mirror of
https://github.com/Kong/insomnia
synced 2024-11-08 06:39:48 +00:00
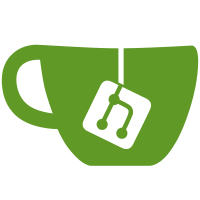
* Start working on insomnia-testing package to generate Mocha code * Moved some things around * Integration tests and ability to run multiple files * Fix integration tests * Rename runSuite to runMochaTests * Add types for test results * Fix lint errors * Play with Chia assertions * insomnia and chai globals, plus a bunch of improvements * Stub chai and axios Flow types * Ability to reference requests by ID * Fix chai flow-typed location * Address PR comments (small tweaks) * Basic UI going * Lots and lots and lots * Pretty-much done with the unit test UI * Minor CSS tweak * Activity bar triple toggle and more * Minor tweak * Unit Test and Suite deletion * Bump Designer version * Fix eslint stuff * Fix insomnia-testing tests * Fix tests * lib@2.2.9 * Remove tests tab from response pane * Hook up Insomnia networking * Fix linting * Bump version for alpha * Remove extra ActivityToggleSwitch * Remove unused import * Add test:pre-release script that excludes CLI tests * Less repetition * Clean some things up * Tweaks after self-cr * Undo request ID tag * Swap out switch for new activity toggle component * Extra check * Remove dead code * Delete dead test * Oops, revert example code * PR feedback * More PR comment addresses
113 lines
2.7 KiB
JavaScript
113 lines
2.7 KiB
JavaScript
// @flow
|
|
import * as React from 'react';
|
|
import styled from 'styled-components';
|
|
|
|
type Props = {
|
|
onClick?: (e: SyntheticEvent<HTMLButtonElement>) => any,
|
|
bg?: 'default' | 'success' | 'notice' | 'warning' | 'danger' | 'surprise' | 'info',
|
|
variant?: 'outlined' | 'contained' | 'text',
|
|
size?: 'default' | 'small',
|
|
};
|
|
|
|
const StyledButton: React.ComponentType<Props> = styled.button`
|
|
color: ${({ bg }) => (bg ? `var(--color-${bg})` : 'var(--color-font)')};
|
|
text-align: center;
|
|
font-size: var(--font-size-sm);
|
|
border-radius: 3px;
|
|
display: inline-flex !important;
|
|
flex-direction: row !important;
|
|
align-items: center !important;
|
|
border: 1px solid transparent;
|
|
|
|
${({ size }) => {
|
|
switch (size) {
|
|
case 'small':
|
|
return `
|
|
padding: 0 calc(var(--padding-md) * 0.8);
|
|
height: calc(var(--line-height-xs) * 0.8);
|
|
font-size: var(--font-size-sm);
|
|
`;
|
|
default:
|
|
return `
|
|
padding: 0 var(--padding-md);
|
|
height: var(--line-height-xs);
|
|
`;
|
|
}
|
|
}}}
|
|
|
|
${({ variant, bg }) => {
|
|
if (variant !== 'outlined') {
|
|
return '';
|
|
}
|
|
|
|
// Inherit border color from text color for colored outlines
|
|
if (bg === 'default') {
|
|
return 'border-color: var(--hl-lg)';
|
|
}
|
|
|
|
// Colored borders inherit from button text color
|
|
return 'border-color: inherit';
|
|
}}}
|
|
|
|
${({ variant, bg }) => {
|
|
if (variant !== 'contained') {
|
|
return 'background-color: transparent;';
|
|
}
|
|
|
|
if (bg === 'default') {
|
|
return 'background-color: var(--hl-xs)';
|
|
}
|
|
|
|
return `background: var(--color-${bg}); color: var(--color-font-${bg})`;
|
|
}}}
|
|
|
|
&:focus,
|
|
&:hover {
|
|
&:not(:disabled) {
|
|
outline: 0;
|
|
${({ variant, bg }) => {
|
|
if (variant === 'contained') {
|
|
// kind of a hack, but using inset box shadow to darken the theme color
|
|
return 'box-shadow: inset 0 0 99px rgba(0, 0, 0, 0.1)';
|
|
}
|
|
|
|
if (bg === 'default') {
|
|
return 'background-color: var(--hl-xs)';
|
|
}
|
|
|
|
return `background-color: rgba(var(--color-${bg}-rgb), 0.1)`;
|
|
}}
|
|
}
|
|
}
|
|
|
|
&:active:not(:disabled) {
|
|
${({ variant, bg }) => {
|
|
if (variant === 'contained') {
|
|
// kind of a hack, but using inset box shadow to darken the theme color
|
|
return 'box-shadow: inset 0 0 99px rgba(0, 0, 0, 0.2)';
|
|
}
|
|
|
|
if (bg === 'default') {
|
|
return 'background-color: var(--hl-sm)';
|
|
}
|
|
|
|
return `background-color: rgba(var(--color-${bg}-rgb), 0.2)`;
|
|
}}
|
|
}
|
|
|
|
&:disabled {
|
|
opacity: 60%;
|
|
}
|
|
`;
|
|
|
|
const Button = ({ variant, bg, size, ...props }: Props) => (
|
|
<StyledButton
|
|
{...props}
|
|
variant={variant || 'outlined'}
|
|
bg={bg || 'default'}
|
|
size={size || 'default'}
|
|
/>
|
|
);
|
|
|
|
export default Button;
|