mirror of
https://github.com/Kong/insomnia
synced 2024-11-08 06:39:48 +00:00
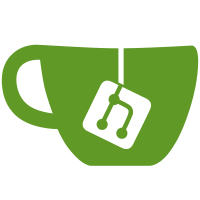
* chore: refactor * chore: fix one test * chore: fix all tests * chore: fix all existing tests * chore: add prompting tests * chore: ignore flaky test for now
37 lines
1.0 KiB
JavaScript
37 lines
1.0 KiB
JavaScript
import findAsync from './find-async';
|
|
|
|
export const waitUntilOpened = async (app, { modalName, title }) => {
|
|
if (modalName) {
|
|
const modal = await app.client.react$(modalName);
|
|
await modal.waitForDisplayed();
|
|
} else {
|
|
await app.client.waitUntilTextExists('div.modal__header__children', title);
|
|
}
|
|
};
|
|
|
|
export const close = async (app, modalName) => {
|
|
let modal;
|
|
if (modalName) {
|
|
modal = await app.client.react$(modalName);
|
|
} else {
|
|
const modals = await app.client.react$$('Modal');
|
|
modal = await findAsync(modals, m => m.isDisplayed());
|
|
}
|
|
|
|
await modal.$('button.modal__close-btn').then(e => e.click());
|
|
};
|
|
|
|
export const clickModalFooterByText = async (app, text) => {
|
|
const btn = await app.client
|
|
.$('.modal[aria-hidden=false] .modal__footer')
|
|
.then(e => e.$(`button*=${text}`));
|
|
await btn.waitForClickable();
|
|
await btn.click();
|
|
};
|
|
|
|
export const typeIntoModalInput = async (app, text) => {
|
|
const input = await app.client.$('.modal input');
|
|
await input.waitUntil(() => input.isFocused());
|
|
await input.keys(text);
|
|
};
|