jumping and form encoding templating * New cookies now show up in filter * Checkpoint * Stuff and fix environments css * Added manage cookies button to cookie pane * Fix accidental sidebar item drag on sidebar resize * Environments modal is looking pretty good now * Pretty much done environments nad cookies * Some changes * Fixed codemirror in modals * Fixed some things * Add basic proxy support * Updated shortcuts * Code snippet generation * Some style * bug fix * Code export now gets cookies for correct domain
86 lines
2.1 KiB
JavaScript
86 lines
2.1 KiB
JavaScript
import React, {PropTypes, Component} from 'react';
|
|
|
|
import EnvironmentEditor from '../editors/EnvironmentEditor';
|
|
import Modal from '../base/Modal';
|
|
import ModalBody from '../base/ModalBody';
|
|
import ModalHeader from '../base/ModalHeader';
|
|
import ModalFooter from '../base/ModalFooter';
|
|
|
|
|
|
class EnvironmentEditModal extends Component {
|
|
constructor (props) {
|
|
super(props);
|
|
|
|
this.state = {
|
|
requestGroup: null,
|
|
isValid: true
|
|
}
|
|
}
|
|
|
|
_saveChanges () {
|
|
if (!this._envEditor.isValid()) {
|
|
return;
|
|
}
|
|
|
|
const environment = this._envEditor.getValue();
|
|
const {requestGroup} = this.state;
|
|
|
|
this.props.onChange(Object.assign({}, requestGroup, {environment}));
|
|
}
|
|
|
|
_didChange () {
|
|
this._saveChanges();
|
|
|
|
const isValid = this._envEditor.isValid();
|
|
|
|
if (this.state.isValid !== isValid) {
|
|
this.setState({isValid});
|
|
}
|
|
}
|
|
|
|
show (requestGroup) {
|
|
this.modal.show();
|
|
this.setState({requestGroup});
|
|
}
|
|
|
|
toggle (requestGroup) {
|
|
this.modal.toggle();
|
|
this.setState({requestGroup});
|
|
}
|
|
|
|
render () {
|
|
const {requestGroup, isValid} = this.state;
|
|
|
|
return (
|
|
<Modal ref={m => this.modal = m} tall={true} top={true} {...this.props}>
|
|
<ModalHeader>Environment Overrides (JSON Format)</ModalHeader>
|
|
<ModalBody noScroll={true}>
|
|
<EnvironmentEditor
|
|
ref={node => this._envEditor = node}
|
|
key={requestGroup ? requestGroup._id : 'n/a'}
|
|
environment={requestGroup ? requestGroup.environment : {}}
|
|
didChange={this._didChange.bind(this)}
|
|
lightTheme={true}
|
|
/>
|
|
</ModalBody>
|
|
<ModalFooter>
|
|
<div className="pull-right">
|
|
<button className="btn" disabled={!isValid} onClick={e => this.modal.hide()}>
|
|
Done
|
|
</button>
|
|
</div>
|
|
<div className="pad faint italic txt-sm tall">
|
|
* this can be used to override data in the global environment
|
|
</div>
|
|
</ModalFooter>
|
|
</Modal>
|
|
);
|
|
}
|
|
}
|
|
|
|
EnvironmentEditModal.propTypes = {
|
|
onChange: PropTypes.func.isRequired
|
|
};
|
|
|
|
export default EnvironmentEditModal;
|