mirror of
https://github.com/Kong/insomnia
synced 2024-11-08 23:00:30 +00:00
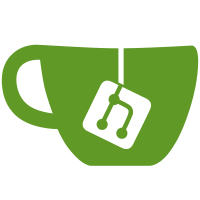
* use selector and improve types * Loading remote projects now requires a team id; it is no longer optional * activate with workspace or workspace id * extract helper * normalize graphql return type for a remote project to include the team * pull into the space as defined by the project team id, not by the active space. If the space doesn't exist, create it * use helper * make testing easier by putting the active space name as the first breadcrumb * fix tests * add the active space into the title * refactor and add tests * remove todo
34 lines
1.0 KiB
TypeScript
34 lines
1.0 KiB
TypeScript
|
|
import { useCallback, useEffect } from 'react';
|
|
import { useSelector } from 'react-redux';
|
|
|
|
import { database } from '../../common/database';
|
|
import { initializeSpaceFromTeam } from '../../sync/vcs/initialize-model-from';
|
|
import { VCS } from '../../sync/vcs/vcs';
|
|
import { selectIsLoggedIn } from '../redux/selectors';
|
|
import { useSafeState } from './use-safe-state';
|
|
|
|
export const useRemoteSpaces = (vcs?: VCS) => {
|
|
const [loading, setLoading] = useSafeState(false);
|
|
const isLoggedIn = useSelector(selectIsLoggedIn);
|
|
|
|
const refresh = useCallback(async () => {
|
|
if (vcs && isLoggedIn) {
|
|
setLoading(true);
|
|
|
|
const teams = await vcs.teams();
|
|
const spaces = await Promise.all(teams.map(initializeSpaceFromTeam));
|
|
await database.batchModifyDocs({ upsert: spaces });
|
|
|
|
setLoading(false);
|
|
}
|
|
}, [vcs, setLoading, isLoggedIn]);
|
|
|
|
// If the refresh callback changes, refresh
|
|
useEffect(() => {
|
|
(async () => { await refresh(); })();
|
|
}, [refresh]);
|
|
|
|
return { loading, refresh };
|
|
};
|