mirror of
https://github.com/Kong/insomnia
synced 2024-11-08 14:49:53 +00:00
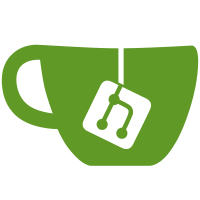
* VCS proof of concept underway! * Stuff * Some things * Replace deprecated Electron makeSingleInstance * Rename `window` variables so not to be confused with window object * Don't unnecessarily update request when URL does not change * Regenerate package-lock * Fix tests + ESLint * Publish - insomnia-app@1.0.49 - insomnia-cookies@0.0.12 - insomnia-httpsnippet@1.16.18 - insomnia-importers@2.0.13 - insomnia-libcurl@0.0.23 - insomnia-prettify@0.1.7 - insomnia-url@0.1.6 - insomnia-xpath@1.0.9 - insomnia-plugin-base64@1.0.6 - insomnia-plugin-cookie-jar@1.0.8 - insomnia-plugin-core-themes@1.0.5 - insomnia-plugin-default-headers@1.1.9 - insomnia-plugin-file@1.0.7 - insomnia-plugin-hash@1.0.7 - insomnia-plugin-jsonpath@1.0.12 - insomnia-plugin-now@1.0.11 - insomnia-plugin-os@1.0.13 - insomnia-plugin-prompt@1.1.9 - insomnia-plugin-request@1.0.18 - insomnia-plugin-response@1.0.16 - insomnia-plugin-uuid@1.0.10 * Broken but w/e * Some tweaks * Big refactor. Create local snapshots and push done * POC merging and a lot of improvements * Lots of work done on initial UI/UX * Fix old tests * Atomic writes and size-based batches * Update StageEntry definition once again to be better * Factor out GraphQL query logic * Merge algorithm, history modal, other minor things * Fix test * Merge, checkout, revert w/ user changes now work * Force UI to refresh when switching branches changes active request * Rough draft pull() and some cleanup * E2EE stuff and some refactoring * Add ability to share project with team and fixed tests * VCS now created in root component and better remote project handling * Remove unused definition * Publish - insomnia-account@0.0.2 - insomnia-app@1.1.1 - insomnia-cookies@0.0.14 - insomnia-httpsnippet@1.16.20 - insomnia-importers@2.0.15 - insomnia-libcurl@0.0.25 - insomnia-prettify@0.1.9 - insomnia-sync@0.0.2 - insomnia-url@0.1.8 - insomnia-xpath@1.0.11 - insomnia-plugin-base64@1.0.8 - insomnia-plugin-cookie-jar@1.0.10 - insomnia-plugin-core-themes@1.0.7 - insomnia-plugin-file@1.0.9 - insomnia-plugin-hash@1.0.9 - insomnia-plugin-jsonpath@1.0.14 - insomnia-plugin-now@1.0.13 - insomnia-plugin-os@1.0.15 - insomnia-plugin-prompt@1.1.11 - insomnia-plugin-request@1.0.20 - insomnia-plugin-response@1.0.18 - insomnia-plugin-uuid@1.0.12 * Move some deps around * Fix Flow errors * Update package.json * Fix eslint errors * Fix tests * Update deps * bootstrap insomnia-sync * TRy fixing appveyor * Try