mirror of
https://github.com/Kong/insomnia
synced 2024-11-08 06:39:48 +00:00
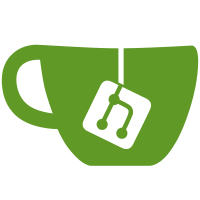
* add /cookies endpoint to smoke test mock server * add smoke test steps for /cookies endpoint * Update packages/insomnia-smoke-test/tests/app.test.ts Co-authored-by: James Gatz <jamesgatzos@gmail.com>
61 lines
1.4 KiB
TypeScript
61 lines
1.4 KiB
TypeScript
import express from 'express';
|
|
import basicAuth from 'express-basic-auth';
|
|
|
|
import { basicAuthCreds } from '../fixtures/constants';
|
|
|
|
const app = express();
|
|
const basicAuthRouter = express.Router();
|
|
const port = 4010;
|
|
|
|
// Artificially slow each request down
|
|
app.use((_req, _res, next) => {
|
|
setTimeout(next, 500);
|
|
});
|
|
|
|
app.get('/pets/:id', (req, res) => {
|
|
res.status(200).send({ id: req.params.id });
|
|
});
|
|
|
|
app.get('/sleep', (_req, res) => {
|
|
res.status(200).send({ sleep: true });
|
|
});
|
|
|
|
app.get('/cookies', (_req, res) => {
|
|
res
|
|
.status(200)
|
|
.header('content-type', 'text/plain')
|
|
.cookie('insomnia-test-cookie', 'value123')
|
|
.send(`${_req.headers['cookie']}`);
|
|
});
|
|
|
|
app.use('/file', express.static('fixtures/files'));
|
|
|
|
const { utf8, latin1 } = basicAuthCreds;
|
|
|
|
const users = {
|
|
[utf8.encoded.user]: utf8.encoded.pass,
|
|
[latin1.encoded.user]: latin1.encoded.pass,
|
|
};
|
|
|
|
basicAuthRouter.use(basicAuth({ users }));
|
|
|
|
basicAuthRouter.get('/', (_, res) => {
|
|
res
|
|
.status(200)
|
|
.header('content-type', 'text/plain')
|
|
.send('basic auth received');
|
|
});
|
|
|
|
app.use('/auth/basic', basicAuthRouter);
|
|
|
|
app.listen(port, () => {
|
|
console.log(`Listening at http://localhost:${port}`);
|
|
});
|
|
|
|
app.get('/delay/seconds/:duration', (req, res) => {
|
|
const delaySec = Number.parseInt(req.params.duration || '2');
|
|
setTimeout(function() {
|
|
res.send(`Delayed by ${delaySec} seconds`);
|
|
}, delaySec * 1000);
|
|
});
|