mirror of
https://github.com/Kong/insomnia
synced 2024-11-08 14:49:53 +00:00
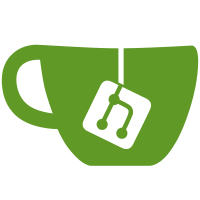
* Removed some unnecessary rendering * Only PureComponents and some other stuff * Removed all functional components * Only deal with nunjucks marks * Remove ref assign functions in modals * Lots of tweaks * A bit snappier * A bit snappier
61 lines
1.4 KiB
JavaScript
61 lines
1.4 KiB
JavaScript
import React, {PropTypes, PureComponent} from 'react';
|
|
import Editor from '../codemirror/Editor';
|
|
import {DEBOUNCE_MILLIS} from '../../../common/constants';
|
|
|
|
class EnvironmentEditor extends PureComponent {
|
|
_handleChange = () => this.props.didChange();
|
|
|
|
_setEditorRef = n => this._editor = n;
|
|
|
|
getValue () {
|
|
return JSON.parse(this._editor.getValue());
|
|
}
|
|
|
|
isValid () {
|
|
try {
|
|
return this.getValue() !== undefined;
|
|
} catch (e) {
|
|
// Failed to parse JSON
|
|
return false;
|
|
}
|
|
}
|
|
|
|
render () {
|
|
const {
|
|
environment,
|
|
editorFontSize,
|
|
editorKeyMap,
|
|
render,
|
|
lineWrapping,
|
|
...props
|
|
} = this.props;
|
|
|
|
return (
|
|
<Editor
|
|
ref={this._setEditorRef}
|
|
fontSize={editorFontSize}
|
|
lineWrapping={lineWrapping}
|
|
keyMap={editorKeyMap}
|
|
onChange={this._handleChange}
|
|
debounceMillis={DEBOUNCE_MILLIS * 6}
|
|
value={JSON.stringify(environment)}
|
|
autoPrettify={true}
|
|
render={render}
|
|
mode="application/json"
|
|
{...props}
|
|
/>
|
|
)
|
|
}
|
|
}
|
|
|
|
EnvironmentEditor.propTypes = {
|
|
environment: PropTypes.object.isRequired,
|
|
didChange: PropTypes.func.isRequired,
|
|
editorFontSize: PropTypes.number.isRequired,
|
|
editorKeyMap: PropTypes.string.isRequired,
|
|
render: PropTypes.func.isRequired,
|
|
lineWrapping: PropTypes.bool.isRequired,
|
|
};
|
|
|
|
export default EnvironmentEditor;
|