something else * Bump lerna * try powershell * Try again * Fix imports * Fixed errors * sync types refactor * Show remote projects in workspace dropdown * Improved pulling of non-local workspaces * Loading indicators and some tweaks * Clean up sync staging modal * Some sync improvements: - No longer store stage - Upgrade Electron - Sync UI/UX improvements * Fix snyc tests * Upgraded deps and hot loader tweaks (it's broken for some reason) * Fix tests * Branches dialog, network refactoring, some tweaks * Fixed merging when other branch is empty * A bunch of small fixes from real testing * Fixed pull merge logic * Fix tests * Some bug fixes * A few small tweaks * Conflict resolution and other improvements * Fix tests * Add revert changes * Deal with duplicate projects per workspace * Some tweaks and accessibility improvements * Tooltip accessibility * Fix API endpoint * Fix tests * Remove jest dep from insomnia-importers
103 lines
4.2 KiB
JavaScript
103 lines
4.2 KiB
JavaScript
import * as crypt from '../crypt';
|
|
|
|
describe('crypt', () => {
|
|
describe('deriveKey()', () => {
|
|
it('derives a key properly', async () => {
|
|
const result = await crypt.deriveKey('Password', 'email', 'salt');
|
|
const expected = 'fb058595c02ae9660ed7098273bf50e49407942ecc437bf317638d76c4578eae';
|
|
|
|
expect(result).toBe(expected);
|
|
});
|
|
});
|
|
|
|
describe('AES', () => {
|
|
it('encrypts and decrypts', () => {
|
|
const key = {
|
|
kty: 'oct',
|
|
alg: 'A256GCM',
|
|
ext: true,
|
|
key_ops: ['encrypt', 'decrypt'],
|
|
k: '5hs1f2xuiNPHUp11i6SWlsqYpWe_hWPcEKucZlwBfFE',
|
|
};
|
|
|
|
const resultEncrypted = crypt.encryptAES(key, 'Hello World!', 'additional data');
|
|
const resultDecrypted = crypt.decryptAES(key, resultEncrypted);
|
|
|
|
expect(resultDecrypted).toEqual('Hello World!');
|
|
});
|
|
});
|
|
|
|
describe('AES Buffer', () => {
|
|
it('encrypts and decrypts', () => {
|
|
const key = {
|
|
kty: 'oct',
|
|
alg: 'A256GCM',
|
|
ext: true,
|
|
key_ops: ['encrypt', 'decrypt'],
|
|
k: '5hs1f2xuiNPHUp11i6SWlsqYpWe_hWPcEKucZlwBfFE',
|
|
};
|
|
|
|
const resultEncrypted = crypt.encryptAESBuffer(key, Buffer.from('Hello World!', 'utf8'));
|
|
const resultDecrypted = crypt.decryptAESToBuffer(key, resultEncrypted);
|
|
|
|
expect(resultDecrypted).toEqual(Buffer.from('Hello World!', 'utf8'));
|
|
});
|
|
});
|
|
|
|
describe('RSA', () => {
|
|
it('encrypts and decrypts', () => {
|
|
const privateKey = {
|
|
alg: 'RSA-OAEP-256',
|
|
kty: 'RSA',
|
|
key_ops: ['decrypt'],
|
|
ext: true,
|
|
d:
|
|
