mirror of
https://github.com/Kong/insomnia
synced 2024-11-08 06:39:48 +00:00
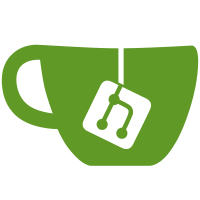
* More shortcuts and accessibility tweaks * Use hotkey objects everywhere * A few last-minute tweaks
58 lines
1.3 KiB
JavaScript
58 lines
1.3 KiB
JavaScript
// @flow
|
|
import React from 'react';
|
|
import ReactDOM from 'react-dom';
|
|
import autobind from 'autobind-decorator';
|
|
import {isMac} from '../../common/constants';
|
|
|
|
type Props = {
|
|
onKeydown: Function,
|
|
children: React.Children,
|
|
disabled?: boolean,
|
|
scoped?: boolean,
|
|
stopMetaPropagation?: boolean
|
|
};
|
|
|
|
@autobind
|
|
class KeydownBinder extends React.Component {
|
|
props: Props;
|
|
|
|
_handleKeydown (e: KeyboardEvent) {
|
|
const {stopMetaPropagation, onKeydown, disabled} = this.props;
|
|
|
|
if (disabled) {
|
|
return;
|
|
}
|
|
|
|
const isMeta = isMac() ? e.metaKey : e.ctrlKey;
|
|
if (stopMetaPropagation && isMeta) {
|
|
e.stopPropagation();
|
|
}
|
|
|
|
onKeydown(e);
|
|
}
|
|
|
|
componentDidMount () {
|
|
if (this.props.scoped) {
|
|
const el = ReactDOM.findDOMNode(this);
|
|
el && el.addEventListener('keydown', this._handleKeydown);
|
|
} else {
|
|
document.body && document.body.addEventListener('keydown', this._handleKeydown);
|
|
}
|
|
}
|
|
|
|
componentWillUnmount () {
|
|
if (this.props.scoped) {
|
|
const el = ReactDOM.findDOMNode(this);
|
|
el && el.removeEventListener('keydown', this._handleKeydown);
|
|
} else {
|
|
document.body && document.body.removeEventListener('keydown', this._handleKeydown);
|
|
}
|
|
}
|
|
|
|
render () {
|
|
return this.props.children;
|
|
}
|
|
}
|
|
|
|
export default KeydownBinder;
|