mirror of
https://github.com/Kong/insomnia
synced 2024-11-08 14:49:53 +00:00
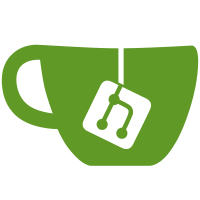
* Removed some unnecessary rendering * Only PureComponents and some other stuff * Removed all functional components * Only deal with nunjucks marks * Remove ref assign functions in modals * Lots of tweaks * A bit snappier * A bit snappier
48 lines
933 B
JavaScript
48 lines
933 B
JavaScript
import React, {PureComponent, PropTypes} from 'react';
|
|
import {Cookie} from 'tough-cookie';
|
|
|
|
|
|
class CookieInput extends PureComponent {
|
|
state = {isValid: true};
|
|
|
|
_handleChange () {
|
|
const isValid = this._isValid();
|
|
|
|
if (isValid) {
|
|
this.props.onChange(this._input.value);
|
|
}
|
|
|
|
this.setState({isValid})
|
|
}
|
|
|
|
_isValid () {
|
|
try {
|
|
const cookie = Cookie.parse(this._input.value);
|
|
return !!(cookie && cookie.domain);
|
|
} catch (e) {
|
|
return false;
|
|
}
|
|
}
|
|
|
|
render () {
|
|
const {defaultValue} = this.props;
|
|
const {isValid} = this.state;
|
|
|
|
return (
|
|
<input
|
|
className={isValid ? '' : 'input--error'}
|
|
ref={n => this._input = n}
|
|
type="text"
|
|
defaultValue={defaultValue}
|
|
onChange={e => this._handleChange(e.target.value)}
|
|
/>
|
|
);
|
|
}
|
|
}
|
|
|
|
CookieInput.propTypes = {
|
|
onChange: PropTypes.func.isRequired
|
|
};
|
|
|
|
export default CookieInput;
|