mirror of
https://github.com/Kong/insomnia
synced 2024-11-08 14:49:53 +00:00
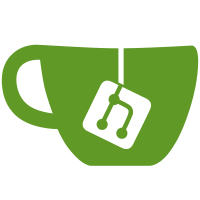
* Start on workspace dropdown and upgrade fontawesome * WorkspaceDropdown start and Elm components! * Lots of CSS shit * Refactor some db stuff and move filter out of sidebar * Adjust dropdown css * Handle duplicate header names, and stuff * Shitty cookies tab * fixed cookie table a bit * Modal refactor * Starteed cookie modal design * Better cookie storage and filter cookie modal * Cookie editor round 1 * Fix kve cursor jumping and form encoding templating * New cookies now show up in filter * Checkpoint * Stuff and fix environments css * Added manage cookies button to cookie pane * Fix accidental sidebar item drag on sidebar resize * Environments modal is looking pretty good now * Pretty much done environments nad cookies * Some changes * Fixed codemirror in modals * Fixed some things * Add basic proxy support * Updated shortcuts * Code snippet generation * Some style * bug fix * Code export now gets cookies for correct domain
101 lines
3.0 KiB
JavaScript
101 lines
3.0 KiB
JavaScript
import React, {PropTypes, Component} from 'react';
|
|
import {Cookie} from 'tough-cookie';
|
|
|
|
import CookieInput from '../CookieInput';
|
|
import {cookieToString} from '../../lib/cookies';
|
|
|
|
|
|
class CookiesEditor extends Component {
|
|
_handleCookieUpdate (cookie, cookieStr) {
|
|
const newCookie = Cookie.parse(cookieStr);
|
|
this.props.onCookieUpdate(cookie, newCookie);
|
|
}
|
|
|
|
_handleCookieAdd () {
|
|
const newCookie = new Cookie({
|
|
key: 'foo',
|
|
value: 'bar',
|
|
domain: this.props.newCookieDomainName,
|
|
path: '/'
|
|
});
|
|
|
|
this.props.onCookieAdd(newCookie);
|
|
}
|
|
|
|
_handleDeleteCookie (cookie) {
|
|
this.props.onCookieDelete(cookie);
|
|
}
|
|
|
|
render () {
|
|
const {cookies} = this.props;
|
|
return (
|
|
<div>
|
|
<table className="cookie-edit-table table--striped">
|
|
<thead>
|
|
<tr>
|
|
<th style={{minWidth: '10rem'}}>Domain</th>
|
|
<th style={{width: '90%'}}>Cookie</th>
|
|
<th style={{width: '2rem'}} className="text-right">
|
|
<button className="btn btn--super-compact"
|
|
onClick={e => this._handleCookieAdd()}
|
|
title="Add cookie">
|
|
<i className="fa fa-plus-circle"></i>
|
|
</button>
|
|
</th>
|
|
</tr>
|
|
</thead>
|
|
<tbody key={cookies.length}>
|
|
{cookies.map((cookie, i) => {
|
|
const cookieString = cookieToString(Cookie.fromJSON(JSON.stringify(cookie)));
|
|
|
|
return (
|
|
<tr className="selectable" key={i}>
|
|
<td>{cookie.domain}</td>
|
|
<td>
|
|
<div className="form-control form-control--underlined no-margin">
|
|
<CookieInput
|
|
defaultValue={cookieString}
|
|
onChange={value => this._handleCookieUpdate(cookie, value)}
|
|
/>
|
|
</div>
|
|
</td>
|
|
<td className="text-right">
|
|
<button className="btn btn--super-compact"
|
|
onClick={e => this._handleDeleteCookie(cookie)}
|
|
title="Delete cookie">
|
|
<i className="fa fa-trash-o"></i>
|
|
</button>
|
|
</td>
|
|
</tr>
|
|
)
|
|
})}
|
|
</tbody>
|
|
</table>
|
|
{cookies.length === 0 ? (
|
|
<div className="pad faint italic text-center">
|
|
<p>
|
|
I couldn't find any cookies for you.
|
|
</p>
|
|
<p>
|
|
<button className="btn btn--super-compact btn--outlined"
|
|
onClick={e => this._handleCookieAdd()}>
|
|
Add Cookie <i className="fa fa-plus-circle"></i>
|
|
</button>
|
|
</p>
|
|
</div>
|
|
) : null}
|
|
</div>
|
|
);
|
|
}
|
|
}
|
|
|
|
CookiesEditor.propTypes = {
|
|
onCookieUpdate: PropTypes.func.isRequired,
|
|
onCookieAdd: PropTypes.func.isRequired,
|
|
onCookieDelete: PropTypes.func.isRequired,
|
|
cookies: PropTypes.array.isRequired,
|
|
newCookieDomainName: PropTypes.string.isRequired
|
|
};
|
|
|
|
export default CookiesEditor;
|