mirror of
https://github.com/Kong/insomnia
synced 2024-11-12 17:26:32 +00:00
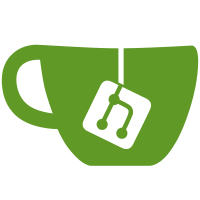
* feat: add prompt and update import logic * feat: update usages and plugin api * chore: WIP * chore: convert to options * chore: don't create workspace model until needed * chore: add OR condition * chore: add workspace name and don't change scope * feat: prompt with appropriate name * chore: rename * chore: rename type * chore: update signature * chore: properly type the import functions * feat: don't activate the workspace after importing * chore: show loading on homepage * fix: typo * chore: fix tests and rename * Update packages/insomnia-app/app/common/__tests__/import.test.js Co-authored-by: David Marby <david@dmarby.se> Co-authored-by: David Marby <david@dmarby.se>
54 lines
1.7 KiB
JavaScript
54 lines
1.7 KiB
JavaScript
// @flow
|
|
import {
|
|
exportWorkspacesHAR,
|
|
exportWorkspacesData,
|
|
importRaw,
|
|
importUri,
|
|
} from '../../common/import';
|
|
import type { Workspace, WorkspaceScope } from '../../models/workspace';
|
|
import type { ImportRawConfig } from '../../common/import';
|
|
|
|
type PluginImportOptions = { workspaceId?: string, scope?: WorkspaceScope };
|
|
|
|
export function init(): { data: { import: Object, export: Object } } {
|
|
return {
|
|
data: {
|
|
import: {
|
|
async uri(uri: string, options: PluginImportOptions = {}): Promise<void> {
|
|
await importUri(uri, buildImportRawConfig(options));
|
|
},
|
|
async raw(text: string, options: PluginImportOptions = {}): Promise<void> {
|
|
await importRaw(text, buildImportRawConfig(options));
|
|
},
|
|
},
|
|
export: {
|
|
async insomnia(
|
|
options: {
|
|
includePrivate?: boolean,
|
|
format?: 'json' | 'yaml',
|
|
workspace?: Workspace,
|
|
} = {},
|
|
): Promise<string> {
|
|
options = options || {};
|
|
return exportWorkspacesData(
|
|
options.workspace || null,
|
|
!!options.includePrivate,
|
|
options.format || 'json',
|
|
);
|
|
},
|
|
async har(
|
|
options: { includePrivate?: boolean, workspace?: Workspace } = {},
|
|
): Promise<string> {
|
|
return exportWorkspacesHAR(options.workspace || null, !!options.includePrivate);
|
|
},
|
|
},
|
|
},
|
|
};
|
|
}
|
|
|
|
function buildImportRawConfig(options: PluginImportOptions): ImportRawConfig {
|
|
const getWorkspaceId = () => Promise.resolve(options.workspaceId || null);
|
|
const getWorkspaceScope = options.scope && (() => Promise.resolve(options.scope));
|
|
return { getWorkspaceId, getWorkspaceScope };
|
|
}
|