mirror of
https://github.com/Kong/insomnia
synced 2024-11-08 14:49:53 +00:00
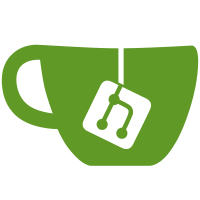
* Start on workspace dropdown and upgrade fontawesome * WorkspaceDropdown start and Elm components! * Lots of CSS shit * Refactor some db stuff and move filter out of sidebar * Adjust dropdown css * Handle duplicate header names, and stuff * Shitty cookies tab * fixed cookie table a bit * Modal refactor * Starteed cookie modal design * Better cookie storage and filter cookie modal * Cookie editor round 1 * Fix kve cursor jumping and form encoding templating * New cookies now show up in filter * Checkpoint * Stuff and fix environments css * Added manage cookies button to cookie pane * Fix accidental sidebar item drag on sidebar resize * Environments modal is looking pretty good now * Pretty much done environments nad cookies * Some changes * Fixed codemirror in modals * Fixed some things * Add basic proxy support * Updated shortcuts * Code snippet generation * Some style * bug fix * Code export now gets cookies for correct domain
74 lines
1.6 KiB
JavaScript
74 lines
1.6 KiB
JavaScript
import {combineReducers} from 'redux';
|
|
|
|
import {trackEvent} from '../../lib/analytics';
|
|
import * as network from '../../lib/network';
|
|
|
|
export const REQUEST_CHANGE_FILTER = 'requests/filter';
|
|
export const REQUEST_SEND_START = 'requests/start';
|
|
export const REQUEST_SEND_STOP = 'requests/stop';
|
|
|
|
|
|
// ~~~~~~~~ //
|
|
// REDUCERS //
|
|
// ~~~~~~~~ //
|
|
|
|
function filterReducer(state = '', action) {
|
|
switch (action.type) {
|
|
|
|
case REQUEST_CHANGE_FILTER:
|
|
const filter = action.filter;
|
|
return Object.assign({}, state, {filter});
|
|
|
|
default:
|
|
return state;
|
|
}
|
|
}
|
|
|
|
function loadingRequestsReducer(state = {}, action) {
|
|
let newState;
|
|
switch (action.type) {
|
|
|
|
case REQUEST_SEND_START:
|
|
newState = Object.assign({}, state);
|
|
newState[action.requestId] = Date.now();
|
|
return newState;
|
|
|
|
case REQUEST_SEND_STOP:
|
|
newState = Object.assign({}, state);
|
|
delete newState[action.requestId];
|
|
return newState;
|
|
|
|
default:
|
|
return state;
|
|
}
|
|
}
|
|
|
|
export default combineReducers({
|
|
filter: filterReducer,
|
|
loadingRequests: loadingRequestsReducer
|
|
});
|
|
|
|
|
|
// ~~~~~~~ //
|
|
// ACTIONS //
|
|
// ~~~~~~~ //
|
|
|
|
export function changeFilter(filter) {
|
|
return {type: REQUEST_CHANGE_FILTER, filter};
|
|
}
|
|
|
|
export function send(request) {
|
|
return dispatch => {
|
|
dispatch({type: REQUEST_SEND_START, requestId: request._id});
|
|
|
|
trackEvent('Request Send');
|
|
|
|
network.send(request._id).then(() => {
|
|
dispatch({type: REQUEST_SEND_STOP, requestId: request._id});
|
|
}, err => {
|
|
console.warn('Error sending request', err);
|
|
dispatch({type: REQUEST_SEND_STOP, requestId: request._id});
|
|
});
|
|
}
|
|
}
|