mirror of
https://github.com/Kong/insomnia
synced 2024-11-08 14:49:53 +00:00
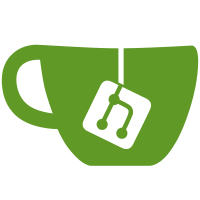
* Removed some unnecessary rendering * Only PureComponents and some other stuff * Removed all functional components * Only deal with nunjucks marks * Remove ref assign functions in modals * Lots of tweaks * A bit snappier * A bit snappier
44 lines
984 B
JavaScript
44 lines
984 B
JavaScript
import React, {PureComponent, PropTypes} from 'react';
|
|
const {clipboard} = require('electron');
|
|
|
|
class CopyButton extends PureComponent {
|
|
state = {showConfirmation: false};
|
|
|
|
_handleClick = e => {
|
|
e.preventDefault();
|
|
e.stopPropagation();
|
|
|
|
clipboard.writeText(this.props.content);
|
|
|
|
this.setState({showConfirmation: true});
|
|
|
|
this._triggerTimeout = setTimeout(() => {
|
|
this.setState({showConfirmation: false});
|
|
}, 2000);
|
|
};
|
|
|
|
componentWillUnmount () {
|
|
clearTimeout(this._triggerTimeout);
|
|
}
|
|
|
|
render () {
|
|
const {content, children, ...other} = this.props;
|
|
const {showConfirmation} = this.state;
|
|
|
|
return (
|
|
<button {...other} onClick={this._handleClick}>
|
|
{showConfirmation ?
|
|
<span>Copied <i className="fa fa-check-circle-o"/></span> :
|
|
(children || 'Copy to Clipboard')
|
|
}
|
|
</button>
|
|
)
|
|
}
|
|
}
|
|
|
|
CopyButton.propTypes = {
|
|
content: PropTypes.string.isRequired
|
|
};
|
|
|
|
export default CopyButton;
|