mirror of
https://github.com/Kong/insomnia
synced 2024-11-08 14:49:53 +00:00
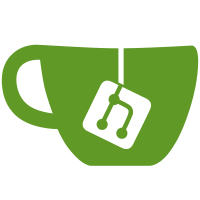
* Proof of concept authorize call * Authorize and Refresh endpoints done * OAuth2 editor started * Some small fixes * Set OAuth headers on request * Started on some OAuth tests * Updated network logic with new OAuth API * OAuth forms and refactor flows * Fix grant type handling * Moved auth handling out of render pipeline * Fixed legacy auth header * Fix vertical center * Prompt user on auth type change * Refresh tokens working (I think) and better UI * Catch same type auth change * POC refresh token and small refactor * Better token handling * LOading state to token refresh * Show o-auth-2 errors * Some minor updates
69 lines
1.4 KiB
JavaScript
69 lines
1.4 KiB
JavaScript
import React, {PureComponent} from 'react';
|
|
import autobind from 'autobind-decorator';
|
|
import Modal from '../base/modal';
|
|
import ModalBody from '../base/modal-body';
|
|
import ModalHeader from '../base/modal-header';
|
|
import ModalFooter from '../base/modal-footer';
|
|
|
|
@autobind
|
|
class AlertModal extends PureComponent {
|
|
constructor (props) {
|
|
super(props);
|
|
|
|
this.state = {
|
|
title: '',
|
|
message: '',
|
|
addCancel: false
|
|
};
|
|
}
|
|
|
|
_setModalRef (m) {
|
|
this.modal = m;
|
|
}
|
|
|
|
_handleOk () {
|
|
this.hide();
|
|
this._okCallback();
|
|
}
|
|
|
|
hide () {
|
|
this.modal.hide();
|
|
}
|
|
|
|
show (options = {}) {
|
|
this.modal.show();
|
|
|
|
const {title, message, addCancel} = options;
|
|
this.setState({title, message, addCancel});
|
|
|
|
return new Promise(resolve => {
|
|
this._okCallback = resolve;
|
|
});
|
|
}
|
|
|
|
render () {
|
|
const {message, title, addCancel} = this.state;
|
|
|
|
return (
|
|
<Modal ref={this._setModalRef} closeOnKeyCodes={[13]}>
|
|
<ModalHeader>{title || 'Uh Oh!'}</ModalHeader>
|
|
<ModalBody className="wide pad">
|
|
{message}
|
|
</ModalBody>
|
|
<ModalFooter>
|
|
<div>
|
|
{addCancel ? <button className="btn" onClick={this.hide}>Cancel</button> : null}
|
|
<button className="btn" onClick={this._handleOk}>
|
|
Ok
|
|
</button>
|
|
</div>
|
|
</ModalFooter>
|
|
</Modal>
|
|
);
|
|
}
|
|
}
|
|
|
|
AlertModal.propTypes = {};
|
|
|
|
export default AlertModal;
|