'UkouuQID2o9Q6VyiRMmK8ETPsAHWEL2HMYwy34c4nTpM7KfqlNeMzs6HmbuEfx-bwUvTqOO4Tz7FZw4ILD6s5sE9' +
|
|
'xqIxmV-fIiqiBI4aWKozxgf9OJZWKqru3loSd923O3fI3oa9ZCTaKc1U0bYOB-XP2Q_hB2M64Hb63-McXAM0RQwN' +
|
|
'R5vh_TweqcaBiXAyhuYl2NarOwrbSlSttkhZzy4i-otulPGkW61I5rNflsSmnYEijmD7zl9EouEOYcHlJGmNLHjG' +
|
|
'nHlb-avvwvER5NZVDwd6vT61QR1wwpnYSjVH_Z_OrqJu8U2J64J_MaZPJog3KbPYqZnGcxJ9ldnSYQ',
|
|
dp:
|
|
'SnO3kTAogveLWkqSuDVxQLOo4QXEq-Us_lM00dfGIuZrfWnyqOd6_NJKu-G62PCQgUMBy7r_f3N3sOseRVl_5fy3' +
|
|
'kd21n7WmnQcMGBm-VRathT0nOz-fhzbwCJwtuI1g36YtaCQEQiC4pYxMYmXaX1WGPhL4rGSNz5SMHrPvyTU',
|
|
dq:
|
|
'eaK2-w2Jb7rWWYhLon-RKlzWXTPHjnt1JfLkD_D12FNE6KAcbyIETDid8_sXnqj3oCr3HrO-AuNI87zZJ4UVsy2J' +
|
|
'mvNNVFTn0s8R6TZ9_o9LCXBdBcRST5qpAax0t-duHRaKMuWPG3xAb2Uub6T42C7yd-mpOIRo7uSHFGQGzWk',
|
|
e: 'AQAB',
|
|
n:
|
|
'5wOecCVWaDB2s-ybsCp1BskBW5fj3iH4xja5hNTfW_s-ERgbEoBA_PSF60PC1se4r_oWwFBfIRL9OUTYwYOuQOuC' +
|
|
'rgd7ODa_YBeOjzHUA1b0K5kWXaqPxkmN8kJPISyLdLjCCOwHtTFnqxL9JjnU4aIxy4OU1S5KR6v-XVeLOZtUOm4k' +
|
|
'AhLVfmdzYB1nZmq9xH1O8_acIUoWDbAWX6fIXhPhn7jCuCO4WZQVDxZ5_bc27UVhR4VYe2Our7aESUQ5ZyMtYNym' +
|
|
'o9Oy0y_m3OS6W_JR_feXBbxRCBuGf7fjnvV9ohx1ZqLpJFx9_xL7naoVCQhBDfVE31iYz3L6KTIhFQ',
|
|
p:
|
|
'_Pyx-puBM_BQubDg8BZsrssgmECHuieKEwlR19fckczS4dlgQVUemUTr-RqmItj7k-WMG7mWuRgbIrGO1sigpuDy' +
|
|
'uKWkg3KyqoeIvgsaJV05xu8pneVblTXJSCtNMXvYMa6mPNYudUSy8-TlLyLg_w5lUnuA8Mq8xehzCsPrM-0',
|
|
q:
|
|
'6cPuxuW0zaMIVAJpOcLf5D0dA-mLXxmmJoMDodamM7t7_oGYgFR3Os9gtB5TYxsUjjRCWGkDy7ru8arcbTNCnNFd' +
|
|
'PAayzTdWCW-GBs3xswggjmugGupjOnrtD-N1_fG040Ka8UqwMyI1lUapxaKhViR9TNYUz6EAOjxycf8MTMk',
|
|
qi:
|
|
'FMVx12_ioueu052xgFxWdIS_lImUGTrw8Iiw_kp-KKsONtofs91A5GVRtyg_wdXpG2qyomaet1hTlHhLnoI23L2a' +
|
|
'EkQ87SokIpoR9lR8jfIRwLwKKXMc33_bRRQXvWop0yvTzmSGaC0gULcqj0OHiUR1u9Ver1ZvgGz2jh4mP_E',
|
|
};
|
|
|
|
const publicKey = {
|
|
alg: 'RSA-OAEP-256',
|
|
kty: 'RSA',
|
|
key_ops: ['encrypt'],
|
|
e: 'AQAB',
|
|
n:
|
|
'5wOecCVWaDB2s-ybsCp1BskBW5fj3iH4xja5hNTfW_s-ERgbEoBA_PSF60PC1se4r_oWwFBfIRL9OUTYwYOuQO' +
|
|
'uCrgd7ODa_YBeOjzHUA1b0K5kWXaqPxkmN8kJPISyLdLjCCOwHtTFnqxL9JjnU4aIxy4OU1S5KR6v-XVeLOZtU' +
|
|
'Om4kAhLVfmdzYB1nZmq9xH1O8_acIUoWDbAWX6fIXhPhn7jCuCO4WZQVDxZ5_bc27UVhR4VYe2Our7aESUQ5Zy' +
|
|
'MtYNymo9Oy0y_m3OS6W_JR_feXBbxRCBuGf7fjnvV9ohx1ZqLpJFx9_xL7naoVCQhBDfVE31iYz3L6KTIhFQ',
|
|
};
|
|
|
|
const resultEncrypted = crypt.encryptRSAWithJWK(publicKey, 'aaaaaaaaa');
|
|
const resultDecrypted = crypt.decryptRSAWithJWK(privateKey, resultEncrypted);
|
|
|
|
const expectedDecrypted = 'aaaaaaaaa';
|
|
|
|
expect(resultDecrypted.toString()).toEqual(expectedDecrypted);
|
|
});
|
|
});
|
|
});
|