mirror of
https://github.com/Kong/insomnia
synced 2024-11-08 14:49:53 +00:00
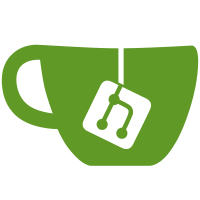
* Removed some unnecessary rendering * Only PureComponents and some other stuff * Removed all functional components * Only deal with nunjucks marks * Remove ref assign functions in modals * Lots of tweaks * A bit snappier * A bit snappier
45 lines
1.3 KiB
JavaScript
45 lines
1.3 KiB
JavaScript
import React, {PureComponent, PropTypes} from 'react';
|
|
import {shell} from 'electron';
|
|
import {trackEvent} from '../../../analytics/index';
|
|
import * as querystring from '../../../common/querystring';
|
|
import {getAppVersion} from '../../../common/constants';
|
|
import {isDevelopment} from '../../../common/constants';
|
|
|
|
class Link extends PureComponent {
|
|
_handleClick = e => {
|
|
e && e.preventDefault();
|
|
const {href, onClick} = this.props;
|
|
|
|
// Also call onClick that was passed to us if there was one
|
|
onClick && onClick(e);
|
|
|
|
if (href.match(/^http/i)) {
|
|
const appName = isDevelopment() ? 'Insomnia Dev' : 'Insomnia';
|
|
const qs = `utm_source=${appName}&utm_medium=app&utm_campaign=v${getAppVersion()}`;
|
|
const attributedHref = querystring.joinUrl(href, qs);
|
|
shell.openExternal(attributedHref);
|
|
} else {
|
|
// Don't modify non-http urls
|
|
shell.openExternal(href);
|
|
}
|
|
|
|
trackEvent('Link', 'Click', href);
|
|
};
|
|
|
|
render () {
|
|
const {onClick, button, href, children, ...other} = this.props;
|
|
return button ?
|
|
<button onClick={this._handleClick} {...other}>{children}</button> :
|
|
<a href={href} onClick={this._handleClick} {...other}>{children}</a>
|
|
}
|
|
}
|
|
|
|
Link.propTypes = {
|
|
href: PropTypes.string.isRequired,
|
|
|
|
// Optional
|
|
button: PropTypes.bool
|
|
};
|
|
|
|
export default Link;
|