mirror of
https://github.com/Kong/insomnia
synced 2024-11-08 14:49:53 +00:00
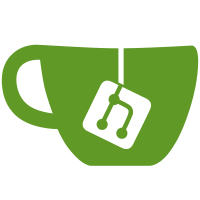
* Start working on insomnia-testing package to generate Mocha code * Moved some things around * Integration tests and ability to run multiple files * Fix integration tests * Rename runSuite to runMochaTests * Add types for test results * Fix lint errors * Play with Chia assertions * insomnia and chai globals, plus a bunch of improvements * Stub chai and axios Flow types * Ability to reference requests by ID * Fix chai flow-typed location * Address PR comments (small tweaks) * Basic UI going * Lots and lots and lots * Pretty-much done with the unit test UI * Minor CSS tweak * Activity bar triple toggle and more * Minor tweak * Unit Test and Suite deletion * Bump Designer version * Fix eslint stuff * Fix insomnia-testing tests * Fix tests * lib@2.2.9 * Remove tests tab from response pane * Hook up Insomnia networking * Fix linting * Bump version for alpha * Remove extra ActivityToggleSwitch * Remove unused import * Add test:pre-release script that excludes CLI tests * Less repetition * Clean some things up * Tweaks after self-cr * Undo request ID tag * Swap out switch for new activity toggle component * Extra check * Remove dead code * Delete dead test * Oops, revert example code * PR feedback * More PR comment addresses
54 lines
1.2 KiB
JavaScript
54 lines
1.2 KiB
JavaScript
// @flow
|
|
import * as db from '../common/database';
|
|
import type { BaseModel } from './index';
|
|
|
|
export const name = 'Unit Test';
|
|
export const type = 'UnitTest';
|
|
export const prefix = 'ut';
|
|
export const canDuplicate = true;
|
|
export const canSync = true;
|
|
|
|
type BaseUnitTest = {
|
|
name: string,
|
|
code: string,
|
|
requestId: string | null,
|
|
};
|
|
|
|
export type UnitTest = BaseModel & BaseUnitTest;
|
|
|
|
export function init() {
|
|
return {
|
|
requestId: null,
|
|
name: 'My Test',
|
|
code: '',
|
|
};
|
|
}
|
|
|
|
export function migrate(doc: UnitTest): UnitTest {
|
|
return doc;
|
|
}
|
|
|
|
export function create(patch: $Shape<UnitTest> = {}): Promise<UnitTest> {
|
|
if (!patch.parentId) {
|
|
throw new Error('New UnitTest missing `parentId` ' + JSON.stringify(patch));
|
|
}
|
|
|
|
return db.docCreate(type, patch);
|
|
}
|
|
|
|
export function remove(unitTest: UnitTest): Promise<void> {
|
|
return db.remove(unitTest);
|
|
}
|
|
|
|
export function update(unitTest: UnitTest, patch: $Shape<UnitTest> = {}): Promise<UnitTest> {
|
|
return db.docUpdate(unitTest, patch);
|
|
}
|
|
|
|
export function getByParentId(parentId: string): Promise<UnitTest | null> {
|
|
return db.getWhere(type, { parentId });
|
|
}
|
|
|
|
export function all(): Promise<Array<UnitTest>> {
|
|
return db.all(type);
|
|
}
|