mirror of
https://github.com/Kong/insomnia
synced 2024-11-08 14:49:53 +00:00
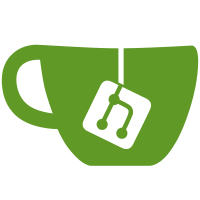
* make all migration functions non-async * migrate is not a promise * simplify scope migration * remove apispec and fix test * fix tests * fix tests, for real this time * removed api spec from collection * default doc name to my-spec.yaml again * fix apispec typing * remove pointless generic * fix type * Create an api spec on first launch * Remove flaky firstLaunch data creation * fix test * create apispec only when scope is deisgn * oauth test left * gitlab test --------- Co-authored-by: gatzjames <jamesgatzos@gmail.com>
92 lines
3.5 KiB
TypeScript
92 lines
3.5 KiB
TypeScript
import { expect, Locator } from '@playwright/test';
|
|
|
|
import { loadFixture } from '../../playwright/paths';
|
|
import { test } from '../../playwright/test';
|
|
|
|
test.describe('gRPC interactions', () => {
|
|
|
|
test.slow(process.platform === 'darwin' || process.platform === 'win32', 'Slow app start on these platforms');
|
|
let statusTag: Locator;
|
|
let responseBody: Locator;
|
|
let streamMessage: Locator;
|
|
|
|
test.beforeEach(async ({ app, page }) => {
|
|
await page.getByRole('button', { name: 'Create' }).click();
|
|
|
|
const text = await loadFixture('grpc.yaml');
|
|
await app.evaluate(async ({ clipboard }, text) => clipboard.writeText(text), text);
|
|
|
|
await page.getByRole('menuitem', { name: 'Import' }).click();
|
|
await page.getByText('Clipboard').click();
|
|
await page.getByRole('button', { name: 'Scan' }).click();
|
|
await page.getByRole('button', { name: 'Import' }).click();
|
|
await page.getByText('CollectionPreRelease gRPCjust now').click();
|
|
statusTag = page.locator('[data-testid="response-status-tag"]:visible');
|
|
responseBody = page.locator('[data-testid="response-pane"] >> [data-testid="CodeEditor"]:visible', {
|
|
has: page.locator('.CodeMirror-activeline'),
|
|
});
|
|
streamMessage = page.locator('[data-testid="request-pane"] button:has-text("Stream")');
|
|
await page.locator('button:has-text("Route Guide ExampleOPEN")').click();
|
|
});
|
|
|
|
test('can send unidirectional requests', async ({ page }) => {
|
|
await page.getByRole('button', { name: 'gRPC Unary', exact: true }).click();
|
|
await page.locator('[data-testid="request-pane"] >> text=Unary').click();
|
|
await page.click('text=Send');
|
|
|
|
// Check for the single Unary response
|
|
await page.click('text=Response 1');
|
|
await expect(statusTag).toContainText('0 OK');
|
|
await expect(responseBody).toContainText('Berkshire Valley Management Area Trail');
|
|
});
|
|
|
|
test('can send bidirectional requests', async ({ page }) => {
|
|
await page.click('button:has-text("gRPCBidirectional Stream")');
|
|
await page.locator('text=Bi-directional Streaming').click();
|
|
await page.click('text=Start');
|
|
|
|
// Stream 3 client messages
|
|
await streamMessage.click();
|
|
await streamMessage.click();
|
|
await streamMessage.click();
|
|
|
|
// Check for the 3rd stream and response
|
|
await page.locator('text=Stream 3').click();
|
|
await page.locator('text=Response 3').click();
|
|
|
|
// Finish the stream
|
|
await page.locator('text=Commit').click();
|
|
await expect(statusTag).toContainText('0 OK');
|
|
});
|
|
|
|
test('can send client stream requests', async ({ page }) => {
|
|
await page.click('button:has-text("gRPCClient Stream")');
|
|
await page.click('text=Client Streaming');
|
|
await page.click('text=Start');
|
|
|
|
// Stream 3 client messages
|
|
await streamMessage.click();
|
|
await streamMessage.click();
|
|
await streamMessage.click();
|
|
|
|
// Finish the stream and check response
|
|
await page.locator('text=Commit').click();
|
|
await page.locator('text=Stream 3').click();
|
|
await page.locator('text=Response 1').click();
|
|
await expect(statusTag).toContainText('0 OK');
|
|
await expect(responseBody).toContainText('point_count": 3');
|
|
});
|
|
|
|
test('can send server stream requests', async ({ page }) => {
|
|
await page.click('button:has-text("gRPCServer Stream")');
|
|
await page.click('text=Server Streaming');
|
|
await page.click('text=Start');
|
|
|
|
// Check response
|
|
await expect(statusTag).toContainText('0 OK');
|
|
await page.locator('text=Response 64').click();
|
|
await expect(responseBody).toContainText('3 Hasta Way');
|
|
});
|
|
|
|
});
|