mirror of
https://github.com/Kong/insomnia
synced 2024-11-08 06:39:48 +00:00
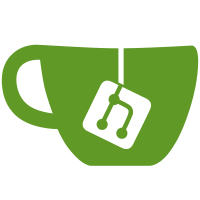
* Add digest auth and response timeline * Some css fixes * Fixed Button * More auth methods, fixed URL regex, and fix gzip * Get rid of negotiate auth * Fix proxy * Fixed GA tracking * Some very small changes and fixes * Fix content type names * Even better auth switching and timeline syntax * Some auth tweaks * CSS tweaks * Reworked toasts * Fixed timer rounding quirk * Request settings and render errors * Fixed tests * Vertical dragging and stuff * Remove beta
55 lines
1.1 KiB
JavaScript
55 lines
1.1 KiB
JavaScript
import * as db from '../common/database';
|
|
|
|
export const name = 'Settings';
|
|
export const type = 'Settings';
|
|
export const prefix = 'set';
|
|
export const canDuplicate = false;
|
|
|
|
export function init () {
|
|
return {
|
|
showPasswords: false,
|
|
useBulkHeaderEditor: false,
|
|
followRedirects: true,
|
|
editorFontSize: 12,
|
|
editorLineWrapping: true,
|
|
editorKeyMap: 'default',
|
|
httpProxy: '',
|
|
httpsProxy: '',
|
|
proxyEnabled: false,
|
|
timeout: 0,
|
|
validateSSL: true,
|
|
forceVerticalLayout: false,
|
|
theme: 'default'
|
|
};
|
|
}
|
|
|
|
export function migrate (doc) {
|
|
return doc;
|
|
}
|
|
|
|
export async function all () {
|
|
const settings = await db.all(type);
|
|
if (settings.length === 0) {
|
|
return [await getOrCreate()];
|
|
} else {
|
|
return settings;
|
|
}
|
|
}
|
|
|
|
export async function create (patch = {}) {
|
|
return db.docCreate(type, patch);
|
|
}
|
|
|
|
export async function update (settings, patch) {
|
|
return db.docUpdate(settings, patch);
|
|
}
|
|
|
|
export async function getOrCreate () {
|
|
const results = await db.all(type);
|
|
if (results.length === 0) {
|
|
return await create();
|
|
} else {
|
|
return results[0];
|
|
}
|
|
}
|