mirror of
https://github.com/Kong/insomnia
synced 2024-11-08 14:49:53 +00:00
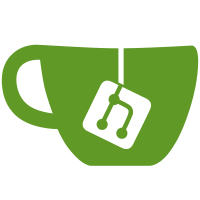
* fix: insomnia open dialog for proto directory can't select directories * uses a named export for selectFileOrFolder (also, removes original js file from rebase) * clears error by leveraging exhaustiveness check * fixes bug: the `name` field is actually for a file filter see the referenced pull request. As for the `extensions: ['*']`, there's no reason I can see to include a filter and then tell the filter to then accept everything. * update selectFileOrFolder mocks * use switch (for exhaustiveness checking) and type selectedFormat * removes unnecessary filters from _save_ dialog from the docs: > The filters specifies an array of file types that can be displayed As suspected, this is not needed. A user is free to save it wherever they want. * adds extension to saved file not sure why this was missing before, but it appears to have been a bug * formatting updates best to "ignore whitespace" for this commit. I did this with the hope of using the `ThunkAction` type from `redux-thunk`, but once I got them all looking good and started adding the type I quickly learned there's quite a bit more work to do in this area before we can have such a thing. I therefore opted to just call it a day at that and take the (no-op) formatting changes and typings. * removes remaining name filters from save dialogs same reason as the 2nd to prior commit - they cause the bug Co-authored-by: Dimitri Mitropoulos <dimitrimitropoulos@gmail.com> Co-authored-by: Opender Singh <opender.singh@konghq.com>
55 lines
1.2 KiB
TypeScript
55 lines
1.2 KiB
TypeScript
import { OpenDialogOptions, remote } from 'electron';
|
|
|
|
interface Options {
|
|
itemTypes?: ('file' | 'directory')[];
|
|
extensions?: string[];
|
|
}
|
|
|
|
interface FileSelection {
|
|
filePath: string;
|
|
canceled: boolean;
|
|
}
|
|
|
|
export const selectFileOrFolder = async ({ itemTypes, extensions }: Options) => {
|
|
// If no types are selected then default to just files and not directories
|
|
const types = itemTypes || ['file'];
|
|
let title = 'Select ';
|
|
|
|
if (types.includes('file')) {
|
|
title += ' File';
|
|
|
|
if (types.length > 2) {
|
|
title += ' or';
|
|
}
|
|
}
|
|
|
|
if (types.includes('directory')) {
|
|
title += ' Directory';
|
|
}
|
|
|
|
const options: OpenDialogOptions = {
|
|
title,
|
|
buttonLabel: 'Select',
|
|
properties: types.map(type => {
|
|
switch (type) {
|
|
case 'file':
|
|
return 'openFile';
|
|
|
|
case 'directory':
|
|
return 'openDirectory';
|
|
}
|
|
}),
|
|
// @ts-expect-error https://github.com/electron/electron/pull/29322
|
|
filters: [{
|
|
extensions: (extensions?.length ? extensions : ['*']),
|
|
}],
|
|
};
|
|
|
|
const { canceled, filePaths } = await remote.dialog.showOpenDialog(options);
|
|
const fileSelection: FileSelection = {
|
|
filePath: filePaths[0],
|
|
canceled,
|
|
};
|
|
return fileSelection;
|
|
};
|